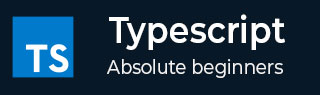
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 联合类型
TypeScript 使程序能够组合一个或两个类型。联合类型是表达可以是多种类型之一的值的强大方法。使用管道符号 (|) 组合两个或多个数据类型来表示联合类型。换句话说,联合类型写成用竖线分隔的类型序列。
语法:联合字面量
我们在 TypeScript 中使用管道符号 (|) 来组合两种或多种类型以实现联合类型 -
Type1 | Type2 | Type3
联合类型变量
我们可以定义一个不同类型联合类型的变量。例如,
let value: number | string | boolean;
在上面的示例中,我们定义了一个名为 value 的联合类型变量。我们可以为该变量分配数字、字符串或布尔值。
示例:联合类型变量
在下面的示例中,变量的类型是联合类型。这意味着变量可以包含数字或字符串作为其值。
var val: string | number val = 12 console.log("numeric value of val: "+val) val = "This is a string" console.log("string value of val: "+val)
编译后,它将生成以下 JavaScript 代码。
var val; val = 12; console.log("numeric value of val: " + val); val = "This is a string"; console.log("string value of val: " + val);
上述示例代码的输出如下 -
numeric value of val: 12 string value of val: this is a string
联合类型和函数参数
我们可以定义一个具有联合类型参数的函数。当您可以调用该函数时,您可以传递联合类型中任何类型的参数。例如,
function display(name: string | string[]){ // function body; }
在上面的代码片段中,函数 display() 具有联合类型(字符串或字符串数组)的参数。您可以调用该函数,传递字符串或字符串数组作为参数。
示例
在下面的示例中,我们定义了一个名为 disp 的函数,它具有联合类型参数。
函数 disp() 可以接受字符串或字符串数组类型的参数。
function disp(name: string|string[]) { if(typeof name == "string") { console.log(name) } else { var i; for(i = 0;i<name.length;i++) { console.log(name[i]) } } } disp("mark") console.log("Printing names array....") disp(["Mark","Tom","Mary","John"])
编译后,它将生成以下 JavaScript 代码。
function disp(name) { if (typeof name == "string") { console.log(name); } else { var i; for (i = 0; i < name.length; i++) { console.log(name[i]); } } } disp("mark"); console.log("Printing names array...."); disp(["Mark", "Tom", "Mary", "John"]);
输出如下 -
Mark Printing names array…. Mark Tom Mary John
联合类型和数组
联合类型也可以应用于数组、属性和接口。以下说明了联合类型与数组的用法。
示例
程序声明一个数组。该数组可以表示数字集合或字符串集合。
var arr: number[]|string[]; var i: number; arr = [1,2,4] console.log("**numeric array**") for(i = 0;i<arr.length;i++) { console.log(arr[i]) } arr = ["Mumbai","Pune","Delhi"] console.log("**string array**") for(i = 0;i<arr.length;i++) { console.log(arr[i]) }
编译后,它将生成以下 JavaScript 代码。
var arr; var i; arr = [1, 2, 4]; console.log("**numeric array**"); for (i = 0; i < arr.length; i++) { console.log(arr[i]); } arr = ["Mumbai", "Pune", "Delhi"]; console.log("**string array**"); for (i = 0; i < arr.length; i++) { console.log(arr[i]); }
输出如下 -
**numeric array** 1 2 4 **string array** Mumbai Pune Delhi
广告