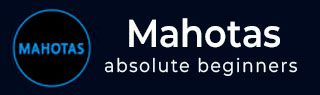
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 处理图像
- Mahotas - 处理图像
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像的质心
- Mahotas - 图像卷积
- Mahotas - 创建 RGB 图像
- Mahotas - 图像的欧拉数
- Mahotas - 图像中零值的比例
- Mahotas - 获取图像矩
- Mahotas - 图像中的局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸 RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB 到灰度转换
- Mahotas - RGB 到 LAB 转换
- Mahotas - RGB 到 Sepia 转换
- Mahotas - RGB 到 XYZ 转换
- Mahotas - XYZ 到 LAB 转换
- Mahotas - XYZ 到 RGB 转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标签图像函数
- Mahotas - 标签图像函数
- Mahotas - 图像标记
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学操作
- Mahotas - 形态学算子
- Mahotas - 查找图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像的偏心率
- Mahotas - 图像叠加
- Mahotas - 图像的圆形度
- Mahotas - 图像缩放
- Mahotas - 图像直方图
- Mahotas - 图像膨胀
- Mahotas - 图像腐蚀
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像孔洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭
- Mahotas - 图像中的局部最小值
- Mahotas - 图像的区域最大值
- Mahotas - 图像的区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen 局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形实用程序
- Mahotas - 局部二值模式
- 阈值邻接统计
- Mahotas - Haralic 特征
- 标记区域的权重
- Mahotas - Zernike 特征
- Mahotas - Zernike 矩
- Mahotas - 排序滤波器
- Mahotas - 2D 拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu 方法
- Mahotas - 高斯滤波
- Mahotas - 击中或错过变换
- Mahotas - 标记最大数组
- Mahotas - 图像的平均值
- Mahotas - SURF 密集点
- Mahotas - SURF 积分
- Mahotas - Haar 变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签的边界
- 反转 Haar 变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 去除带边框的标记
- Mahotas - Daubechies 小波
- Mahotas - Sobel 边缘检测
Mahotas - 均值滤波器
均值滤波器用于平滑图像以减少噪声。它的工作原理是计算指定邻域内所有像素的平均值,然后用平均值替换原始像素的值。
假设我们有一个具有不同强度值的灰度图像。因此,某些像素可能具有比其他像素更高的强度值。
因此,均值滤波器用于通过稍微模糊图像来创建像素的均匀外观。
Mahotas 中的均值滤波器
要在 mahotas 中应用均值滤波器,我们可以使用 **mean_filter()** 函数。
Mahotas 中的均值滤波器使用结构元素来检查邻域中的像素。
结构元素将每个像素值替换为其相邻像素的平均值。
结构元素的大小决定了平滑的程度。更大的邻域会导致更强的平滑效果,同时会减少一些更精细的细节,而较小的邻域会导致平滑效果较弱,但会保留更多细节。
mahotas.mean_filter() 函数
mean_filter() 函数使用指定的邻域大小将均值滤波器应用于输入图像。
它用其邻居中的多数值替换每个像素值。滤波后的图像存储在输出数组中。
语法
以下是 mahotas 中 mean filter() 函数的基本语法:
