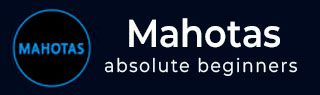
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建 RGB 图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸 RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB 到灰度转换
- Mahotas - RGB 到 LAB 转换
- Mahotas - RGB 到 Sepia 转换
- Mahotas - RGB 到 XYZ 转换
- Mahotas - XYZ 到 LAB 转换
- Mahotas - XYZ 到 RGB 转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 图像标记
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 求图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像离心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 图像缩放
- Mahotas - 图像直方图
- Mahotas - 图像膨胀
- Mahotas - 图像腐蚀
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 条件分水岭算法
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen 局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralic 特征
- 标记区域的权重
- Mahotas - Zernike 特征
- Mahotas - Zernike 矩
- Mahotas - 排序滤波器
- Mahotas - 2D 拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu 方法
- Mahotas - 高斯滤波
- Mahotas - Hit & Miss 变换
- Mahotas - 标记最大值数组
- Mahotas - 图像均值
- Mahotas - SURF 密集点
- Mahotas - SURF 积分图
- Mahotas - Haar 变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签边界
- 反转 Haar 变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 移除边界标记
- Mahotas - Daubechies 小波
- Mahotas - Sobel 边缘检测
Mahotas - 图像标记
图像标记是指将类别(标签)分配给图像的不同区域。标签通常用整数值表示,每个值对应于特定类别或区域。
例如,让我们考虑一个包含各种物体或区域的图像。每个区域都被分配一个唯一的值(整数)以将其与其他区域区分开来。背景区域的标签值为 0。
在 Mahotas 中标记图像
在 Mahotas 中,我们可以使用 label() 或 labeled.label() 函数来标记图像。
这些函数通过为图像中不同的连通组件分配唯一的标签或标识符来将图像分割成不同的区域。每个连通组件是一组相邻的像素,它们共享一个共同的属性,例如强度或颜色。
标记过程创建一个图像,其中属于同一区域的像素被分配相同的标签值。
使用 mahotas.label() 函数
mahotas.label() 函数以图像作为输入,其中感兴趣的区域由前景(非零)值表示,背景由零表示。
该函数返回标记的数组,其中每个连通组件或区域都被分配一个唯一的整数标签。
label() 函数使用 8 连通性进行标记,这指的是图像中像素之间的关系,其中每个像素都与其周围的八个邻居(包括对角线)相连。
语法
以下是 mahotas 中 label() 函数的基本语法:
mahotas.label(array, Bc={3x3 cross}, output={new array})
其中:
array - 输入数组。
Bc (可选) - 用于连通性的结构元素。
output (可选) - 输出数组(默认为与 array 形状相同的新的数组)。
示例
在下面的示例中,我们使用 mh.label() 函数来标记图像。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('sun.png') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Converting it to a labeled image labeled, num_objects = mh.label(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the labeled image axes[1].imshow(labeled) axes[1].set_title('Labeled Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
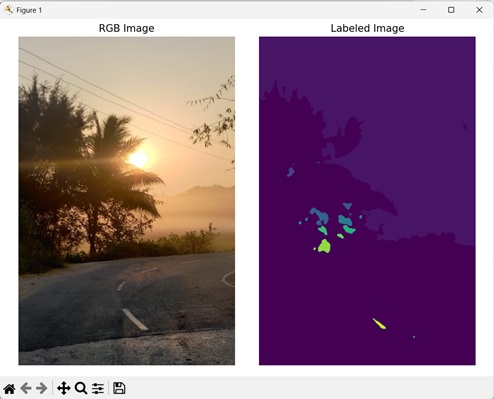
使用 mahotas.labeled.label() 函数
mahotas.labeled.label() 函数为图像的不同区域分配从 1 开始的连续标签。它与 mahotas.label() 函数类似,用于将图像分割成不同的区域。
如果你的标记图像具有非连续的标签值,labeled.label() 函数会将标签值更新为连续顺序。
例如,假设我们有一个标记图像,其中四个区域的标签分别为 2、4、7 和 9。labeled.label() 函数会将图像转换为一个新的标记图像,其连续标签分别为 1、2、3 和 4。
语法
以下是 mahotas 中 labeled.label() 函数的基本语法:
mahotas.labeled.label(array, Bc={3x3 cross}, output={new array})
其中:
array - 输入数组。
Bc (可选) - 用于连通性的结构元素。
output (可选) - 输出数组(默认为与 array 形状相同的新的数组)。
示例
以下示例演示了如何使用 mh.labeled.label() 函数将图像转换为标记图像。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('sea.bmp') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Converting it to a labeled image labeled, num_objects = mh.labeled.label(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the labeled image axes[1].imshow(labeled) axes[1].set_title('Labeled Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
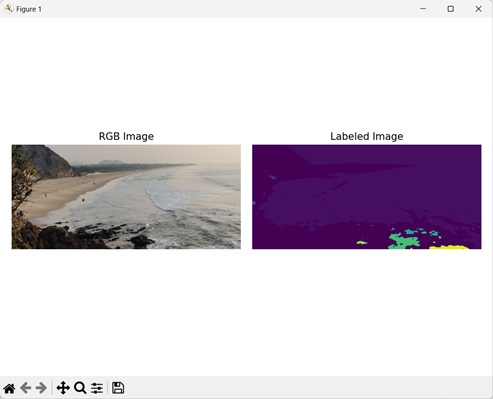
使用自定义结构元素
我们可以使用自定义结构元素和标签函数根据需要分割图像。结构元素是一个奇数维的二元数组,由 1 和 0 组成,它定义了图像标记过程中邻域像素的连接模式。
1 表示连接分析中包含的相邻像素,而 0 表示排除或忽略的邻居。
例如,让我们考虑自定义结构元素:[[1, 0, 0], [0, 1, 0], [0, 0, 1]]。 这个结构元素意味着对角线连接。这意味着对于图像中的每个像素,在标记或分割过程中,只有对角线向上和向下像素被认为是其邻居。
示例
在这里,我们定义了一个自定义结构元素来标记图像。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image_rgb = mh.imread('sea.bmp') image = image_rgb[:,:,0] # Applying gaussian filtering image = mh.gaussian_filter(image, 4) image = (image > image.mean()) # Creating a custom structuring element binary_closure = np.array([[0, 1, 0], [0, 1, 0], [0, 1, 0]]) # Converting it to a labeled image labeled, num_objects = mh.labeled.label(image, Bc=binary_closure) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image_rgb) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the labeled image axes[1].imshow(labeled) axes[1].set_title('Labeled Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figure mtplt.show()
输出
执行上述代码后,我们将得到以下输出:
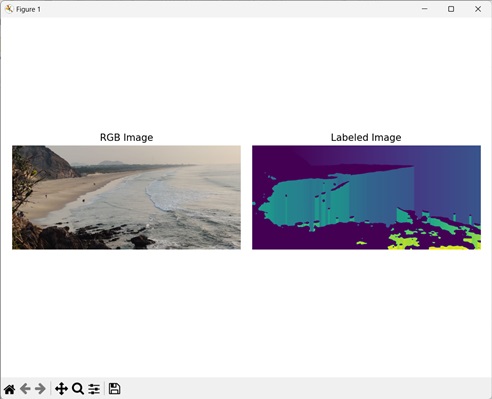