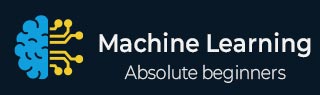
- 机器学习基础
- ML - 首页
- ML - 简介
- ML - 入门
- ML - 基本概念
- ML - 生态系统
- ML - Python 库
- ML - 应用
- ML - 生命周期
- ML - 必备技能
- ML - 实现
- ML - 挑战与常见问题
- ML - 限制
- ML - 真实案例
- ML - 数据结构
- ML - 数学基础
- ML - 人工智能
- ML - 神经网络
- ML - 深度学习
- ML - 获取数据集
- ML - 分类数据
- ML - 数据加载
- ML - 数据理解
- ML - 数据准备
- ML - 模型
- ML - 监督学习
- ML - 无监督学习
- ML - 半监督学习
- ML - 强化学习
- ML - 监督学习 vs. 无监督学习
- 机器学习数据可视化
- ML - 数据可视化
- ML - 直方图
- ML - 密度图
- ML - 箱线图
- ML - 相关矩阵图
- ML - 散点矩阵图
- 机器学习统计学
- ML - 统计学
- ML - 均值、中位数、众数
- ML - 标准差
- ML - 百分位数
- ML - 数据分布
- ML - 偏度和峰度
- ML - 偏差和方差
- ML - 假设
- 机器学习中的回归分析
- ML - 回归分析
- ML - 线性回归
- ML - 简单线性回归
- ML - 多元线性回归
- ML - 多项式回归
- 机器学习中的分类算法
- ML - 分类算法
- ML - 逻辑回归
- ML - K 近邻算法 (KNN)
- ML - 朴素贝叶斯算法
- ML - 决策树算法
- ML - 支持向量机
- ML - 随机森林
- ML - 混淆矩阵
- ML - 随机梯度下降
- 机器学习中的聚类算法
- ML - 聚类算法
- ML - 基于质心的聚类
- ML - K 均值聚类
- ML - K 中值聚类
- ML - 均值漂移聚类
- ML - 层次聚类
- ML - 基于密度的聚类
- ML - DBSCAN 聚类
- ML - OPTICS 聚类
- ML - HDBSCAN 聚类
- ML - BIRCH 聚类
- ML - 关联传播
- ML - 基于分布的聚类
- ML - 凝聚层次聚类
- 机器学习中的降维
- ML - 降维
- ML - 特征选择
- ML - 特征提取
- ML - 向后消除法
- ML - 向前特征构造
- ML - 高相关性过滤器
- ML - 低方差过滤器
- ML - 缺失值比例
- ML - 主成分分析
- 强化学习
- ML - 强化学习算法
- ML - 利用与探索
- ML - Q 学习
- ML - REINFORCE 算法
- ML - SARSA 强化学习
- ML - 演员-评论家方法
- 深度强化学习
- ML - 深度强化学习
- 量子机器学习
- ML - 量子机器学习
- ML - 使用 Python 的量子机器学习
- 机器学习杂项
- ML - 性能指标
- ML - 自动化工作流程
- ML - 提升模型性能
- ML - 梯度提升
- ML - 自举汇聚 (Bagging)
- ML - 交叉验证
- ML - AUC-ROC 曲线
- ML - 网格搜索
- ML - 数据缩放
- ML - 训练和测试
- ML - 关联规则
- ML - Apriori 算法
- ML - 高斯判别分析
- ML - 成本函数
- ML - 贝叶斯定理
- ML - 精度和召回率
- ML - 对抗样本
- ML - 堆叠
- ML - 轮次
- ML - 感知器
- ML - 正则化
- ML - 过拟合
- ML - P 值
- ML - 熵
- ML - MLOps
- ML - 数据泄露
- ML - 机器学习的商业化
- ML - 数据类型
- 机器学习 - 资源
- ML - 快速指南
- ML - 速查表
- ML - 面试题
- ML - 有用资源
- ML - 讨论
机器学习 - 对抗样本
对抗机器学习是机器学习的一个子领域,专注于研究机器学习模型对抗攻击的脆弱性。对抗攻击是故意尝试通过在输入数据中引入小的扰动来欺骗机器学习模型。这些扰动通常对人类来说是难以察觉的,但它们会导致模型以高置信度做出错误的预测。对抗攻击可能在自动驾驶、安全系统和医疗保健等现实世界应用中造成严重后果。
有几种类型的对抗攻击,包括:
逃避攻击 - 这些攻击旨在操纵输入数据以导致模型将其错误分类。逃避攻击可以是有针对性的,攻击者知道目标类别;也可以是非针对性的,攻击者只想导致错误分类。
投毒攻击 - 这些攻击旨在操纵训练数据,使模型偏向于特定类别或降低其整体准确性。投毒攻击可以是数据投毒(攻击者修改训练数据)或模型投毒(攻击者修改模型本身)。
模型反演攻击 - 这些攻击旨在通过观察模型的输出,推断有关训练数据或模型本身的敏感信息。
为了防御对抗攻击,研究人员提出了一些技术,包括:
对抗训练 - 此技术包括使用对抗样本增强训练数据,以使模型更能抵抗对抗攻击。
防御性蒸馏 - 此技术涉及训练第二个模型来学习第一个模型的输出,使其更能抵抗对抗攻击。
随机化 - 此技术包括向输入数据或模型参数添加随机噪声,以使攻击者更难以创建对抗样本。
检测和拒绝 - 此技术包括检测对抗样本并在它们被模型处理之前将其拒绝。
Python 实现
在 Python 中,一些库提供了对抗攻击和防御的实现,包括:
CleverHans - 此库为 TensorFlow、Keras 和 PyTorch 提供了对抗攻击和防御的集合。
ART(对抗鲁棒性工具箱) - 此库提供了一套全面的工具,用于评估和防御机器学习模型中的对抗攻击。
Foolbox - 此库为 PyTorch、TensorFlow 和 Keras 提供了对抗攻击的集合。
在下面的示例中,我们将使用对抗鲁棒性工具箱 (ART) 来实现对抗机器学习:
首先,我们需要使用 pip 安装 ART 包:
