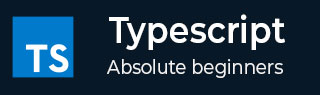
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript 与 JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null 与 undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 嵌套 if 语句
TypeScript 中的嵌套 if 语句是指存在于另一个 if 或 else 语句体内的 if 语句。else...if 阶梯式结构是一种嵌套 if 语句。嵌套 if 语句或 else...if 阶梯式结构可用于测试多个条件。其语法如下所示:
语法
if (boolean_expression1) { //statements if the expression1 evaluates to true } else if (boolean_expression2) { //statements if the expression2 evaluates to true } else if (boolean_expression3) { //statements if the expression3 evaluates to false } else { //statements if all three boolean expressions result to false }
在使用if...else...if 和else 语句时,需要注意以下几点。
一个if 可以有零个或一个else,并且它必须位于任何else...if 之后。
一个if 可以有零个到多个else...if,并且它们必须位于else 之前。
一旦else...if 成功,就不会再测试任何剩余的else...if 或else。
示例:else…if 阶梯式结构
var num:number = 2 if(num > 0) { console.log(num+" is positive") } else if(num < 0) { console.log(num+" is negative") } else { console.log(num+" is neither positive nor negative") }
此代码片段显示值是正数、负数还是零。
编译后,将生成以下 JavaScript 代码:
//Generated by typescript 1.8.10 var num = 2; if (num > 0) { console.log(num + " is positive"); } else if (num < 0) { console.log(num + " is negative"); } else { console.log(num + " is neither positive nor negative"); }
以下是上述代码的输出:
2 is positive
广告