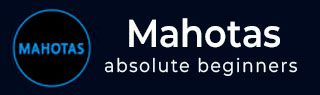
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 以灰度加载图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB转灰度转换
- Mahotas - RGB转LAB转换
- Mahotas - RGB转棕褐色
- Mahotas - RGB转XYZ转换
- Mahotas - XYZ转LAB转换
- Mahotas - XYZ转RGB转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标记图像函数
- Mahotas - 标记图像函数
- Mahotas - 标记图像
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 查找图像平均值
- Mahotas - 裁剪图像
- Mahotas - 图像偏心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 调整图像大小
- Mahotas - 图像直方图
- Mahotas - 膨胀图像
- Mahotas - 腐蚀图像
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭算法
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralic特征
- 标记区域的权重
- Mahotas - Zernike特征
- Mahotas - Zernike矩
- Mahotas - 排序滤波器
- Mahotas - 2D拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu方法
- Mahotas - 高斯滤波
- Mahotas - Hit & Miss变换
- Mahotas - 标记最大值数组
- Mahotas - 图像平均值
- Mahotas - SURF密集点
- Mahotas - SURF积分图像
- Mahotas - Haar变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签的边界
- 反转Haar变换
- Riddler-Calvard方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 去除带边框的标签
- Mahotas - Daubechies小波
- Mahotas - Sobel边缘检测
Mahotas - 中值滤波器
中值滤波器是另一种常用的图像降噪技术。它的工作原理是计算相邻像素的中值(中间值),并用该中值替换原始像素值。
为了理解中值滤波器,让我们考虑同样的黑白图像场景,其中小的黑点代表噪声。图像中的每个像素都有一个二值——白色(代表感兴趣的对象)或黑色(代表背景)。
对于每个像素,中值滤波器都会获取窗口内相邻像素的像素值。然后,它根据像素的强度值将它们按升序排列。
之后,它选择中间值(即中值),并用该中值替换原始像素值。
Mahotas中的中值滤波器
要在Mahotas中应用中值滤波器,可以使用`median_filter()`函数。
Mahotas中的中值滤波器函数使用结构元素来检查邻域中的像素。
结构元素通过计算其邻域内的中值来替换每个像素的值。
结构元素的大小决定了中值滤波器应用的平滑程度。
较大的邻域将产生更强的平滑效果,同时减少图像的精细细节。另一方面,较小的邻域将产生较少的平滑效果,但会保留更多细节。
`mahotas.median_filter()`函数
`median_filter()`函数使用指定的邻域大小对输入图像应用中值滤波器。它用在其邻居中计算的中值替换每个像素值。过滤后的图像存储在输出数组中。
语法
以下是Mahotas中中值滤波器函数的基本语法:
mahotas.median_filter(img, Bc={square}, mode='reflect', cval=0.0, out={np.empty(f.shape, f.dtype})
其中,
img - 输入图像。
Bc - 定义邻域的结构元素。默认情况下,它是一个边长为3的正方形。
mode (可选) - 指定函数如何处理图像的边界。它可以取不同的值,例如'reflect','constant','nearest','mirror'或'wrap'。默认设置为'reflect'。
