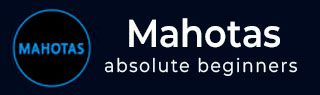
- Mahotas 教程
- Mahotas - 首页
- Mahotas - 简介
- Mahotas - 计算机视觉
- Mahotas - 历史
- Mahotas - 特性
- Mahotas - 安装
- Mahotas 图像处理
- Mahotas - 图像处理
- Mahotas - 加载图像
- Mahotas - 加载灰度图像
- Mahotas - 显示图像
- Mahotas - 显示图像形状
- Mahotas - 保存图像
- Mahotas - 图像质心
- Mahotas - 图像卷积
- Mahotas - 创建RGB图像
- Mahotas - 图像欧拉数
- Mahotas - 图像中零的比例
- Mahotas - 获取图像矩
- Mahotas - 图像局部最大值
- Mahotas - 图像椭圆轴
- Mahotas - 图像拉伸RGB
- Mahotas 颜色空间转换
- Mahotas - 颜色空间转换
- Mahotas - RGB到灰度转换
- Mahotas - RGB到LAB转换
- Mahotas - RGB到褐色转换
- Mahotas - RGB到XYZ转换
- Mahotas - XYZ到LAB转换
- Mahotas - XYZ到RGB转换
- Mahotas - 增加伽马校正
- Mahotas - 拉伸伽马校正
- Mahotas 标签图像函数
- Mahotas - 标签图像函数
- Mahotas - 图像标记
- Mahotas - 过滤区域
- Mahotas - 边界像素
- Mahotas - 形态学运算
- Mahotas - 形态学算子
- Mahotas - 查找图像均值
- Mahotas - 裁剪图像
- Mahotas - 图像偏心率
- Mahotas - 图像叠加
- Mahotas - 图像圆度
- Mahotas - 图像缩放
- Mahotas - 图像直方图
- Mahotas - 图像膨胀
- Mahotas - 图像腐蚀
- Mahotas - 分水岭算法
- Mahotas - 图像开运算
- Mahotas - 图像闭运算
- Mahotas - 填充图像空洞
- Mahotas - 条件膨胀图像
- Mahotas - 条件腐蚀图像
- Mahotas - 图像条件分水岭算法
- Mahotas - 图像局部最小值
- Mahotas - 图像区域最大值
- Mahotas - 图像区域最小值
- Mahotas - 高级概念
- Mahotas - 图像阈值化
- Mahotas - 设置阈值
- Mahotas - 软阈值
- Mahotas - Bernsen局部阈值化
- Mahotas - 小波变换
- 制作图像小波中心
- Mahotas - 距离变换
- Mahotas - 多边形工具
- Mahotas - 局部二值模式
- 阈值邻域统计
- Mahotas - Haralick特征
- 标记区域的权重
- Mahotas - Zernike特征
- Mahotas - Zernike矩
- Mahotas - 排序滤波器
- Mahotas - 二维拉普拉斯滤波器
- Mahotas - 多数滤波器
- Mahotas - 均值滤波器
- Mahotas - 中值滤波器
- Mahotas - Otsu方法
- Mahotas - 高斯滤波
- Mahotas - Hit & Miss变换
- Mahotas - 标记最大值数组
- Mahotas - 图像平均值
- Mahotas - SURF密集点
- Mahotas - SURF积分图
- Mahotas - Haar变换
- 突出显示图像最大值
- 计算线性二值模式
- 获取标签边界
- 反转Haar变换
- Riddler-Calvard方法
- 标记区域的大小
- Mahotas - 模板匹配
- 加速鲁棒特征
- 去除边界标记
- Mahotas - Daubechies小波
- Mahotas - Sobel边缘检测
Mahotas - 图像区域最大值
区域最大值指的是图像中像素强度值最高的点。在一幅图像中,构成区域最大值的区域是所有其他区域中最亮的。区域最大值也称为全局最大值。
区域最大值考虑整幅图像,而局部最大值只考虑局部邻域,以查找强度值最高的像素。
区域最大值是局部最大值的一个子集,因此所有区域最大值都是局部最大值,但并非所有局部最大值都是区域最大值。
一幅图像可以包含多个区域最大值,但所有区域最大值的强度都相同。这是因为区域最大值只考虑最高的强度值。
Mahotas中的图像区域最大值
在Mahotas中,我们可以使用mahotas.regmax()函数查找图像中的区域最大值。区域最大值通过图像中的强度峰值来识别,因为它们代表高强度区域。
区域最大值点突出显示为白色,而其他点则为黑色。
mahotas.regmax()函数
mahotas.regmax()函数从输入的灰度图像中提取区域最大值。
它输出一个图像,其中1代表区域最大值点的存在,0代表普通点。
regmax()函数使用基于形态学重建的方法来查找区域最大值。在这种方法中,每个局部最大值区域的强度值与其邻居进行比较。
如果发现邻居具有更高的强度,则它成为新的区域最大值。此过程持续进行,直到没有更高强度区域剩余,表明已达到区域最大值。
语法
以下是Mahotas中regmax()函数的基本语法:
mahotas.regmax(f, Bc={3x3 cross}, out={np.empty(f.shape, bool)})
其中,
f - 输入灰度图像。
Bc (可选) - 用于连接的结构元素。
out (可选) - 布尔数据类型的输出数组(默认为与f大小相同的新的数组)。
示例
