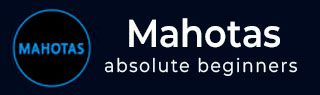
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 处理图像
- Mahotas - 处理图像
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB转灰度转换
- Mahotas - RGB转LAB转换
- Mahotas - RGB转棕褐色
- Mahotas - RGB转XYZ转换
- Mahotas - XYZ转LAB转换
- Mahotas - XYZ转RGB转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 标记图像
- Mahotas - 区域过滤
- Mahotas - 边界像素
- Mahotas - 形态学操作
- Mahotas - 形态学运算符
- Mahotas - 查找图像平均值
- Mahotas - 裁剪图像
- Mahotas - 图像偏心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 调整图像大小
- Mahotas - 图像直方图
- Mahotas - 膨胀图像
- Mahotas - 腐蚀图像
- Mahotas - 分水岭
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen 局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形实用程序
- Mahotas - 局部二值模式
- 阈值邻接统计
- Mahotas - Haralic 特征
- 标记区域的权重
- Mahotas - Zernike 特征
- Mahotas - Zernike 矩
- Mahotas - 排序滤波器
- Mahotas - 2D 拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu 方法
- Mahotas - 高斯滤波
- Mahotas - 击中与错过变换
- Mahotas - 标记最大数组
- Mahotas - 图像的平均值
- Mahotas - SURF 密集点
- Mahotas - SURF 积分
- Mahotas - Haar 变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签的边界
- 反转 Haar 变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 删除带边框的标签
- Mahotas - Daubechies 小波
- Mahotas - Sobel 边缘检测
Mahotas - 区域过滤
区域过滤指的是根据某些条件排除标记图像的特定区域。基于区域大小是常用的区域过滤条件。通过指定大小限制,可以排除过小或过大的区域,从而获得干净的输出图像。
另一个区域过滤标准是检查区域是否为边界。通过应用这些过滤器,我们可以选择性地移除或保留图像中感兴趣的区域。
在 Mahotas 中进行区域过滤
在 Mahotas 中,我们可以使用labeled.filter_labeled() 函数转换标记图像的过滤器区域。此函数将过滤器应用于图像的选定区域,同时保持其他区域不变。
使用 mahotas.labeled.filter_labeled() 函数
mahotas.labeled.filter_labeled() 函数以标记图像作为输入,并根据某些属性移除不需要的区域。它根据图像的标签识别区域。
结果图像仅包含与过滤器条件匹配的区域。
语法
以下是 Mahotas 中 filter_labeled() 函数的基本语法:
mahotas.labeled.filter_labeled(labeled, remove_bordering=False, min_size=None, max_size=None)
其中,
labeled - 它是一个数组。
remove_bordering (可选) - 它定义是否移除接触边界的区域。
min_size (可选) - 这是需要保留的区域的最小大小(默认情况下没有最小值)。
max_size (可选) - 这是需要保留的区域的最大大小(默认情况下没有最大值)。
示例
在以下示例中,我们正在过滤标记图像以移除边界像素。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('tree.tiff') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Converting it to a labeled image labeled, num_objects = mh.label(image) # Applying filters filtered_image, num_objects = mh.labeled.filter_labeled(labeled, remove_bordering=True) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the filtered image axes[1].imshow(filtered_image) axes[1].set_title('Filtered Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
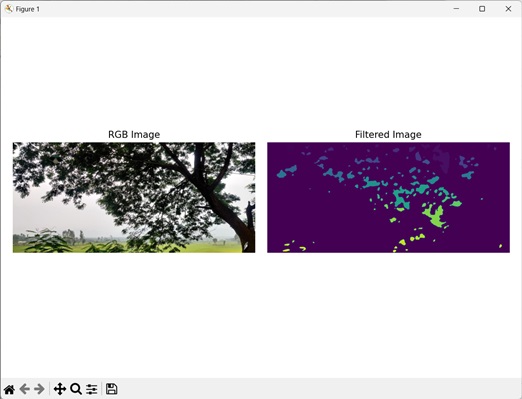
特定大小区域的过滤
我们还可以过滤图像中特定大小的区域。通过这种方式,我们可以从标记图像中移除不符合特定大小限制的区域(过小或过大的区域)。
在 mahotas 中,我们可以通过在 labeled.filter_label() 函数中为可选参数min_size 和max_size 指定值来实现此目的。
示例
以下示例显示了过滤标记图像以移除特定大小区域。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('tree.tiff') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Converting to a labeled image labeled, num_objects = mh.label(image) # Applying filters filtered_image, num_objects = mh.labeled.filter_labeled(labeled, min_size=10, max_size=50000) # Create a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the filtered image axes[1].imshow(filtered_image) axes[1].set_title('Filtered Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
上述代码的输出如下:
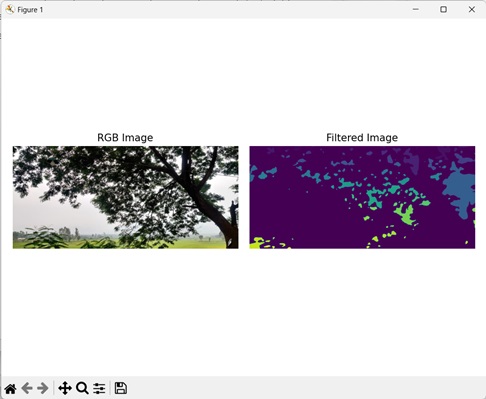
边界区域和特定大小区域的过滤
我们可以过滤图像中的边界区域以及特定大小的区域。在此,我们移除接触边界的区域以及不符合特定大小限制的区域。
在 mahotas 中,我们可以通过为可选参数min_size 和max_size 指定值,并将可选参数remove_bordering 设置为True 来实现此目的,这些参数都在 labeled.filter_label() 函数中。
示例
在此示例中,应用过滤器以移除标记图像的边界区域和特定大小的区域。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('tree.tiff') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Converting it to a labeled image labeled, num_objects = mh.label(image) # Applying filters filtered_image, num_objects = mh.labeled.filter_labeled(labeled, remove_bordering=True, min_size=1000, max_size=50000) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the filtered image axes[1].imshow(filtered_image) axes[1].set_title('Filtered Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
产生的输出如下所示:
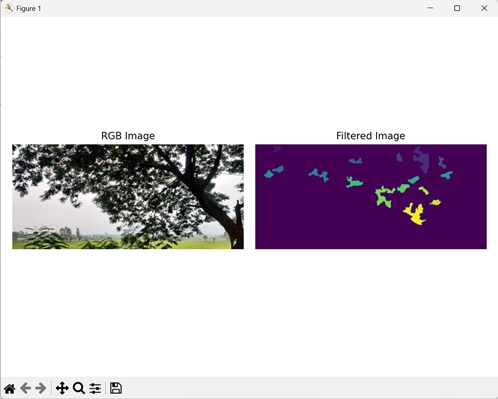