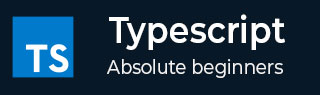
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策语句
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型保护
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用工具类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - typeof 类型运算符
在 TypeScript 中,typeof 是一个非常有用的运算符和关键字,用于检查变量或标识符的类型。然而,TypeScript 是一种类型严格的语言。因此,我们在定义标识符本身时需要定义变量或对象的类型。尽管如此,有时我们仍然需要检查变量的类型。
例如,我们通过表单从用户那里获取数据,并通过 TypeScript 处理它。然后,我们必须验证数据并执行一些数据库操作。此外,标识符在 TypeScript 中可以具有多种类型。因此,使用 typeof 运算符,我们可以初始化标识符后检查其类型。
语法
下面的语法演示了 typeof 运算符与标识符一起使用的示例。
let type = typeof <variable>
其中,
variable − 是需要检查类型的标识符。
返回值
它根据 typeof 运算符操作数的数据类型返回一个字符串。
示例
让我们通过 TypeScript 中的示例来了解 typeof 运算符 −
示例 1
在这个示例中,我们使用了 typeof 运算符与字符串、数字、布尔值和未定义变量一起使用。此外,我们还使用 typeof 运算符与值为 null 的变量一起使用。
在输出中,我们可以看到它返回表示标识符类型的字符串。对于 null_value1 标识符,typeof 运算符返回 object。
// Defining the variables of particular type and checking its type. let str1: string = "TutorialsPoint"; console.log(typeof str1); let num1: number = 32; console.log(typeof num1); let bool1: boolean = true; console.log(typeof bool1); let un_defined; console.log(typeof un_defined); let null_value1 = null; console.log(typeof null_value1);
编译后,它将生成以下 JavaScript 代码 −
// Defining the variables of particular type and checking its type. var str1 = "TutorialsPoint"; console.log(typeof str1); var num1 = 32; console.log(typeof num1); var bool1 = true; console.log(typeof bool1); var un_defined; console.log(typeof un_defined); var null_value1 = null; console.log(typeof null_value1);
输出
以上代码将产生以下输出 −
string number boolean undefined object
示例 2
在下面的示例中,我们创建了一个包含一些键值对的对象,一个返回数字值的函数,以及一个包含不同值的数字数组。
此外,我们还使用了 typeof 运算符来检查所有类型的类型。typeof 运算符对于对象返回字符串 "object",对于函数返回字符串 "function",对于数组返回字符串 "object",因为数组是对象的实例。
// using the typeof operator with object, function, and array. let demo_obj = { prop: "value", }; console.log(typeof demo_obj); function func() { let a = 10 + 20; return a; } console.log(typeof func); let array: Array<number> = [4, 23, 212, 2123, 323, 3]; console.log(typeof array);
编译后,它将生成以下 JavaScript 代码 −
// using the typeof operator with object, function, and array. var demo_obj = { prop: "value" }; console.log(typeof demo_obj); function func() { var a = 10 + 20; return a; } console.log(typeof func); var array = [4, 23, 212, 2123, 323, 3]; console.log(typeof array);
输出
以上代码将产生以下输出 −
object function object
示例 3
在这个示例中,我们使用 typeof 运算符检查了 NaN 关键字的类型,它返回字符串 "number"。负无穷大的类型也是数字。PI 是 Math 类的属性,它包含数字值。这就是为什么 typeof 运算符对于 Math.PI 属性返回 "number"。
pow() 是 Math 库函数,用于获取任何数字的幂。因此,typeof 运算符对于 Math.pow() 方法返回 "function"。
// using the typeof operator with NaN, infinity, library methods, and members. let NaN_type = NaN; console.log(typeof NaN_type); let infi = -Infinity; console.log(typeof infi); let pI = Math.PI; console.log(typeof pI); let pow = Math.pow; console.log(typeof pow);
编译后,它将生成以下 JavaScript 代码 −
// using the typeof operator with NaN, infinity, library methods, and members. var NaN_type = NaN; console.log(typeof NaN_type); var infi = -Infinity; console.log(typeof infi); var pI = Math.PI; console.log(typeof pI); var pow = Math.pow; console.log(typeof pow);
输出
以上代码将产生以下输出 −
number number number function
示例 4
在这个示例中,我们使用 typeof 运算符获取 variable1 的类型,并定义与 variable1 类型相同的 variable2。此外,我们还使用类型别名来存储 variable3 的类型。名为 type1 的类型包含 variable3 的类型,它是一个字符串。
之后,我们使用 type1 来定义 variable4 的类型。在输出中,用户可以观察到 variable4 的类型与 variable3 相同。
// defining the variable1 of type number var variable1: number = 10; // To define the variable2 as of same type variable1 let variable2: typeof variable1 = 20; console.log(typeof variable2); // variable3 of string type var variable3: string = "Hello!"; // using the type alias to define the type of same type as variable3 type type1 = typeof variable3; // defining the variable4 of type named type1 let variable4: type1 = "Hi"; console.log(typeof variable4);
编译后,它将生成以下 JavaScript 代码 −
// defining the variable1 of type number var variable1 = 10; // To define the variable2 as of same type variable1 var variable2 = 20; console.log(typeof variable2); // variable3 of string type var variable3 = "Hello!"; // defining the variable4 of type named type1 var variable4 = "Hi"; console.log(typeof variable4);
输出
以上代码将产生以下输出 −
number string