mahotas.mean_filter(f, Bc, mode='ignore', cval=0.0, out=None)
其中,
**img** - 输入图像。
**Bc** - 定义邻域的结构元素。
**mode(可选)** - 指定函数如何处理图像的边界。它可以取不同的值,例如“reflect”、“constant”、“nearest”、“mirror”或“wrap”。
默认情况下,它设置为“ignore”,这意味着滤波器会忽略图像边界之外的像素。
**cval(可选)** - 当 mode='constant' 时要使用的值。默认值为 0.0。
**out(可选)** - 指定将存储滤波后图像的输出数组。它必须与输入图像具有相同的形状。
示例
以下是使用 mean_filter() 函数滤波图像的基本示例:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('tree.tiff', as_grey = True) structuring_element = mh.disk(12) filtered_image = mh.mean_filter(image, structuring_element) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the mean filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Mean Filtered') axes[1].axis('off') mtplt.show()
输出
执行上述代码后,我们将得到以下输出:
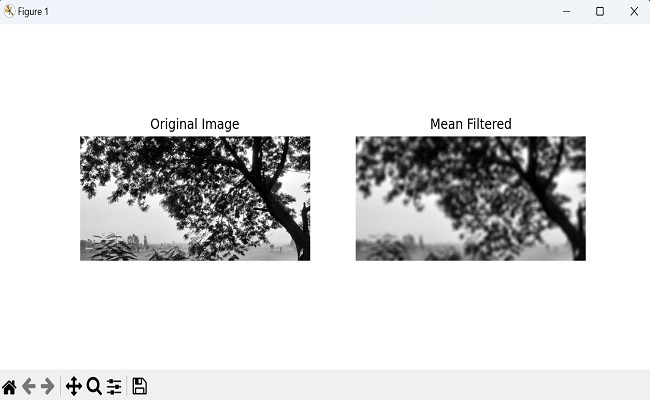
使用反射模式的均值滤波器
当我们将均值滤波器应用于图像时,我们需要考虑每个像素周围的相邻像素来计算平均值。但是,在图像的边缘,有一些像素在一侧或多侧没有邻居。
为了解决这个问题,我们使用 **'reflect'** 模式。反射模式沿着图像边缘创建镜像效果。它允许我们通过镜像方式复制其像素来虚拟地扩展图像。
这样,我们即使在边缘也可以为均值滤波器提供相邻像素。
通过反射图像值,均值滤波器现在可以将这些镜像像素视为真实的邻居。
它使用这些虚拟邻居计算平均值,从而在图像边缘产生更准确的平滑过程。
示例
在这里,我们尝试使用反射模式计算均值滤波器:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('nature.jpeg', as_grey = True) structuring_element = mh.morph.dilate(mh.disk(12), Bc=mh.disk(12)) filtered_image = mh.mean_filter(image, structuring_element, mode='reflect') # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the mean filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Mean Filtered') axes[1].axis('off') mtplt.show()
输出
上述代码的输出如下:
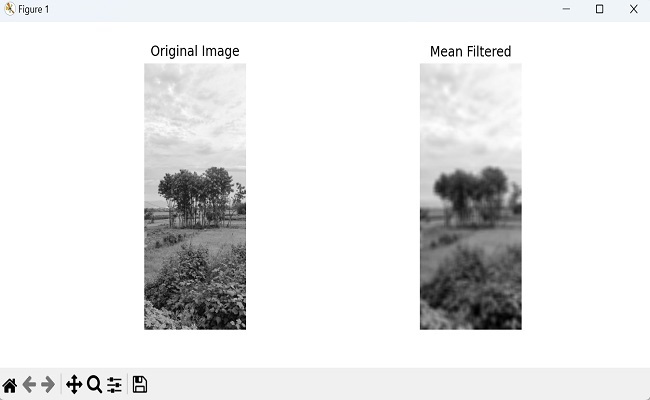
通过将结果存储在输出数组中
我们也可以使用 Mahotas 将均值滤波器的结果存储在输出数组中。为此,我们首先需要使用 NumPy 库创建一个空数组。
此数组初始化为与输入图像相同的形状,以存储生成的滤波图像。数组的数据类型指定为 float(默认值)。
最后,我们通过将其作为参数传递给 mean_filter() 函数,将生成的滤波图像存储在输出数组中。
示例
现在,我们尝试将均值滤波器应用于灰度图像并将结果存储在特定的输出数组中:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('pic.jpg', as_grey = True) # Create an output array for the filtered image output = np.empty(image.shape) # store the result in the output array mh.mean_filter(image, Bc=mh.disk(12), out=output) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the mean filtered image axes[1].imshow(output, cmap='gray') axes[1].set_title('Mean Filtered') axes[1].axis('off') mtplt.show()
输出
以下是上述代码的输出:
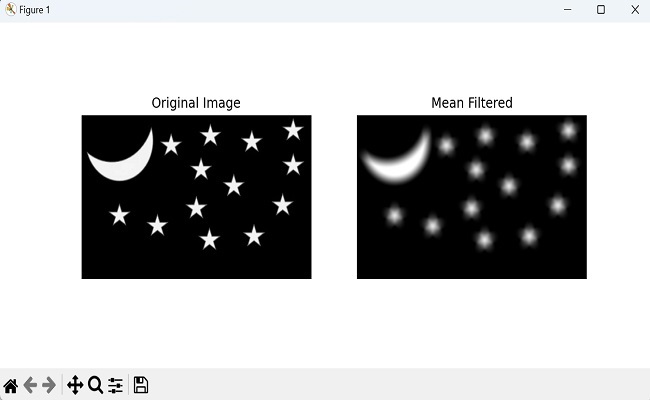