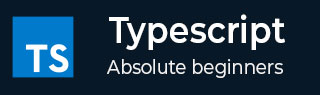
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - 其余参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 杂项
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 其余参数
其余参数
在 TypeScript 中,其余参数允许函数接受可变数量的参数并将它们存储为数组。当您希望定义一个可以处理可变数量参数的函数时,这非常有用。
其余参数允许您将剩余的参数收集到一个数组中。其余参数的名称成为保存此数组的变量。
其余参数语法
其余参数使用省略号/三个点 (...) 后跟函数声明中的参数名称来编写。我们使用数组类型作为其余参数的类型注解。
function funcName(...rest: type[]): returnType{ // function body; }
其中,
funcName - 它是我们函数的名称。
...rest - 它将多个参数存储到名为 rest 的数组中。
type[] - 它指定参数的类型
一个函数可以有任意数量的普通参数以及其余参数。
其余参数必须位于参数列表的最后。
function funcName (...rest1: type[], param1: type){} //Error : A rest parameter must be last in a parameter list.
函数定义中只能有一个其余参数。
function funcName (...rest1: type[], ...rest2: number[]){} //Error: A rest parameter must be last in a parameter list.
与上述函数声明相同,函数表达式也可以有其余参数。
let getSum = function(...rest: type[]){ function body; }
示例
让我们通过 TypeScript 中的一些示例来了解其余参数。
示例:可变长度参数列表
使用其余参数,我们可以使用不同的参数数量来调用函数。其余参数可以处理这些不同的参数数量。
在下面的示例中,我们定义了一个名为 sum 的函数,它带有一个其余参数 ...nums。参数存储为数组 nums 的元素。每次我们使用不同数量的参数调用函数时,nums 都会存储这些参数。并且我们可以在 nums 上执行任何数组操作。
function sum(...nums: number[]) { let totalSum = 0; for (let num of nums) { totalSum += num; } return totalSum; } console.log(sum(10, 20, 30, 40)); console.log(sum(10, 20)); console.log(sum());
编译后,它将生成以下 JavaScript 代码。
function sum(...nums) { let totalSum = 0; for (let num of nums) { totalSum += num; } return totalSum; } console.log(sum(10, 20, 30, 40)); console.log(sum(10, 20)); console.log(sum());
上述示例代码的输出如下:
100 30 0
示例:访问参数长度
在此示例中,我们定义了一个名为 getLen 的函数,它带有一个其余参数 ...theArgs。我们使用数组的 length 属性来获取参数的长度或数量。
function getLen(...theArgs:number[]) { console.log(theArgs.length); } getLen(); getLen(5); getLen(5, 6, 7);
编译后,它将生成以下 JavaScript 代码。
function getLen(...theArgs) { console.log(theArgs.length); } getLen(); getLen(5); getLen(5, 6, 7);
上述示例代码的输出如下:
0 1 3
其余参数 & 展开运算符
我们已经讨论了其余参数。展开运算符用三个点 (...) 表示,与其余参数相同,但工作方式不同。
其余参数用于将剩余的参数收集为一个数组。展开运算符将数组的元素扩展为单个元素。
展开参数必须具有元组类型或传递给其余参数。
示例:数组作为展开参数
在此示例中,我们定义了两个数组 arr1 和 arr2,每个数组包含三个元素。我们对 arr1 调用 push() 方法,并将 ...arr2 作为参数传递。这作为展开参数工作,因为它将 arr2 的元素扩展为单个元素。
const arr1: number[] = [10, 20, 30]; const arr2: number[] = [40, 50, 60]; arr1.push(...arr2); console.log(arr1);
编译后,它将生成以下 JavaScript 代码。
const arr1 = [10, 20, 30]; const arr2 = [40, 50, 60]; arr1.push(...arr2); console.log(arr1);
上述代码的输出如下:
[10, 20, 30, 40, 50, 60]
示例:查找最大/最小数字
在下面的示例中,我们找到最大数字。我们定义了一个名为 getMax 的函数,它带有一个参数,...args: number[]。我们调用 Math.max() 方法,并传递参数 ...args。参数 ...args 中的三个点充当展开运算符。它扩展/解包数组 args 的元素。
function getMax(...args:number[]){ // here ...args as rest parameter return Math.max(...args); // here ... works as spread operator } console.log(getMax(10,20,30,40)); console.log(getMax(10,20,30));
编译后,它将生成以下 JavaScript 代码。
function getMax(...args) { return Math.max(...args); // here ... works as spread operator } console.log(getMax(10, 20, 30, 40)); console.log(getMax(10, 20, 30));
上述示例代码的输出如下:
40 30
示例:传递其余参数
其余参数将参数解包为单个元素。
在此示例中,我们定义了一个名为 multiply 的函数,它接受三个数字类型的参数并返回它们的乘积。函数的返回类型也是数字。我们调用该函数,并传递一个其余参数 (...numbers)。
function multiply(a: number, b: number, c: number): number { return a * b * c; } let numbers: [number, number, number]; numbers = [2, 3, 4]; console.log(multiply(...numbers));
编译后,它将生成以下 JavaScript 代码。
function multiply(a, b, c) { return a * b * c; } let numbers; numbers = [2, 3, 4]; console.log(multiply(...numbers));
上述示例代码的输出如下:
24