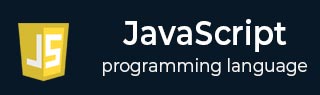
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - 处理程序
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象的 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxy
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期的方法
- JavaScript - 设置日期的方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - Unicode
什么是 Unicode?
Unicode 是一个通用的字符集,包含大多数语言、书写系统等的字符列表。它为每个字符提供一个唯一的编号,而不依赖于编程语言、平台、操作系统等。此外,它还包括标点符号、表情符号、特殊字符等。
简而言之,Unicode 集合包含唯一的数字,每个数字代表一个唯一的字符,其含义与平台、操作系统等无关。
Unicode 背后的直觉
在理解 Unicode 之前,让我们先了解其背后的理念。你能回答为什么你能阅读本教程吗?这是因为你知道所写字母的含义。读者(你)和作者对英语字母都有相同的理解;这就是你能够阅读作者所写内容的原因。
同样,计算机不理解字母。对于计算机而言,字母是位的序列,每个序列都映射到一个唯一的字符,称为 Unicode。
现在,让我们深入了解 Unicode。
JavaScript 中的 Unicode
JavaScript 允许开发人员在字符串文字和源代码中使用 Unicode 字符。开发人员需要使用转义表示法 (\u) 来在 JavaScript 代码中使用 Unicode 字符。
语法
用户可以按照以下语法在 JavaScript 中使用 Unicode 字符。
const char = '\uxxxx';
在上述语法中,'\uxxxx' 是一个 Unicode 字符。“xxxx”代表十六进制字符,“\u”代表转义表示法。
示例
示例:Unicode 转义序列
在下面的示例中,我们使用了 Unicode 转义序列来打印“hello”消息。
<html> <body> <div>Using unicode escape sequence</div> <div id = "output"> </div> <script> let str = '\u0068\u0065\u006c\u006c\u006f' document.getElementById("output").innerHTML = str; </script> </body> </html>
输出
Using unicode escape sequence hello
示例:在变量名中使用 Unicode 字符
在下面的代码中,我们使用了两个不同的 Unicode 字符作为两个不同的标识符(变量名)。在输出中,您可以观察到这两个标识符的值。
<html> <body> <div>Using unicode characters in variable names</div> <div id = "output"> </div> <script> // Using the Unicode characters in variable names let \u0061 = "Hello"; let \u0062 = "World"; document.getElementById("output").innerHTML = a + " " + b; </script> </body> </html>
输出
Using unicode characters in variable names Hello World
示例:在字符串中使用 Unicode 字符
在这个示例中,我们在字符串文字中使用了 Unicode 字符。输出显示字符串中间的特殊字符。
<html> <body> <div> Using the Unicode Characters in String </div> <div id = "output"> </div> <script> // Using the Unicode characters in the string let str = 'Hello \u00D8 \u00F8 World'; document.getElementById("output").innerHTML = str; </script> </body> </html>
输出
Using the Unicode Characters in String Hello Ø ø World
示例:为非 BMP(基本多语言平面)字符使用 Unicode
在下面的示例中,我们使用了 Unicode 字符(代码点)来显示非 BMP(基本多语言平面)字符。我们为一名医护人员进行了演示。
<html> <body> <div>showing person heath worker using unicode code point</div> <div id = "output"> </div> <script> // Showing emojis using the unicode characters const smileyFace = '\u{1F9D1}\u200D\u2695\uFE0F'; document.getElementById("output").innerHTML = smileyFace; </script> </body> </html>
输出
showing person heath worker using unicode code point 🧑⚕️
示例:使用 Unicode 字符显示表情符号
在下面的代码中,我们使用了Unicode字符来显示笑脸表情符号。
<html> <body> <div>Showing Emojies Using the Unicode Characters </div> <div id = "output"> </div> <script> // Showing emojis using the unicode characters const smileyFace = '\uD83D\uDE0A'; document.getElementById("output").innerHTML = smileyFace; </script> </body> </html>
输出
Showing Emojies Using the Unicode Characters 😊
正如我们所看到的,每个Unicode字符都代表一个唯一的字符。在JavaScript中,我们可以使用带标识符、字符串字面量等的Unicode字符。