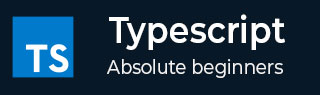
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbols
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 抽象类
抽象类
抽象类用于在 TypeScript 中实现抽象。抽象类只包含方法声明,而不包含实现。我们需要在继承类中实现抽象类所有抽象方法。
抽象是一种向用户和一些开发者隐藏底层代码实现的方式。此外,它用于仅显示方法的必要信息,而不是显示方法的整个复杂实现。
创建抽象类
我们可以使用abstract关键字定义抽象类或方法。抽象类可以包含普通方法和抽象方法。在抽象类中,我们需要实现函数或普通方法,并且只需要声明抽象方法。
我们可以使用任何其他类继承抽象类,但是我们需要在继承类中实现抽象类中的所有抽象方法。如果我们不想在继承类中实现抽象方法,我们需要使用 abstract 关键字将继承类也设置为抽象类。
此外,我们不能创建抽象类的对象,但是可以创建继承类的对象并使用抽象类方法。抽象类的限制是,我们不能使用多个抽象类实现多重继承。
语法
您可以遵循以下语法来创建和继承抽象类到其他类。
abstract class sample { // define variables inside the abstract class, // declare the abstract methods or non-abstract method inside the abstract class abstract demo(string): void; } // extend sample class and implement all abstract methods of sample to demo class class test extends sample { demo(name: string): void { // code for the demo method } }
示例 1
在下面的示例中,我们定义了一个包含抽象方法的抽象类。在继承的测试类中,我们实现了示例类的抽象方法。接下来,我们创建了一个带有 3 个参数的测试类对象,并调用了 demo() 和 save() 方法。
abstract class sample { // define variables inside the abstract class, property1: string; constructor(property1: string, property2: number) { this.property1 = property1; } // declare the abstract methods abstract demo(): void; // defining the non-abstract methods save(): void { console.log("The save method of the abstract class is executed."); } } // extend sample class and implement all abstract methods of sample to demo class class test extends sample { property2: number; constructor(property1: string, property2: number) { super(property1); this.property2 = property2; } demo(): void { // code for the demo method console.log("The value of the property 3 is " + this.propert2); } } let test_obj = new test("TutorialsPont", 9999); test_obj.demo(); test_obj.save();
在上面的示例中,我们从继承类测试中隐藏了 save() 方法的实现。我们允许开发者根据需要实现 demo() 方法,但隐藏了其他类信息,例如 property1、property2 和 save() 方法的实现。
现在,用户可以正确理解使用抽象类的目的,以及如何使用它来隐藏信息并仅显示所需信息。
编译后,以上代码将生成以下 JavaScript 代码:
var __extends = (this && this.__extends) || (function () { var extendStatics = function (d, b) { extendStatics = Object.setPrototypeOf || ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; return extendStatics(d, b); }; return function (d, b) { extendStatics(d, b); function __() { this.constructor = d; } d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); }; })(); var sample = /** @class */ (function () { function sample(property1, property2) { this.property1 = property1; } // defining the non-abstract methods sample.prototype.save = function () { console.log("The save method of the abstract class is executed."); }; return sample; }()); // extend sample class and implement all abstract methods of sample to demo class var test = /** @class */ (function (_super) { __extends(test, _super); function test(property1, property2) { var _this = _super.call(this, property1) || this; _this.property2 = property2; return _this; } test.prototype.demo = function () { // code for the demo method console.log("The value of the property 3 is " + this.propert2); }; return test; }(sample)); var test_obj = new test("TutorialsPont", 9999); test_obj.demo(); test_obj.save();
输出
它将产生以下输出:
The value of the property 3 is undefined The save method of the abstract class is executed.
示例 2
在下面的示例中,class1 是抽象类,它包含抽象方法 method1 的声明。class2 只包含 method2() 的定义。它扩展了 class1 但没有实现名为 method1() 的抽象方法。
之后,我们定义了 class3 并通过 class2 继承它。我们还在 class3 中定义了 class 的 method1。最后,我们创建了 class3 的对象并调用了 method1() 和 method2()。
// define the abstract class1 containing the abstract method1 abstract class class1 { abstract method1(): void; } // Need to create class2 to abstract as we inherited class1 but doesn't defined abstract method1() abstract class class2 extends class1 { method2(): void { console.log("Inside the method 2 of class2."); } } // defining the class3 inherited by the class2 class class3 extends class2 { // Implementation of the method1 of the abstract class1 method1(): void { console.log( "Implemented the abstract method name method1 of class1 inside the class3" ); } } // Crating the object of the class3 var object = new class3(); // Invoking the method1 of class1 which is declared in the abstract class1 object.method1(); // Invoking the method2 of class2 object.method2();
上面的例子向我们展示了,如果我们通过任何类继承抽象类,并且不想在继承类中实现抽象方法,我们需要将继承类设为抽象类。
编译后,以上代码将生成以下 JavaScript 代码:
var __extends = (this && this.__extends) || (function () { var extendStatics = function (d, b) { extendStatics = Object.setPrototypeOf || ({ __proto__: [] } instanceof Array && function (d, b) { d.__proto__ = b; }) || function (d, b) { for (var p in b) if (b.hasOwnProperty(p)) d[p] = b[p]; }; return extendStatics(d, b); }; return function (d, b) { extendStatics(d, b); function __() { this.constructor = d; } d.prototype = b === null ? Object.create(b) : (__.prototype = b.prototype, new __()); }; })(); // define the abstract class1 containing the abstract method1 var class1 = /** @class */ (function () { function class1() { } return class1; }()); // Need to create class2 to abstract as we inherited class1 but doesn't defined abstract method1() var class2 = /** @class */ (function (_super) { __extends(class2, _super); function class2() { return _super !== null && _super.apply(this, arguments) || this; } class2.prototype.method2 = function () { console.log("Inside the method 2 of class2."); }; return class2; }(class1)); // defining the class3 inherited by the class2 var class3 = /** @class */ (function (_super) { __extends(class3, _super); function class3() { return _super !== null && _super.apply(this, arguments) || this; } // Implementation of the method1 of the abstract class1 class3.prototype.method1 = function () { console.log("Implemented the abstract method name method1 of class1 inside the class3"); }; return class3; }(class2)); // Crating the object of the class3 var object = new class3(); // Invoking the method1 of class1 which is declared in the abstract class1 object.method1(); // Invoking the method2 of class2 object.method2();
输出
它将产生以下输出:
Implemented the abstract method name method1 of class1 inside the class3 Inside the method 2 of class2.