pip install adversarial-robustness-toolbox
然后,我们可以使用 ART 库在一个预训练的模型上创建一个对抗样本。
示例
import tensorflow as tf from keras.datasets import mnist from keras.models import Sequential from keras.layers import Dense, Flatten, Conv2D, MaxPooling2D from keras.optimizers import Adam from keras.utils import to_categorical from art.attacks.evasion import FastGradientMethod from art.estimators.classification import KerasClassifier import tensorflow as tf tf.compat.v1.disable_eager_execution() # Load the MNIST dataset (x_train, y_train), (x_test, y_test) = mnist.load_data() # Preprocess the data x_train = x_train.reshape(-1, 28, 28, 1).astype('float32') / 255 x_test = x_test.reshape(-1, 28, 28, 1).astype('float32') / 255 y_train = to_categorical(y_train, 10) y_test = to_categorical(y_test, 10) # Define the model architecture model = Sequential() model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1))) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Flatten()) model.add(Dense(10, activation='softmax')) # Compile the model model.compile(loss='categorical_crossentropy', optimizer=Adam(lr=0.001), metrics=['accuracy']) # Wrap the model with ART KerasClassifier classifier = KerasClassifier(model=model, clip_values=(0, 1), use_logits=False) # Train the model classifier.fit(x_train, y_train) # Evaluate the model on the test set accuracy = classifier.evaluate(x_test, y_test)[1] print("Accuracy on test set: %.2f%%" % (accuracy * 100)) # Generate adversarial examples using the FastGradientMethod attack attack = FastGradientMethod(estimator=classifier, eps=0.1) x_test_adv = attack.generate(x_test) # Evaluate the model on the adversarial examples accuracy_adv = classifier.evaluate(x_test_adv, y_test)[1] print("Accuracy on adversarial examples: %.2f%%" % (accuracy_adv * 100))
在此示例中,我们首先加载并预处理 MNIST 数据集。然后,我们定义一个简单的卷积神经网络 (CNN) 模型,并使用分类交叉熵损失和 Adam 优化器对其进行编译。
我们将模型与 ART KerasClassifier 包装起来,使其与 ART 攻击兼容。然后,我们在训练集上训练模型 10 个 epoch,并在测试集上对其进行评估。
接下来,我们使用最大扰动为 0.1 的 FastGradientMethod 攻击生成对抗样本。最后,我们评估模型在对抗样本上的性能。
输出
执行此代码时,将生成以下输出:
Train on 60000 samples Epoch 1/20 60000/60000 [==============================] - 17s 277us/sample - loss: 0.3530 - accuracy: 0.9030 Epoch 2/20 60000/60000 [==============================] - 15s 251us/sample - loss: 0.1296 - accuracy: 0.9636 Epoch 3/20 60000/60000 [==============================] - 18s 300us/sample - loss: 0.0912 - accuracy: 0.9747 Epoch 4/20 60000/60000 [==============================] - 18s 295us/sample - loss: 0.0738 - accuracy: 0.9791 Epoch 5/20 60000/60000 [==============================] - 18s 300us/sample - loss: 0.0654 - accuracy: 0.9809 -------continue