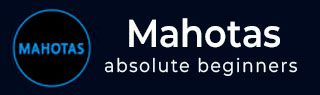
- Mahotas教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零值的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - RGB图像拉伸
- Mahotas颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB转灰度
- Mahotas - RGB转LAB
- Mahotas - RGB转棕褐色
- Mahotas - RGB转XYZ
- Mahotas - XYZ转LAB
- Mahotas - XYZ转RGB
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 标记图像
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学操作
- Mahotas - 形态学算子
- Mahotas - 查找图像平均值
- Mahotas - 裁剪图像
- Mahotas - 图像离心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 调整图像大小
- Mahotas - 图像直方图
- Mahotas - 图像膨胀
- Mahotas - 图像腐蚀
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像孔洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen局部阈值化
- Mahotas - 小波变换
- 创建图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralick特征
- 标记区域的权重
- Mahotas - Zernike特征
- Mahotas - Zernike矩
- Mahotas - 排序滤波器
- Mahotas - 二维拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu方法
- Mahotas - 高斯滤波
- Mahotas - 击中击不中变换
- Mahotas - 标记最大数组
- Mahotas - 图像平均值
- Mahotas - SURF密集点
- Mahotas - SURF积分
- Mahotas - Haar变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签边界
- 反转Haar变换
- Riddler-Calvard方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 移除带边框的标签
- Mahotas - Daubechies小波
- Mahotas - Sobel边缘检测
Mahotas - RGB图像拉伸
图像拉伸是一种通过将原始像素值映射到新的值范围来增强图像对比度的技术。这通常是为了提高细节的可视性并增强图像的整体外观。
对于RGB图像,每个像素都有三个颜色通道——红色、绿色和蓝色。拉伸RGB图像涉及独立地拉伸每个颜色通道的值以扩展强度范围。
Mahotas中的RGB图像拉伸
在Mahotas中,RGB图像拉伸是通过拉伸图像的各个颜色通道(红色、绿色和蓝色)来执行的。拉伸过程涉及将原始像素强度映射到新的范围以增加对比度。
拉伸各个RGB通道后,需要将它们组合起来以创建最终的拉伸图像。
我们可以使用stretch()函数和stretch_rgb()函数在mahotas中执行RGB图像拉伸。
使用stretch()和stretch_rgb()函数
mahotas.stretch()和mahotas.stretch_rgb()函数将RGB图像每个通道中的像素值拉伸到指定的min_value和max_value之间。
值低于min_value的像素将设置为min_value,而值高于max_value的像素将设置为max_value。拉伸在每个通道上独立执行。
语法
以下是mahotas.stretch()函数的基本语法:
mahotas.stretch(img,arg0=None, arg1=None, dtype=<type 'numpy.uint8'>)
以下是mahotas.stretch_rgb()函数的基本语法:
mahotas.stretch(img,arg0=None, arg1=None, dtype=<type 'numpy.uint8'>)
参数
以下是传递给mahotas.stretch()函数的参数:
img - 输入图像。
arg0和arg1(可选) - 这些参数指定像素值应拉伸到的范围。这些参数的解释取决于它们的值:
如果arg0和arg1都提供且不为None,则它们分别表示像素值应拉伸到的最小值和最大值。低于arg0的像素值将设置为arg0,高于arg1的像素值将设置为arg1。
如果仅提供arg0且arg1为None,则arg0应为长度为2的元组或列表,表示百分位数值。低于arg0第一个元素的值的像素值将设置为该值,高于arg0第二个元素的值的像素值将设置为该值。
如果arg0和arg1都为None,则像素值将拉伸到由dtype参数指定的数据类型的完整范围(默认值为numpy.uint8)。
