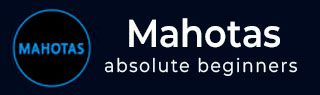
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 以灰度加载图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB转灰度转换
- Mahotas - RGB转LAB转换
- Mahotas - RGB转棕褐色
- Mahotas - RGB转XYZ转换
- Mahotas - XYZ转LAB转换
- Mahotas - XYZ转RGB转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标签图像函数
- Mahotas - 标签图像函数
- Mahotas - 图像标记
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 查找图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像离心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 调整图像大小
- Mahotas - 图像直方图
- Mahotas - 膨胀图像
- Mahotas - 腐蚀图像
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭算法
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralick特征
- 标记区域的权重
- Mahotas - Zernike特征
- Mahotas - Zernike矩
- Mahotas - 排序滤波器
- Mahotas - 2D拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu方法
- Mahotas - 高斯滤波
- Mahotas - Hit & Miss变换
- Mahotas - 标记最大值数组
- Mahotas - 图像均值
- Mahotas - SURF密集点
- Mahotas - SURF积分图
- Mahotas - Haar变换
- 突出图像最大值
- 计算线性二值模式
- 获取标签边界
- 反转Haar变换
- Riddler-Calvard方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 去除带边框的标记
- Mahotas - Daubechies小波
- Mahotas - Sobel边缘检测
Mahotas - 软阈值
软阈值是指降低图像的噪声(降噪)以提高其质量。
它根据像素与阈值的接近程度,为像素分配一个连续的值范围。这导致前景和背景区域之间逐渐过渡。
在软阈值中,阈值决定了降噪和图像保存之间的平衡。较高的阈值会导致更强的降噪,但会导致信息丢失。
相反,较低的阈值保留更多信息,但会导致不需要的噪声。
Mahotas中的软阈值
在Mahotas中,我们可以使用thresholding.soft_threshold()函数对图像应用软阈值。它根据相邻像素动态调整阈值,以增强具有非均匀噪声水平的图像。
通过使用动态调整,该函数按比例降低那些强度超过阈值的像素的强度,并将它们分配给前景。
另一方面,如果像素的强度低于阈值,则将其分配给背景。
mahotas.thresholding.soft_threshold() 函数
mahotas.thresholding.soft_threshold() 函数接收灰度图像作为输入,并返回已应用软阈值的图像。它的工作原理是将像素强度与提供的阈值进行比较。
语法
以下是Mahotas中soft_threshold()函数的基本语法:
mahotas.thresholding.soft_threshold(f, tval)
其中,
f - 输入灰度图像。
tval - 阈值。
示例
在下面的示例中,我们使用mh.thresholding.soft_threshold()函数对灰度图像应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Setting threshold value tval = 150 # Applying soft threshold on the image threshold_image = mh.thresholding.soft_threshold(image, tval) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the threshold image axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
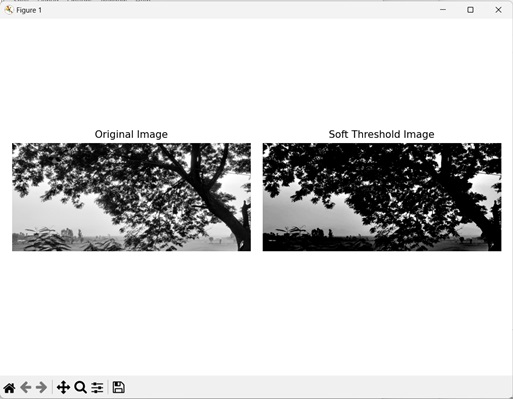
使用均值进行软阈值
我们可以使用图像上像素强度的均值来应用软阈值。均值是指图像的平均强度。
它是通过将所有像素的强度值相加,然后除以像素总数来计算的。
在Mahotas中,我们可以使用numpy.mean()函数找到图像所有像素的平均像素强度。然后,可以将均值传递给mahotas.thresholding.soft_threshold()函数的tval参数以生成软阈值图像。
这种应用软阈值的方法在降噪和图像质量之间保持了良好的平衡,因为阈值既不太高也不太低。
示例
下面的示例显示了当阈值为像素强度均值时,对灰度图像应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Setting mean threshold value tval = np.mean(image) # Applying soft threshold on the image threshold_image = mh.thresholding.soft_threshold(image, tval) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the threshold image axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
上述代码的输出如下:
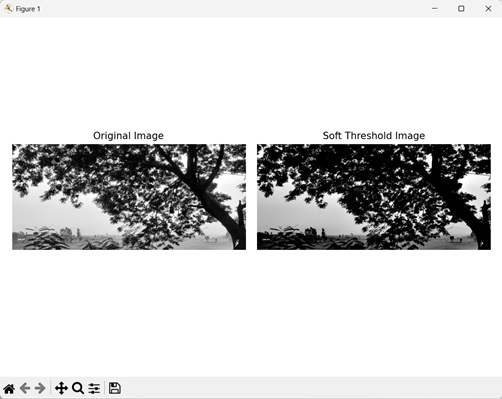
使用百分位数进行软阈值
除了均值,我们还可以使用图像像素强度的百分位数来应用软阈值。百分位数是指低于该值的数据所占的百分比;在图像处理中,它指的是图像中像素强度的分布。
例如,让我们将阈值百分位数设置为85。这意味着只有强度大于图像中其他像素的85%的像素才会被分类为前景,而其余像素会被分类为背景。
在Mahotas中,我们可以使用numpy.percentile()函数根据像素强度的百分位数设置阈值。然后,此值用于soft_thresholding()函数对图像应用软阈值。
示例
在这个例子中,我们展示了当使用百分位数查找阈值时如何应用软阈值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Setting percentile threshold value tval = np.percentile(image, 85) # Applying soft threshold on the image threshold_image = mh.thresholding.soft_threshold(image, tval) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the threshold image axes[1].imshow(threshold_image, cmap='gray') axes[1].set_title('Soft Threshold Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
执行上述代码后,我们将得到以下输出:
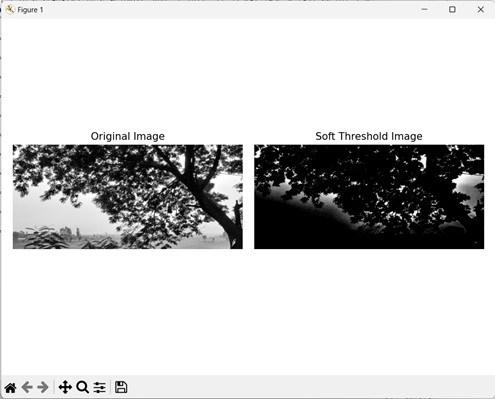