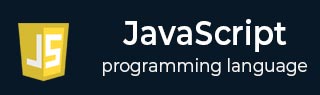
- Javascript 基础教程
- Javascript - 首页
- JavaScript - 路线图
- JavaScript - 概述
- JavaScript - 特性
- JavaScript - 启用
- JavaScript - 放置位置
- JavaScript - 语法
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - 注释
- JavaScript - 变量
- JavaScript - let 语句
- JavaScript - 常量
- JavaScript - 数据类型
- JavaScript - 类型转换
- JavaScript - 严格模式
- JavaScript - 保留关键字
- JavaScript 运算符
- JavaScript - 运算符
- JavaScript - 算术运算符
- JavaScript - 比较运算符
- JavaScript - 逻辑运算符
- JavaScript - 位运算符
- JavaScript - 赋值运算符
- JavaScript - 条件运算符
- JavaScript - typeof 运算符
- JavaScript - 空值合并运算符
- JavaScript - 删除运算符
- JavaScript - 逗号运算符
- JavaScript - 分组运算符
- JavaScript - Yield 运算符
- JavaScript - 展开运算符
- JavaScript - 幂运算符
- JavaScript - 运算符优先级
- JavaScript 控制流
- JavaScript - If...Else
- JavaScript - While 循环
- JavaScript - For 循环
- JavaScript - For...in
- Javascript - For...of
- JavaScript - 循环控制
- JavaScript - Break 语句
- JavaScript - Continue 语句
- JavaScript - Switch Case
- JavaScript - 用户定义迭代器
- JavaScript 函数
- JavaScript - 函数
- JavaScript - 函数表达式
- JavaScript - 函数参数
- JavaScript - 默认参数
- JavaScript - Function() 构造函数
- JavaScript - 函数提升
- JavaScript - 自执行函数
- JavaScript - 箭头函数
- JavaScript - 函数调用
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - 闭包
- JavaScript - 变量作用域
- JavaScript - 全局变量
- JavaScript - 智能函数参数
- JavaScript 对象
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - DataView
- JavaScript - Handler
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - 可迭代对象
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - 模板字面量
- JavaScript - 标记模板
- 面向对象 JavaScript
- JavaScript - 对象
- JavaScript - 类
- JavaScript - 对象属性
- JavaScript - 对象方法
- JavaScript - 静态方法
- JavaScript - 显示对象
- JavaScript - 对象访问器
- JavaScript - 对象构造函数
- JavaScript - 原生原型
- JavaScript - ES5 对象方法
- JavaScript - 封装
- JavaScript - 继承
- JavaScript - 抽象
- JavaScript - 多态
- JavaScript - 解构赋值
- JavaScript - 对象解构
- JavaScript - 数组解构
- JavaScript - 嵌套解构
- JavaScript - 可选链
- JavaScript - 全局对象
- JavaScript - Mixins
- JavaScript - 代理
- JavaScript 版本
- JavaScript - 历史
- JavaScript - 版本
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript 异步
- JavaScript - 异步
- JavaScript - 回调函数
- JavaScript - Promise
- JavaScript - Async/Await
- JavaScript - 微任务
- JavaScript - Promise 化
- JavaScript - Promise 链式调用
- JavaScript - 定时事件
- JavaScript - setTimeout()
- JavaScript - setInterval()
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie 属性
- JavaScript - 删除 Cookies
- JavaScript 浏览器 BOM
- JavaScript - 浏览器对象模型
- JavaScript - Window 对象
- JavaScript - Document 对象
- JavaScript - Screen 对象
- JavaScript - History 对象
- JavaScript - Navigator 对象
- JavaScript - Location 对象
- JavaScript - Console 对象
- JavaScript Web API
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript 事件
- JavaScript - 事件
- JavaScript - DOM 事件
- JavaScript - addEventListener()
- JavaScript - 鼠标事件
- JavaScript - 键盘事件
- JavaScript - 表单事件
- JavaScript - Window/Document 事件
- JavaScript - 事件委托
- JavaScript - 事件冒泡
- JavaScript - 事件捕获
- JavaScript - 自定义事件
- JavaScript 错误处理
- JavaScript - 错误处理
- JavaScript - try...catch
- JavaScript - 调试
- JavaScript - 自定义错误
- JavaScript - 扩展错误
- JavaScript 重要关键字
- JavaScript - this 关键字
- JavaScript - void 关键字
- JavaScript - new 关键字
- JavaScript - var 关键字
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM 方法和属性