dtype - 指定输出图像的数据类型。默认值为numpy.uint8,表示无符号8位整数。
示例
在以下示例中,我们使用mahotas.stretch()函数来拉伸图像:
import mahotas as mh import numpy as np import matplotlib.pyplot as plt image = mh.imread('sun.png') # Stretch image stretched = mh.stretch(image, arg0=100, arg1=280) # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(10, 5)) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the stretched grayscale image axes[1].imshow(stretched, cmap='gray') axes[1].set_title('Stretched Grayscale Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
以下是上述代码的输出:
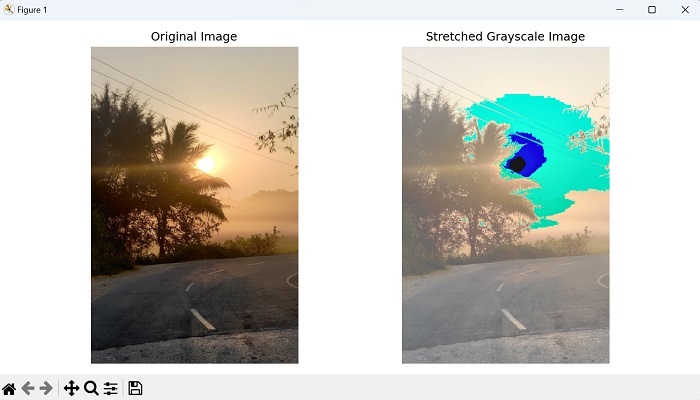
示例
在这个例子中,我们将看到如何使用mahotas.stretch_rgb函数来拉伸图像:
import mahotas as mh import numpy as np import matplotlib.pyplot as plt image = mh.imread('nature.jpeg') # Stretch image stretched = mh.stretch_rgb(image, arg0=100, arg1=280) # Create a figure with subplots fig, axes = plt.subplots(1, 2, figsize=(10, 5)) # Display the original image axes[0].imshow(image) axes[0].set_title('Original Image') axes[0].axis('off') # Display the stretched grayscale image axes[1].imshow(stretched, cmap='gray') axes[1].set_title('Stretched Grayscale Image') axes[1].axis('off') # Adjust the layout and display the plot plt.tight_layout() plt.show()
输出
以下是上述代码的输出:
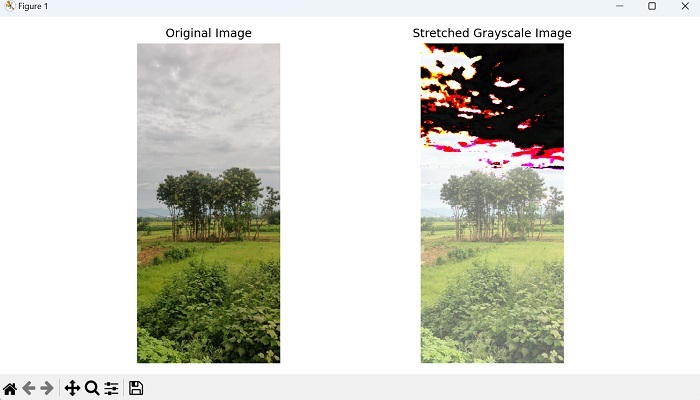
使用百分位数值拉伸图像
要在Mahotas中使用百分位数值拉伸RGB图像,我们可以手动计算百分位数并分别对每个颜色通道使用stretch函数:
- 首先,将RGB图像拆分为各个颜色通道(红色、绿色和蓝色)。
- 这可以通过使用NumPy库中的np.split()函数来实现。
- 一旦图像被拆分,使用NumPy的np.percentile()函数计算每个颜色通道所需的百分位数。
- 获得百分位数后,独立地对每个通道使用Mahotas的stretch()函数,使用计算出的最小值和最大值。
- 这将根据指定的百分位数范围拉伸每个通道中的像素值。
最后,通过沿颜色通道轴连接它们,将拉伸后的通道合并回RGB图像。生成的图像将是基于指定百分位数的拉伸RGB图像。
示例
在这里,我们尝试使用百分位数值拉伸图像:
import mahotas as mh import numpy as np from pylab import imshow, show image = mh.imread('nature.jpeg') # Splitting the RGB image into individual channels r, g, b = np.split(image, 3, axis=2) # Calculating percentiles for each channel r_min, r_max = np.percentile(r, [17, 90]) g_min, g_max = np.percentile(g, [25, 75]) b_min, b_max = np.percentile(b, [50, 99]) # Stretching each channel independently stretched_r = mh.stretch(r, r_min, r_max) stretched_g = mh.stretch(g, g_min, g_max) stretched_b = mh.stretch(b, b_min, b_max) # Merging the stretched channels back into an RGB image stretched_image = np.concatenate((stretched_r, stretched_g, stretched_b), axis=2) imshow(stretched_image) show()
输出
执行上述代码后,我们将得到以下输出:
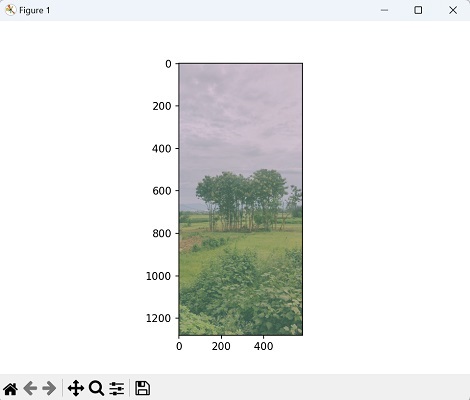