在下面的示例中,我们使用mh.regmax()函数获取图像的区域最大值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('tree.tiff') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Getting the regional maxima regional_maxima = mh.regmax(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the regional maxima axes[1].imshow(regional_maxima, cmap='gray') axes[1].set_title('Regional Maxima') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
以下是上述代码的输出:
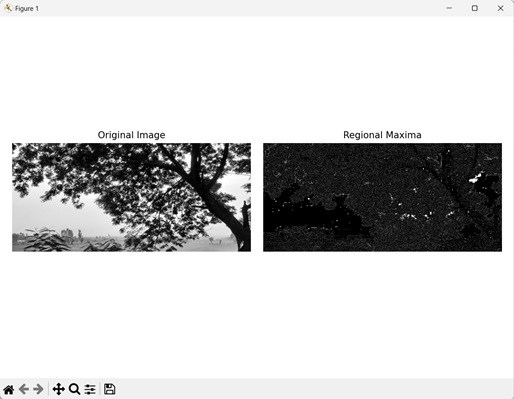
使用自定义结构元素
我们还可以使用自定义结构元素来从图像中获取区域最大值。结构元素是一个奇数维度的二元数组,由1和0组成,它定义了图像标记期间邻域像素的连接模式。
1表示包含在连接性分析中的相邻像素,而0表示被排除或忽略的邻居。
在Mahotas中,提取区域最大值区域时,我们可以使用自定义结构元素来定义相邻像素的连接性。我们首先使用numpy.array()函数创建一个奇数维度的结构元素。
然后,我们将此自定义结构元素输入到regmax()函数的Bc参数中。
例如,让我们考虑自定义结构元素:[[0, 0, 0, 0, 1], [0, 0, 1, 0, 0], [1, 0, 0, 0, 0], [0, 0, 0, 1, 0], [0, 1, 0, 0, 0]]。 此结构元素暗示水平连接,即,只有水平位于另一个像素左侧或右侧的像素才被视为其邻居。
示例
在这个例子中,我们使用自定义结构元素来获取图像的区域最大值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('sun.png') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Setting custom structuring element struct_element = np.array([[0, 0, 0, 0, 1], [0, 0, 1, 0, 0], [1, 0, 0, 0, 0], [0, 0, 0, 1, 0], [0, 1, 0, 0, 0]]) # Getting the regional maxima regional_maxima = mh.regmax(image, Bc=struct_element) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the regional maxima axes[1].imshow(regional_maxima, cmap='gray') axes[1].set_title('Regional Maxima') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
上述代码的输出如下:
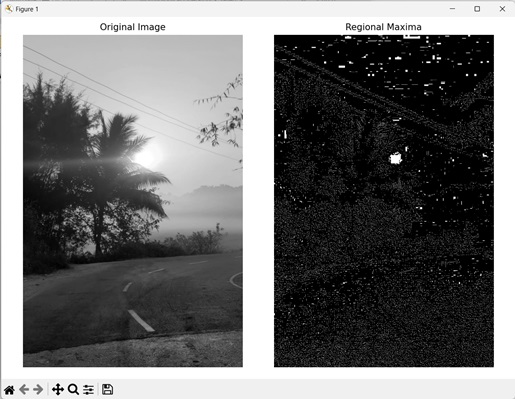
使用图像的特定区域
我们还可以找到图像特定区域的区域最大值。图像的特定区域是指较大图像的一小部分。可以通过裁剪原始图像来去除不需要的区域,从而提取特定区域。
在Mahotas中,我们可以在图像的一部分中找到区域最大值。首先,我们通过指定x轴和y轴所需的尺寸来裁剪原始图像。然后我们使用裁剪后的图像并使用regmax()函数获取区域最大值。
例如,假设我们将[:800, 70:]指定为x轴和y轴的尺寸。然后,裁剪后的图像的x轴范围为0到800像素,y轴范围为70到最大尺寸。
示例
在这个例子中,我们正在获取图像特定区域内的区域最大值。
import mahotas as mh import numpy as np import matplotlib.pyplot as mtplt # Loading the image image = mh.imread('nature.jpeg') # Converting it to grayscale image = mh.colors.rgb2gray(image) # Using specific region of the image image = image[:800, 70:] # Getting the regional maxima regional_maxima = mh.regmax(image) # Creating a figure and axes for subplots fig, axes = mtplt.subplots(1, 2) # Displaying the original image axes[0].imshow(image, cmap='gray') axes[0].set_title('Original Image') axes[0].set_axis_off() # Displaying the regional maxima axes[1].imshow(regional_maxima, cmap='gray') axes[1].set_title('Regional Maxima') axes[1].set_axis_off() # Adjusting spacing between subplots mtplt.tight_layout() # Showing the figures mtplt.show()
输出
执行上述代码后,我们得到以下输出:
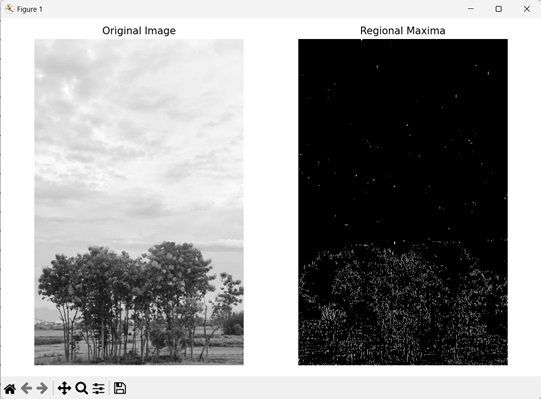