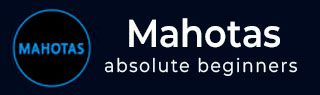
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 以灰度加载图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像RGB拉伸
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB到灰度转换
- Mahotas - RGB到LAB转换
- Mahotas - RGB到褐色转换
- Mahotas - RGB到XYZ转换
- Mahotas - XYZ到LAB转换
- Mahotas - XYZ到RGB转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标签图像函数
- Mahotas - 标签图像函数
- Mahotas - 图像标记
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 求图像平均值
- Mahotas - 裁剪图像
- Mahotas - 图像离心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 图像缩放
- Mahotas - 图像直方图
- Mahotas - 图像膨胀
- Mahotas - 图像腐蚀
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 条件分水岭图像
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen局部阈值化
- Mahotas - 小波变换
- 制作小波中心图像
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralick特征
- 标记区域的权重
- Mahotas - Zernike特征
- Mahotas - Zernike矩
- Mahotas - 排序滤波器
- Mahotas - 二维拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu方法
- Mahotas - 高斯滤波
- Mahotas - Hit & Miss变换
- Mahotas - 标记最大值数组
- Mahotas - 图像平均值
- Mahotas - SURF密集点
- Mahotas - SURF积分图像
- Mahotas - Haar变换
- 突出图像最大值
- 计算线性二值模式
- 获取标签边界
- 反转Haar变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 移除边界标记
- Mahotas - Daubechies小波
- Mahotas - Sobel边缘检测
Mahotas - 分水岭算法
在图像处理中,分水岭算法用于图像分割,这是将图像分割成不同区域的过程。分水岭算法尤其适用于分割具有不均匀区域或边界不明确的区域的图像。
分水岭算法的灵感来自于水文学中的分水岭概念,水从高处流向低处,沿山脊流淌,直到到达最低点,形成盆地。
同样,在图像处理中,该算法将图像的灰度值视为地形表面,其中高强度值代表峰值,低强度值代表谷值。
分水岭算法的工作原理
以下是分水岭算法在图像处理中工作原理的概述:
图像预处理:将输入图像转换为灰度图像(如果它不是灰度图像)。执行任何必要的预处理步骤,例如噪声去除或平滑处理,以改善结果。
梯度计算:计算图像的梯度以识别强度变化。这可以通过使用梯度算子(例如Sobel算子)来突出边缘。
标记生成:识别将用于启动分水岭算法的标记。这些标记通常对应于图像中感兴趣的区域。
它们可以由用户手动指定,也可以根据某些标准(例如局部最小值或聚类算法)自动生成。
标记标记:使用不同的整数值标记图像中的标记,表示不同的区域。
分水岭变换:使用标记的标记计算图像的分水岭变换。这是通过模拟洪水过程来完成的,其中强度值从标记传播并填充图像,直到它们在不同区域的边界处相遇。
结果是一个灰度图像,其中区域之间的边界被突出显示。
后处理:分水岭变换可能会产生过度分割的区域。可以应用后处理步骤(例如合并或平滑)来细化分割结果并获得最终的分割图像。
Mahotas中的分水岭算法
Mahotas库没有直接应用传统分水岭算法于图像的函数。但是,Mahotas提供了一种类似的技术,称为条件分水岭,我们将在后面的章节中详细探讨和讨论。
为了更好地理解传统的分割算法,让我们来看一个使用cv2库进行图像分割的例子。
示例
在下面的示例中,我们应用Otsu阈值化、形态学运算和距离变换来预处理图像并获得前景和背景区域。
然后,我们使用基于标记的分水岭分割来标记区域,并在分割图像中将边界标记为红色。
import cv2 import numpy as np from matplotlib import pyplot as plt image = cv2.imread('tree.tiff') # Convert the image to grayscale gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Apply Otsu's thresholding _, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU) # Perform morphological opening for noise removal kernel = np.ones((3, 3), np.uint8) opening = cv2.morphologyEx(thresh, cv2.MORPH_OPEN, kernel, iterations=2) # Perform distance transform dist_transform = cv2.distanceTransform(opening, cv2.DIST_L2, 5) # Threshold the distance transform to obtain sure foreground _, sure_fg = cv2.threshold(dist_transform, 0.7 * dist_transform.max(), 255, 0) sure_fg = np.uint8(sure_fg) # Determine the sure background region sure_bg = cv2.dilate(opening, kernel, iterations=3) # Determine the unknown region unknown = cv2.subtract(sure_bg, sure_fg) # Label the markers for watershed _, markers = cv2.connectedComponents(sure_fg) markers = markers + 1 markers[unknown == 255] = 0 # Apply watershed algorithm markers = cv2.watershed(image, markers) image[markers == -1] = [255, 0, 0] # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(7,5 )) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the watershed image axes[1].imshow(markers) axes[1].set_title('Watershed Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
以下是上述代码的输出:
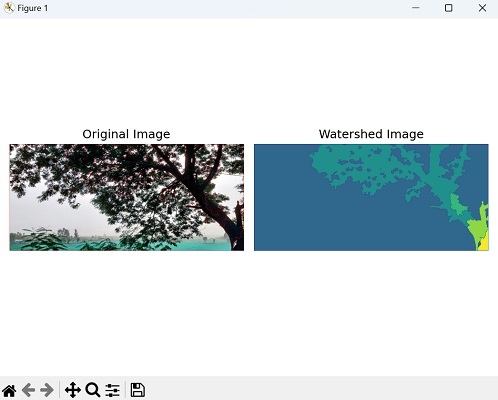