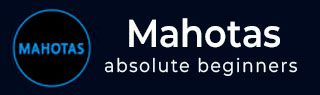
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 处理图像
- Mahotas - 处理图像
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像的质心
- Mahotas - 图像卷积
- Mahotas - 创建 RGB 图像
- Mahotas - 图像的欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像中的局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸 RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB 转灰度
- Mahotas - RGB 转 LAB
- Mahotas - RGB 转褐色
- Mahotas - RGB 转 XYZ
- Mahotas - XYZ 转 LAB
- Mahotas - XYZ 转 RGB
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 标记图像
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 查找图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像的偏心率
- Mahotas - 叠加图像
- Mahotas - 图像的圆度
- Mahotas - 调整图像大小
- Mahotas - 图像的直方图
- Mahotas - 膨胀图像
- Mahotas - 腐蚀图像
- Mahotas - 分水岭
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像孔洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭
- Mahotas - 图像中的局部最小值
- Mahotas - 图像的区域最大值
- Mahotas - 图像的区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen 局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形实用程序
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralic 特征
- 标记区域的权重
- Mahotas - Zernike 特征
- Mahotas - Zernike 矩
- Mahotas - 排序滤波器
- Mahotas - 2D 拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu 方法
- Mahotas - 高斯滤波
- Mahotas - 击中击不中变换
- Mahotas - 标记最大数组
- Mahotas - 图像的平均值
- Mahotas - SURF 密集点
- Mahotas - SURF 积分
- Mahotas - Haar 变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签的边界
- 反转 Haar 变换
- Riddler-Calvard 方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 删除带边框的标记
- Mahotas - Daubechies 小波
- Mahotas - Sobel 边缘检测
Mahotas - RGB 转灰度
图像处理中的 RGB 到灰度转换将 RGB 颜色空间中的彩色图像转换为灰度图像。
- RGB 图像由三个颜色通道组成——红色、绿色和蓝色。RGB 图像中的每个像素都由这三个通道的强度值的组合表示,从而产生广泛的颜色范围。
- 另一方面,灰度图像是单通道图像(仅包含(灰色)的阴影),其中每个像素表示原始图像中相应位置的强度。
- 强度值范围从黑色(0)到白色(255),中间是灰色的阴影。
Mahotas 中的 RGB 转灰度转换
在 Mahotas 中,我们可以使用 **colors.rgb2gray()** 函数将 RGB 图像转换为灰度图像。
该函数根据其 RGB 值的加权平均值计算每个像素的灰度强度。
权重反映了人类对颜色的感知,红色权重最高,其次是绿色,然后是蓝色。
mahotas.colors.rgb2gray() 函数
mahotas.colors.rgb2gray() 函数以 RGB 图像作为输入,并返回图像的灰度版本。
