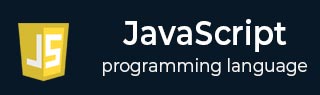
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 放置位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 按位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - 删除运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 带标签的模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookies
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - Array toSpliced() 方法
在 JavaScript 中,Array.toSpliced() 方法用于通过删除或替换现有元素和/或添加新元素来修改数组。此方法类似于 JavaScript Array.splice() 方法。
toSpliced() 方法修改多个数组元素:它从数组中删除给定数量的元素,从给定索引开始,然后在同一索引处插入给定元素。此方法不会修改/覆盖原始数组;而是返回一个新数组。
语法
以下是 JavaScript Array.toSpliced() 方法的语法:
toSpliced(start, deleteCount, item1, item2, ..., itemN)
参数
此方法采用以下参数:
- start − 开始更改数组的索引。
- deleteCount (可选) − 要删除的元素数量。如果省略或为 0,则不删除任何元素。
- item1, item2, ...,itemN (可选) − 要添加到数组中,从 start 索引开始的元素。
返回值
此方法返回一个包含已修改元素的新数组。
示例
示例 1
在以下示例中,我们使用 JavaScript Array toSpliced() 方法从索引位置 2 开始删除所有数组元素。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2); document.write(result); </script> </body> </html>
执行程序后,将删除从索引位置 2 开始的所有元素。
输出
apple,banana
示例 2
如果 toSpliced() 方法的 'deleteCount' 参数设置为 0,则不会从 animals 数组中删除任何元素。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0); document.write(result); </script> </body> </html>
输出
如我们所见,没有从数组中删除任何元素。
apple,banana,cherry,dates
示例 3
以下程序在索引位置 2 之前删除 0(零)个元素,并插入新元素“Pineapple”。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0, "Pineapple"); document.write(result); </script> </body> </html>
输出
如我们所见,元素“Pineapple”在索引位置 2 处插入,而无需删除任何现有元素。
apple,banana,Pineapple,cherry,dates
示例 4
以下程序在索引位置 2 之前删除 0(零)个元素,并插入两个新元素,即“Pineapple”和“Grapes”。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 0, "Pineapple", "Grapes"); document.write(result); </script> </body> </html>
元素“Pineapple”和“Grapes”在索引位置 2 处插入,而无需删除任何现有元素。
输出
apple,banana,Pineapple,Grapes,cherry,dates
示例 5
在以下示例中,我们从索引位置 2 开始删除 2 个元素。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 2); document.write(result); </script> </body> </html>
执行程序后,它将从数组中删除“cherry”和“dates”。
输出
apple,banana
示例 6
这里,我们删除索引位置 2 处的 1 个元素,并插入一个新元素“Grapes”。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 1, "Grapes"); document.write(result); </script> </body> </html>
执行程序后,将删除元素“cherry”,并在该索引处插入“Grapes”。
输出
apple,banana,Grapes,dates
示例 7
在此示例中,我们删除索引位置 2 处的 1 个元素,并插入两个新元素“Grapes”和“Pineapple”。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(2, 1, "Grapes", "Pineapple"); document.write(result); </script> </body> </html>
执行程序后,将删除元素“cherry”,并插入元素“Grapes”和“Pineapple”。
输出
apple,banana,Grapes,Pineapple,dates
示例 8
这里,我们从索引 -2(从数组末尾开始计数)删除 1 个元素。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(-2, 1); document.write(result); </script> </body> </html>
执行程序后,将删除元素“cherry”。
输出
apple,banana,dates
示例 9
在这里,我们从索引 -2(从数组末尾开始计数)删除 1 个元素,并插入两个新元素“Pineapple”和“Grapes”。
<html> <body> <script> let fruits = ['apple', 'banana', 'cherry', 'dates']; let result = fruits.toSpliced(-2, 1, "Pineapple", "Grapes"); document.write(result); </script> </body> </html>
执行程序后,元素“cherry”将被删除,并且“Pineapple”和“Grapes”也将被删除。
输出
apple,banana,Pineapple,Grapes,dates