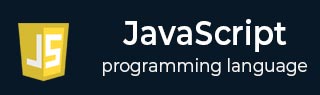
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 放置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - delete 运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 指数运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 带标签的模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookie
- JavaScript - Cookie
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookie
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - 窗口/文档事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM 文档
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - Clickjacking 攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript 字符串 endsWith() 方法
JavaScript 字符串 endsWith() 方法用于确定字符串是否以指定的字符(子字符串)结尾。如果在字符串末尾找到了指定的子字符串字符,则返回布尔值“true”;否则,返回“false”。
如果 searchString 参数是正则表达式,则此方法会抛出 TypeError 异常。它是区分大小写的,因此它将“Hello”和“hello”视为不同的字符串。
语法
以下是 JavaScript 字符串 endsWith() 方法的语法:
endsWith(searchString, endPosition)
参数
此方法接受两个参数:“searchString” 和“endPosition”。endPosition 参数是可选的,并指定字符串中搜索结束的索引(位置)。
- searchString − 要在字符串末尾搜索的字符。
- endPosition(可选) − 字符串中搜索结束的位置。
返回值
如果字符串以指定的子字符串结尾,则此方法返回 true,否则返回 false。
示例 1
如果字符串以指定的字符结尾,则返回 true。
在此示例中,我们使用 JavaScript 字符串 endsWith() 方法来检查字符串“TutorialsPoint”是否以指定的子字符串“Point”结尾。
<html> <head> <title>JavaScript String endsWith() Method</title> </head> <body> <script> const str = "TutorialsPoint"; const searchString = "Point"; document.write("Original string: ", str); document.write("<br>Search string: ", searchString); document.write("<br>Is string '", str, "' ends with '", searchString, "'or not? ", str.endsWith(searchString)); </script> </body> </html>
输出
以上程序返回“true”。
Original string: TutorialsPoint Search string: Point Is string 'TutorialsPoint' ends with 'Point'or not? true
示例 2
如果字符串没有以指定的字符结尾,则返回 false。
以下是 JavaScript 字符串 endsWith() 方法的另一个示例。在此示例中,我们使用此方法来确定字符串“Hello World”是否以指定的字符串“Word”结尾。
<html> <head> <title>JavaScript String endsWith() Method</title> </head> <body> <script> const str = "Hello World"; const searchString = "Word"; document.write("Original string: ", str); document.write("<br>Search string: ", searchString); document.write("<br>Is string '", str, "' ends with '", searchString, "' or not? ", str.endsWith(searchString)); </script> </body> </html>
输出
执行以上程序后,它将返回“false”。
Original string: Hello World Search string: Word Is string 'Hello World' ends with 'Word' or not? false
示例 3
如果我们将 endPosition 参数值传递为 15,它将检查索引 15 处的字符串结尾是否以指定的子字符串结尾,并且其余字符串将被忽略。如果子字符串与 searchString(直到索引 15)的结尾匹配,则返回 true;否则,返回 false。
<html> <head> <title>JavaScript String endsWith() Method</title> </head> <body> <script> const str = "Welcome to Tutorials Point"; let endPosition = 15; const searchString = "Tuto"; document.write("Original string: ", str); document.write("<br>End position: ", endPosition); document.write("<br>Search string: ", searchString); document.write("<br>String actual length: ", str.length); document.write("<br>Is string '", str, "'(upto length 15) ends with '",searchString,"' or not? ", str.endsWith(searchString, endPosition)); </script> </body> </html>
输出
执行以上程序后,它将返回“true”。
Original string: Welcome to Tutorials Point End position: 15 Search string: Tuto String actual length: 26 Is string 'Welcome to Tutorials Point'(upto length 15) ends with 'Tuto' or not? true
示例 4
JavaScript 字符串 endsWith() 方法期望 searchString 参数为字符串。如果它是正则表达式,则会抛出“TypeError”异常。
<html> <head> <title>JavaScript String endsWith() Method</title> </head> <body> <script> const str = "Tutorials Point"; const searchString = /ab+c/; document.write("Original string: ", str); document.write("<br>Search string: ", searchString); try { document.write("Is string '",str,"' is ends with '",searchString,"' or not? ", str.endsWith(searchString)); } catch (error) { document.write("<br>", error); } </script> </body> </html>
输出
以上程序抛出“TypeError”异常。
Original string: Tutorials Point Search string: /ab+c/ TypeError: First argument to String.prototype.endsWith must not be a regular expression