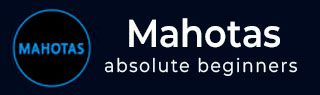
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 处理图像
- Mahotas - 处理图像
- Mahotas - 加载图像
- Mahotas - 加载图像为灰度
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像的质心
- Mahotas - 图像卷积
- Mahotas - 创建 RGB 图像
- Mahotas - 图像的欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像中的局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸 RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB 到灰度转换
- Mahotas - RGB 到 LAB 转换
- Mahotas - RGB 到棕褐色转换
- Mahotas - RGB 到 XYZ 转换
- Mahotas - XYZ 到 LAB 转换
- Mahotas - XYZ 到 RGB 转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 标记图像
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学操作
- Mahotas - 形态学算子
- Mahotas - 查找图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像的偏心率
- Mahotas - 叠加图像
- Mahotas - 图像的圆度
- Mahotas - 调整图像大小
- Mahotas - 图像直方图
- Mahotas - 膨胀图像
- Mahotas - 腐蚀图像
- Mahotas - 分水岭
- Mahotas - 图像上的开运算
- Mahotas - 图像上的闭运算
- Mahotas - 填充图像中的孔洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像的条件分水岭
- Mahotas - 图像中的局部最小值
- Mahotas - 图像的区域最大值
- Mahotas - 图像的区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen 局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形实用程序
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralic 特征
- 标记区域的权重
- Mahotas - Zernike 特征
- Mahotas - Zernike 矩
- Mahotas - 排序滤波器
- Mahotas - 2D 拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu 方法
- Mahotas - 高斯滤波
- Mahotas - 击中与不击中变换
- Mahotas - 标记最大数组
- Mahotas - 图像的平均值
- Mahotas - SURF 密集点
- Mahotas - SURF 积分
- Mahotas - Haar 变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签的边界
- 反转 Haar 变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 移除带边框的标记
- Mahotas - Daubechies 小波
- Mahotas - Sobel 边缘检测
Mahotas - 条件腐蚀图像
在我们上一章中,我们探讨了图像腐蚀的概念,这是一种用于将所有像素缩小到图像中区域边界的操作。另一方面,条件腐蚀基于某些条件,缩小(移除)某些特定区域的像素。
条件可以基于图像像素的强度值或图像中的一些特定模式。
例如,让我们考虑一个灰度图像。您可以有条件地仅腐蚀满足特定强度阈值的像素,而不是腐蚀所有前景像素。
如果像素的强度低于预定义的阈值,则应用腐蚀;否则,像素保持不变。
在 Mahotas 中条件腐蚀图像
在 mahotas 中,条件腐蚀是传统腐蚀操作的扩展,它包含基于第二张图像(通常称为“标记图像”)的条件。
它允许您控制腐蚀过程,以便腐蚀仅在标记图像具有特定像素值的位置发生。
我们可以使用 **cerode()** 函数在 mahotas 中对图像执行条件腐蚀。此函数根据标记图像的像素值将腐蚀过程限制在特定区域。
mahotas.cerode() 函数
Mahotas 中的 cerode() 函数接受两个输入 - 输入图像和掩码(条件)数组。它根据提供的条件对输入图像执行条件腐蚀,并返回生成的腐蚀图像。
掩码用于识别图像内的特定区域。它们充当过滤器,突出显示某些区域,同时忽略其他区域。
语法
以下是 mahotas 中 cerode() 函数的基本语法:
mahotas.cerode(f, g, Bc={3x3 cross}, out={np.empty_as(A)})
其中,
**f** - 它是要执行条件腐蚀的输入图像。
**g** - 它是条件腐蚀期间要应用的掩码。
**Bc={3×3 十字} (可选)** - 它是用于膨胀的结构元素。默认为 {3×3 十字}。
**out={np.empty_as(A)} (可选)** - 它提供一个输出数组来存储腐蚀操作的结果
示例
在以下示例中,我们通过缩减其像素强度来对图像执行条件腐蚀:
import mahotas as mh import numpy as np import matplotlib.pyplot as plt image= mh.imread('nature.jpeg') # Define the scaling factor scale_factor = 0.5 # Scale down the intensity by multiplying with the scale factor scaled_image = image * scale_factor # Convert the scaled image to the appropriate data type scaled_image = scaled_image.astype(np.uint8) conditional_eroded_image = mh.cerode(image, scaled_image) # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(7,5 )) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the conditional eroded image axes[1].imshow(conditional_eroded_image, cmap='gray') axes[1].set_title('Conditional Eroded Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
执行上述代码后,我们将获得以下输出:
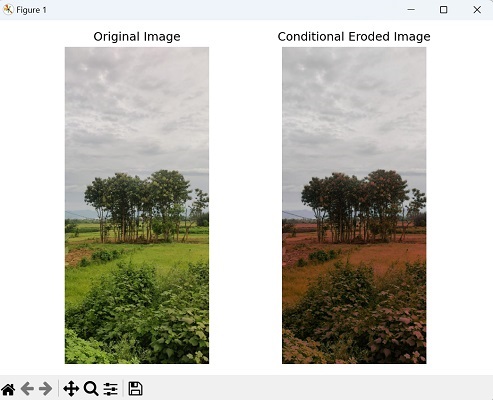
使用结构元素
要在 Mahotas 中使用结构元素执行条件腐蚀,首先,我们需要定义基于其应用腐蚀的条件。例如,您可以根据像素强度指定条件或提供二值掩码。
接下来,选择一个结构元素来定义腐蚀的邻域。Mahotas 提供了几个预定义的结构元素,例如圆盘和正方形,您可以根据所需的形状和大小进行选择。
最后,检索生成的图像,该图像将仅包含满足条件的位置的腐蚀效果。
示例
在这里,我们尝试使用结构元素对图像执行条件腐蚀:
import mahotas as mh import numpy as np import matplotlib.pyplot as plt image= mh.imread('nature.jpeg', as_grey = True).astype(np.uint8) # Define the condition based on pixel intensity condition = image > 0.5 # Define a structuring element for erosion structuring_element = mh.disk(5) conditional_eroded_image = mh.cerode(image, condition, structuring_element) # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(7,5 )) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the conditional eroded image axes[1].imshow(conditional_eroded_image, cmap='gray') axes[1].set_title('Conditional Eroded Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
获得的输出如下所示:
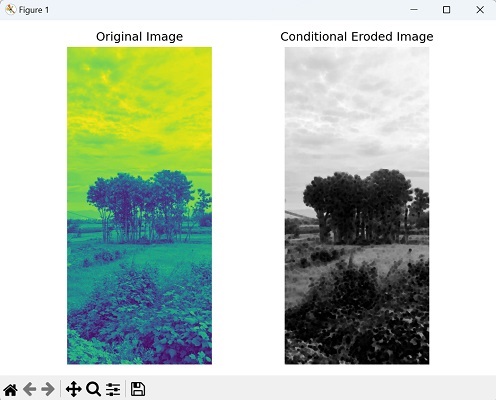
使用自定义条件函数
我们还可以使用自定义条件函数对图像执行条件腐蚀。
为此,我们首先定义一个自定义条件函数,该函数确定哪些像素应该进行腐蚀。
接下来,选择一个结构元素来定义腐蚀操作的形状和大小。最后,执行条件腐蚀并检索腐蚀图像。
示例
现在,我们使用自定义条件函数膨胀图像:
import mahotas as mh import numpy as np import matplotlib.pyplot as plt # Load image image = mh.imread('sea.bmp', as_grey=True).astype(np.uint8) # Define a custom condition function def custom_condition(pixel_value): return pixel_value > 0.5 # Define a structuring element structuring_element = mh.disk(5) # Create a binary mask based on the custom condition function condition = custom_condition(image) # Perform conditional erosion conditional_eroded_image = mh.cerode(image, condition, structuring_element) # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(7,5 )) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the conditional eroded image axes[1].imshow(conditional_eroded_image, cmap='gray') axes[1].set_title('Conditional Eroded Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
以下是上述代码的输出:
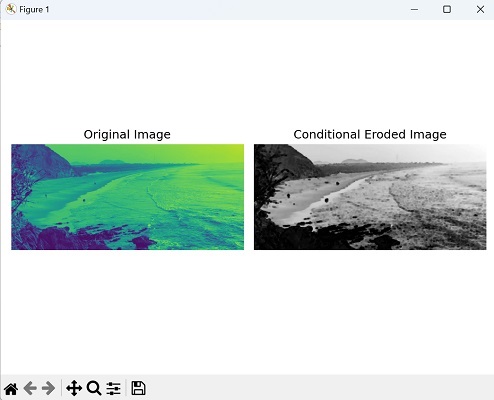