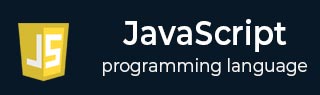
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - 删除运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - 处理程序
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 带标签的模板
- 面向对象的 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promises
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promisification
- JavaScript - Promises 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookies
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - Clickjacking 攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript DataView getBigUint64() 方法
JavaScript DataView 的getBigUint64()方法用于从该 DataView 的指定字节偏移量开始检索 8 字节的数据段。随后,它将它们解码为64 位无符号整数。您可以从 DataView 范围内的任何偏移量检索多个字节。
如果您尝试读取超出 DataView 有效范围的位置的数据,此方法将抛出'RangeError'异常。
语法
以下是 JavaScript DataView getBigUint64()方法的语法:
getBigUnit64(byteOffset, littleEndian)
参数
此方法接受两个名为“byteOffset”和“littleEndian”的参数,如下所述:
- byteOffset - 从 DataView 中读取数据的起始位置。
- littleEndian - 指示数据是以小端序还是大端序格式存储。
返回值
此方法返回一个 BigInt,其范围从0到264 - 1(包括)。
示例 1
以下程序演示了 JavaScript DataView getBigUint64()方法的使用。
<html> <body> <script> const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 0; //find the highest possible BigInt value that fits for the big unsigned integer const value = 2n ** (64n - 1n) - 1n; document.write("The byte offset: ", byteOffset); document.write("<br>Value: ", value); //using the setBigUnit64() method to store value data_view.setBigUint64(byteOffset, value); //using the getBigUnit64() method document.write("<br>The stored value: ", data_view.getBigUint64(byteOffset)); </script> </body> </html>
输出
上述程序返回存储的值。
The byte offset: 0 Value: 9223372036854775807 The stored value: 9223372036854775807
示例 2
以下是setBigUint64()方法的另一个示例。我们使用此方法从指定的字节偏移量1检索数据视图的 8 字节数据段。
<html> <body> <script> const { buffer } = new Uint8Array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]); const data_view = new DataView(buffer); const byteOffset = 1; document.write("The byte offset: ", byteOffset); document.write("<br>The data_view.getBigUnit64(1) method returns: ", data_view.getBigUint64(byteOffset)); </script> </body> </html>
输出
执行上述程序后,它将返回一个 8 字节的数据段,如下所示:
The byte offset: 1 The data_view.getBigUnit64(1) method returns: 72623859790382856
示例 3
如果 byteOffset 参数的值超出此数据视图的范围,则会抛出'RangeError'异常。
<html> <body> <script> const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const byteOffset = 1; //find the highest possible BigInt value const value = 2n ** (64n - 1n) - 1n; document.write("The byte offset: ", byteOffset); document.write("<br>Value: ", value); //storing the value data_view.setBigInt64(byteOffset, value); try { //using the getBigUnit64() method document.write("<br>The store value: ", data_view.getBigUnit64(-1)); } catch (error) { document.write("<br>", error); } </script> </body> </html>
输出
执行上述程序后,将抛出以下异常:
The byte offset: 1 Value: 9223372036854775807 TypeError: data_view.getBigUnit64 is not a function
广告