cval (可选) - 当mode='constant'时使用的值。默认值为0.0。
out (可选) - 指定存储过滤后图像的输出数组。它必须与输入图像具有相同的形状和数据类型。
示例
以下是使用`median_filter()`函数过滤图像的基本示例:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('tree.tiff', as_grey = True) structuring_element = mh.disk(12) filtered_image = mh.median_filter(image, structuring_element) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
输出
执行上述代码后,我们将得到以下输出:
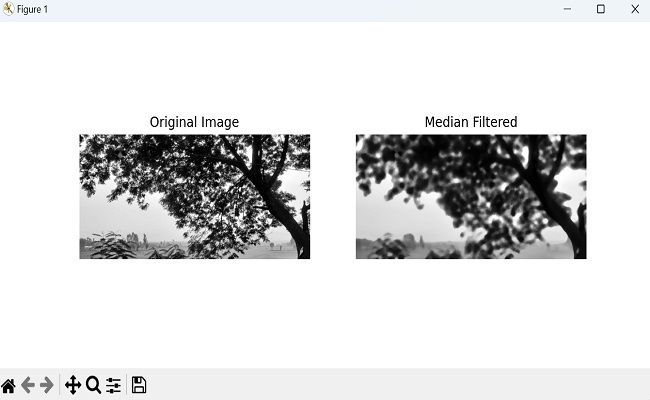
使用反射模式的中值滤波器
当我们将中值滤波器应用于图像时,我们需要考虑每个像素周围的相邻像素来计算中值。
但是,在图像的边缘,有些像素在一侧或多侧没有邻居。
为了解决这个问题,我们使用'reflect'模式。反射模式沿图像边缘创建镜像效果。它允许我们通过镜像方式复制像素来虚拟地扩展图像。
这样,我们即使在边缘也能为中值滤波器提供相邻像素。
通过反射图像值,中值滤波器现在可以将这些镜像像素视为真实的邻居。
它使用这些虚拟邻居计算中值,从而在图像边缘产生更精确的平滑过程。
示例
在这里,我们尝试使用反射模式计算中值滤波器:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('nature.jpeg', as_grey = True) structuring_element = mh.morph.dilate(mh.disk(12), Bc=mh.disk(12)) filtered_image = mh.median_filter(image, structuring_element, mode='reflect') # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(filtered_image, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
输出
上述代码的输出如下:
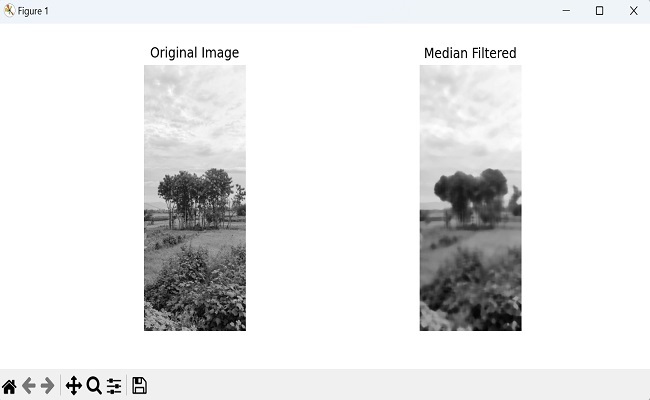
通过将结果存储在输出数组中
我们也可以使用Mahotas将中值滤波器的结果存储在输出数组中。为此,我们首先需要使用NumPy库创建一个空数组。
此数组使用与输入图像相同的形状和数据类型进行初始化,以存储生成的滤波图像。
最后,我们将生成的滤波图像存储在输出数组中,将其作为参数传递给`median_filter()`函数。
示例
现在,我们尝试将中值滤波器应用于灰度图像并将结果存储在特定的输出数组中:
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt image=mh.imread('pic.jpg', as_grey = True) # Create an output array for the filtered image output = np.empty(image.shape) # store the result in the output array mh.median_filter(image, Bc=mh.disk(12), out=output) # Displaying the original image fig, axes = mtplt.subplots(1, 2, figsize=(9, 4)) axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].axis('off') # Displaying the median filtered image axes[1].imshow(output, cmap='gray') axes[1].set_title('Median Filtered') axes[1].axis('off') mtplt.show()
输出
以下是上述代码的输出:
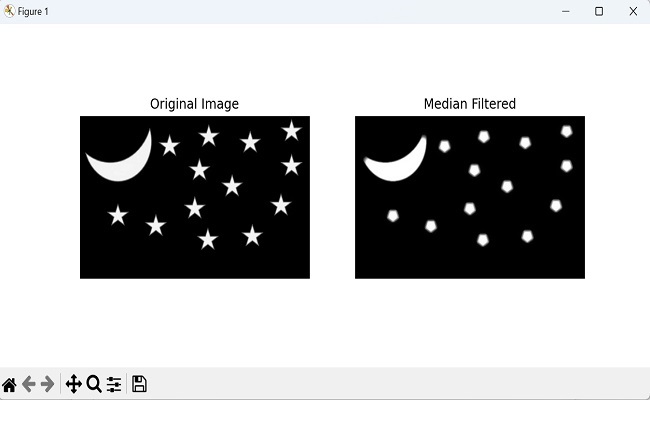