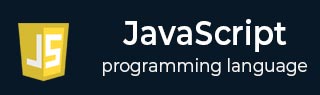
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - if...else
- JavaScript - while 循环
- JavaScript - for 循环
- JavaScript - for...in
- Javascript - for...of
- JavaScript - 循环控制
- JavaScript - break 语句
- JavaScript - continue 语句
- JavaScript - switch case
- JavaScript - 用户自定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - String
- JavaScript - Array
- JavaScript - Date
- JavaScript - DataView
- JavaScript - 处理程序
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Set
- JavaScript - WeakSet
- JavaScript - Map
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标签模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixin
- JavaScript - Proxy
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promisification
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 其他
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图片地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript DataView setInt16() 方法
JavaScript DataView 的setInt16() 方法将数值存储为16 位有符号整数,存储在从 DataView 指定字节偏移量开始的 2 个字节中。多个字节可以存储在边界内的任何偏移量。
如果数值不在-32768 到 32767的范围内,则不会将该值存储到此 DataView 中;如果 byteOffset 参数值超出此 DataView 的范围,则会抛出'RangeError'异常。
语法
以下是 JavaScript DataView setInt16() 方法的语法:
setInt16(byteOffset, value, littleEndian)
参数
此方法接受三个参数,分别为“byteOffset”、“value”和“littleEndian”,如下所述:
- byteOffset - DataView 中将存储字节的位置。
- value - 需要存储的 16 位有符号整数。
- littleEndian - 指示数据值是以小端还是大端格式存储。
返回值
此方法返回'undefined',因为它只存储字节值。
示例 1
以下程序演示了 JavaScript DataView setInt16() 方法的用法。
<html> <body> <script> const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const value = 255; const byteOffset = 1; document.write("Value: ", value); document.write("<br>The byte offset: ", byteOffset); //using the setInt16() method document.write("<br>The data_view.setInt16() method returns: ", data_view.setInt16(byteOffset, value)); </script> </body> </html>
输出
上述程序返回 'undefined':
Value: 255 The byte offset: 1 The data_view.setInt16() method returns: undefined
示例 2
如果数据值超出-32768到32767的范围,则setInt16()方法将不会存储指定的值,因为它超过了 8 位有符号整数的范围。
<html> <body> <script> const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const value = 327678; const byteOffset = 0; document.write("Value: ", value); document.write("<br>The byte offset: ", byteOffset); //using the setInt16() method data_view.setInt16(byteOffset, value); document.write("<br>The store value: ", data_view.getInt32(1)); </script> </body> </html>
输出
程序执行后,由于数据值超出可接受值的范围,因此不会存储数据值:
Value: 327678 The byte offset: 0 The store value: -33554432
示例 3
如果 byteOffset 参数的值超出此数据视图的范围,则会抛出'RangeError'异常。
<html> <body> <script> const buffer = new ArrayBuffer(16); const data_view = new DataView(buffer); const value = 300; const byteOffset = -2; document.write("Value: ", value); document.write("<br>The byte offset: ", byteOffset); try { //using the setInt16() method data_view.setInt16(byteOffset, value); } catch (error) { document.write("<br>", error); } </script> </body> </html>
输出
执行上述程序后,将抛出 'RangeError' 异常,如下所示:
Value: 300 The byte offset: -2 RangeError: Offset is outside the bounds of the DataView
广告