- JavaScript - DOM Document
- JavaScript - DOM 元素
- JavaScript - DOM 属性 (Attr)
- JavaScript - DOM 表单
- JavaScript - 更改 HTML
- JavaScript - 更改 CSS
- JavaScript - DOM 动画
- JavaScript - DOM 导航
- JavaScript - DOM 集合
- JavaScript - DOM NodeList
- JavaScript - DOM DOMTokenList
- JavaScript 杂项
- JavaScript - Ajax
- JavaScript - 异步迭代
- JavaScript - Atomics 对象
- JavaScript - Rest 参数
- JavaScript - 页面重定向
- JavaScript - 对话框
- JavaScript - 页面打印
- JavaScript - 验证
- JavaScript - 动画
- JavaScript - 多媒体
- JavaScript - 图像地图
- JavaScript - 浏览器
- JavaScript - JSON
- JavaScript - 多行字符串
- JavaScript - 日期格式
- JavaScript - 获取日期方法
- JavaScript - 设置日期方法
- JavaScript - 模块
- JavaScript - 动态导入
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - 浅拷贝
- JavaScript - 调用栈
- JavaScript - 引用类型
- JavaScript - IndexedDB
- JavaScript - 点击劫持攻击
- JavaScript - 柯里化
- JavaScript - 图形
- JavaScript - Canvas
- JavaScript - 防抖
- JavaScript - 性能
- JavaScript - 样式指南
JavaScript - RegExp exec 方法
JavaScript 的 RegExp.exec() 方法用于在字符串中搜索正则表达式定义的指定模式。如果找到匹配项,则返回包含匹配文本的 数组,否则返回结果为 null。
在 JavaScript 中,正则表达式 (RegExp) 是一个对象,它描述用于定义搜索模式的字符序列。正则表达式的最佳用途是用于表单验证。当用户通过表单(例如输入字段)提交数据时,您可以应用正则表达式来确保数据满足特定条件。
语法
以下是 JavaScript RegExp.exec() 方法的语法:
RegExp.exec(str)
参数
此方法接受一个名为“str”的参数,如下所述:
str - 需要搜索的字符串。
返回值
如果找到匹配项,此方法返回一个包含匹配文本的数组。否则,它将返回 null 值。
示例
如果找到匹配项,此方法将返回一个包含匹配文本的数组。
示例 1
在下面的示例中,我们使用 JavaScript 的 RegExp.exec() 方法在字符串 "Tutorials point" 中搜索正则表达式定义的模式 ('to*', 'g')。
<html> <head> <title>JavaScript RegExp.exec() Method</title> </head> <body> <script> const regex = RegExp('to*', 'g'); const str = "Tutorials point"; document.write("The string to be searched: ", str); document.write("<br>The regex: ", regex); let arr = {}; arr = regex.exec(str); document.write("<br>An array with matched text: ", arr); document.write("<br>The array length: ", arr.length); </script> </body> </html>
输出
上述程序返回一个包含匹配文本的数组。
The string to be searched: Tutorials point The regex: /to*/g An array with matched text: to The array length: 1
示例 2
如果未找到匹配项,则该方法将返回 null。
以下是 JavaScript RegExp.exec() 方法的另一个示例。我们使用此方法在字符串 "Virat is a damn good batsman" 中搜索正则表达式定义的模式 ('/mn\*', 'g')。
<html> <head> <title>JavaScript RegExp.exec() Method</title> </head> <body> <script> const regex = RegExp('/mn\*', 'g'); const str = "Virat is a damn good batsman"; document.write("The string to be searched: ", str); document.write("<br>The regex: ", regex); let arr = {}; arr = regex.exec(str); document.write("<br>An array with matched text: ", arr); document.write("<br>The array length: ", arr.length); </script> </body> </html>
输出
执行上述程序后,它将返回 null。
The string to be searched: Virat is a damn good batsman The regex: /\/mn*/g An array with matched text: null
示例 3
我们可以在条件语句中使用此方法的结果来检查是否找到匹配项。
<html> <head> <title>JavaScript RegExp.exec() Method</title> </head> <body> <script> const regex = RegExp('\llo*', 'g'); const str = "Hello World"; document.write("The string to be searched: ", str); document.write("<br>The regex: ", regex); let result = {}; result = regex.exec(str); //using method result in conditional statement.... if(result != null){ document.write("<br>The string '" + result[0] + "' found.") } else { document.write("<br>Not found..."); } </script> </body> </html>
输出
执行上述程序后,它将根据条件返回一个语句:
The string to be searched: Hello World The regex: /llo*/g The string 'llo' found.
广告