生成的灰度图像保留了原始 RGB 图像的结构和整体内容,但缺少颜色信息。
语法
以下是 mahotas 中 rgb2gray() 函数的基本语法:
mahotas.colors.rgb2gray(rgb_image, dtype=float)
其中,
**rgb_image** - 它是 RGB 颜色空间中的输入图像。
**dtype(可选)** - 它是返回图像的数据类型(默认为浮点数)。
示例
在以下示例中,我们使用 mh.colors.rgb2gray() 函数将 RGB 图像转换为灰度图像:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('nature.jpeg') # Converting it to grayscale gray_image = mh.colors.rgb2gray(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the grayscale image axes[1].imshow(gray_image, cmap='gray') axes[1].set_title('Grayscale Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
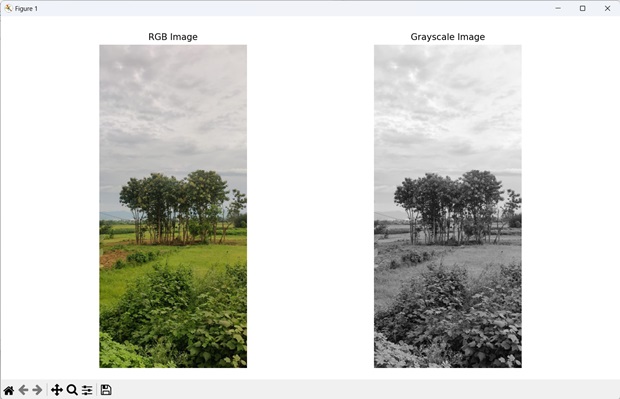
使用 RGB 通道的平均值
我们还可以使用 RGB 通道的平均值将 RGB 图像转换为灰度图像。
将每个像素的红色、绿色和蓝色的强度相加并除以三,即可获得平均强度值。我们可以使用 numpy 库的 mean() 函数实现这一点。
生成的图像将是灰度图像,具有单个通道,其中每个像素表示跨 RGB 通道的平均强度,其中每个颜色通道对整体灰度强度都有同等贡献。
示例
以下示例显示了使用 RGB 通道平均值将 RGB 图像转换为灰度的过程:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sun.png') # Converting it to grayscale gray_image = np.mean(image, axis=2).astype(np.uint8) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the grayscale image axes[1].imshow(gray_image, cmap='gray') axes[1].set_title('Grayscale Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
上述代码的输出如下:
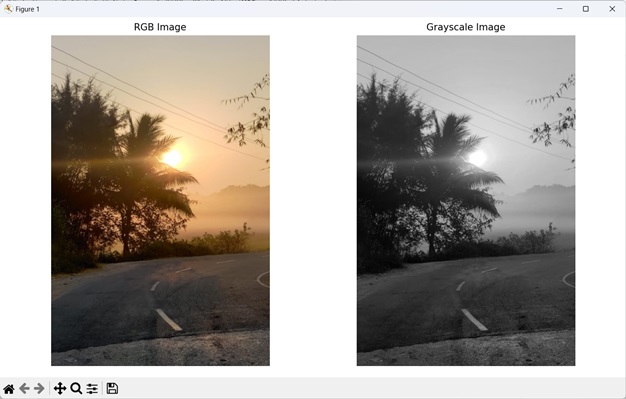
使用亮度
亮度是指对象或图像的感知亮度或亮度。
在图像处理中,亮度为每个像素的红色、绿色和蓝色颜色通道分配特定的权重,并将它们组合起来计算灰度强度值。
RGB 颜色空间中的亮度可以使用以下公式计算:
Luminosity = 0.2989 * R + 0.5870 * G + 0.1140 * B
其中,值 **0.2989、0.5870** 和 **0.1140** 分别是分配给红色、绿色和蓝色通道的权重。这些权重来自用于数字显示的标准 Rec.709 颜色空间。
与简单地平均 RGB 通道相比,此方法可产生更好的结果,因为它可以更好地捕捉原始图像的视觉感知。
示例
在这里,我们定义了 RGB 的亮度,以将彩色图像转换为其等效的灰度图像:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to gray gray_image = np.dot(image[..., :3], [0.2989, 0.5870, 0.1140]).astype(np.uint8) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original RGB image axes[0].imshow(image) axes[0].set_title('RGB Image') axes[0].set_axis_off() # Displaying the grayscale image axes[1].imshow(gray_image, cmap='gray') axes[1].set_title('Grayscale Image') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
执行上述代码后,我们将获得以下输出:
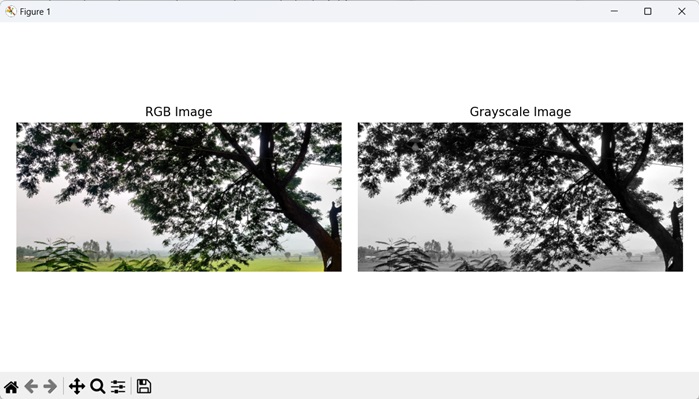