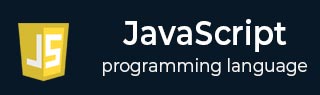
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 修改 HTML
- JavaScript - 修改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript 空值合并运算符
空值合并运算符
JavaScript 中的空值合并运算符用两个问号 (??) 表示。它接受两个操作数,如果第一个操作数不是null 或undefined,则返回第一个操作数。否则,它返回第二个操作数。它是一个在 ES2020 中引入的逻辑运算符。
在许多情况下,变量中可能存储空值或空值,这可能会改变代码的行为或产生错误。因此,当变量包含虚假值时,可以使用空值合并运算符使用默认值。
语法
可以使用以下语法使用空值合并运算符。
op1 ?? op2
空值合并运算符 (??) 如果第一个操作数 (op1) 为null 或undefined,则返回第二个操作数 (op2)。否则,'res' 变量将包含 'op2'。
上述语法类似于以下代码。
let res; if (op1 != null || op1 != undefined) { res = op1; } else { res = op2; }
示例
让我们借助一些示例详细了解空值合并运算符。
示例:处理 null 或 undefined
在下面的示例中,x 的值为null。我们使用 x 作为第一个操作数,5 作为第二个操作数。您可以在输出中看到 y 的值为 5,因为 x 为null。您可以为变量赋值 undefined。
<html> <body> <div id = "output"></div> <script> let x = null; let y = x ?? 5; document.getElementById("output").innerHTML = "The value of y is: " + y; </script> </body> </html>
它将产生以下结果:
The value of y is: 5
示例:处理数组中的 null 或 undefined
在下面的示例中,我们定义了一个包含数字的数组。我们使用空数组 ([]) 作为第二个操作数。因此,如果 arr 为 null 或 undefined,我们将空数组赋值给 arr1 变量。
<html> <body> <div id = "output"></div> <script> const arr = [65, 2, 56, 2, 3, 12]; const arr1 = arr ?? []; document.getElementById("output").innerHTML = "The value of arr1 is: " + arr1; </script> </body> </html>
它将产生以下结果:
The value of arr1 is: 65,2,56,2,3,12
示例:访问对象属性
在下面的示例中,我们创建了包含移动相关属性的对象。之后,我们访问对象的属性并使用值初始化变量。对象不包含 'brand' 属性,因此代码使用 'Apple' 初始化 'brand' 变量,您可以在输出中看到。
这样,在访问具有不同属性的对象的属性时,可以使用空值合并运算符。
<html> <body> <div id = "output"></div> <script> const obj = { product: "Mobile", price: 20000, color: "Blue", } let product = obj.product ?? "Watch"; let brand = obj.brand ?? "Apple"; document.getElementById("output").innerHTML = "The product is " + product + " of the brand " + brand; </script> </body> </html>
它将产生以下结果:
The product is Mobile of the brand Apple
短路
与逻辑 AND 和 OR 运算符一样,如果左操作数既不是 null 也不是 undefined,则空值合并运算符不会评估右操作数。
将 ?? 与 && 或 || 一起使用
当我们将 ?? 运算符与逻辑 AND 或 OR 运算符一起使用时,应使用括号明确指定优先级。
let x = 5 || 7 ?? 9; // Syntax Error let x = (5 || 7) ?? 9; // works
示例
在下面的示例中,我们使用了与 OR 运算符 (||) 和 AND 运算符 (&&) 的空值合并运算符。
<html> <body> <div id = "output"></div> <script> let x = (5 || 7) ?? 9; let y = (5 && 7) ?? 9; document.getElementById("output").innerHTML = "The value of x is : " + x + "<br>" + "The value of y is : " + y; </script> </body> </html>
上述程序将产生以下结果:
The value of x is : 5 The value of y is : 7