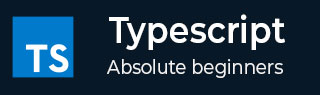
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - Function 构造函数
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 条件类型
在 TypeScript 中,条件类型允许您根据条件为变量赋值类型。这使您可以定义根据特定条件动态更改的类型。此功能对于大型应用程序非常有用,在大型应用程序中,您需要根据不同的情况进行动态类型设置。
基本条件类型
我们将使用三元运算符 (?:) 来使用条件类型。它将评估条件,并根据真或假的结果选择新类型。
语法
您可以按照以下语法使用条件类型。
type A = Type extends anotherType ? TrueType : FalseType;
在上述语法中,'Type extends anotherType' 条件将首先被评估。
如果条件为真,则 'type A' 将包含 'TrueType'。否则,它将包含 'FalseType'。
这里,'extends' 关键字检查 'Type' 是否与 'anotherType' 相同,或者至少包含 'anotherType' 类型的全部属性。
示例
在下面的代码中,我们定义了包含 name、model 和 year 属性的 'car' 类型。我们还定义了只包含 'name' 属性的 'Name' 类型。
'carNameType' 类型变量根据 'Car extends Name' 条件的评估结果存储字符串或任何值。这里,条件将被评估为真,因为 'Car' 类型包含 'Name' 类型的所有属性。
之后,我们创建了 'carNameType' 类型的 'carName' 变量并在控制台中打印它。
type Car = { name: string, model: string, year: number, } type Name = { name: string } // If Car extends Name, then carNameType is string, otherwise it is any type carNameType = Car extends Name ? string : any; // string // Define a variable carName of type carNameType const carName: carNameType = 'Ford'; console.log(carName); // Ford
编译后,它将生成以下 JavaScript 代码。
// Define a variable carName of type carNameType const carName = 'Ford'; console.log(carName); // Ford
输出
Ford
泛型条件类型
现在,让我们学习泛型条件类型。在 TypeScript 中,泛型类型类似于函数中的参数。它允许开发人员定义条件类型,以便可以在代码中的多个位置使用它。它提供了在条件语句中使用不同类型的灵活性。
语法
您可以按照以下语法使用泛型条件类型。
type ConditionalType<T> = T extends Type1 ? TrueType : FalseType;
在上述语法中,'conditionalType<T>' 有一个类型 'T' 参数,'conditionalType' 是类型的名称。
条件 'T extends Type1' 检查类型 'T' 是否扩展 'Type1'。
如果条件评估为真,则 'TrueType' 将被分配给 'ConditionalType'。否则,将分配 'FalseType'。
示例
在下面的代码中,'IsNumArray<T>' 是一个泛型类型,它将类型 'T' 作为参数。它检查 'T' 的类型是否为数字数组 (number[])。如果是,则返回 'number'。否则,返回 'string'。
之后,我们定义了 'num' 和 'str' 变量并使用它们与 'IsNumArray' 类型一起使用。对于 'num' 变量,我们使用了 'number[]' 参数;对于 'str' 变量,我们使用了 'string[]' 参数。
// Generic conditional types type IsNumArray<T> = T extends number[] ? number : string; const num: IsNumArray<number[]> = 5; // number const str: IsNumArray<string[]> = '5'; // string console.log(num); console.log(str);
编译后,它将生成以下 JavaScript 代码。
const num = 5; // number const str = '5'; // string console.log(num); console.log(str);
输出
5 5
条件类型约束
条件类型约束也称为类型断言或条件类型谓词。它用于为泛型类型添加约束。泛型类型是可重用的,但是如果您想将它们重用于特定数据类型(如数组、数字等),则应使用泛型类型添加约束。
语法
您可以按照以下语法使用条件类型约束。
type ConditionalType<T extends T1 | T2> = T extends Type1 ? TrueType : FalseType;
在上述语法中,我们为类型参数 'T' 添加了约束。它接受类型 'T1' 和 'T2' 的值。
示例
在下面的代码中,'CondionalType' 是一个泛型类型。它将数字或字符串类型作为类型参数 'T' 的值。条件类型如果 'T' 的类型是数字,则返回数字。否则,返回字符串。
接下来,我们通过传递数字和字符串作为类型参数来重用 ConditionalType 类型。
您可以注意到,当我们尝试使用 'boolean' 作为类型参数时,它会抛出错误。因此,它允许使用有限类型的此泛型条件类型。
// Defining the conditional type with constraints type ConditionalType<T extends number | string> = T extends number ? number : string; let x: ConditionalType<number> = 10; let y: ConditionalType<string> = 'Hello'; // let z: ConditionalType<boolean> = true; // Error: Type 'boolean' does not satisfy the constraint 'number | string'. console.log("The value of x is: ", x); console.log("The value of y is: ", y);
编译后,它将生成以下 JavaScript 代码。
let x = 10; let y = 'Hello'; // let z: ConditionalType<boolean> = true; // Error: Type 'boolean' does not satisfy the constraint 'number | string'. console.log("The value of x is: ", x); console.log("The value of y is: ", y);
输出
The value of x is: 10 The value of y is: Hello
在条件类型中推断
在 TypeScript 中,在条件类型中推断意味着使用条件类型定义来推断类型。它允许您创建更灵活的类型转换。
例如,如果您有一个元素数组并将其与条件类型中的类型 'T' 匹配。之后,如果条件为真,您想返回数组元素的类型,在这种情况下,推断条件类型很有用。
语法
您可以使用 'infer' 关键字在条件类型中进行推断。
type Flatten<Type> = Type extends Array<infer Item> ? Item : Type;
在上述语法中,'infer' 关键字用于提取数组元素的类型。
示例
在下面的代码中,我们创建了 'Flatten' 条件类型。我们使用类型 'U' 使用 infer 关键字。它从具有类型 'U' 的元素数组中提取 'U' 的类型。
对于 'Flatten<boolean>',它将返回一个布尔值。因此,如果我们尝试将字符串值赋值给 'bool1' 变量,它将抛出错误。
// Inferring within the conditional types type Flatten<T> = T extends (infer U)[] ? U : T; let bool: Flatten<boolean[]> = true; // let bool1: Flatten<boolean> = "s"; // Error as string can't be assigned to boolean console.log(bool);
编译后,它将生成以下 JavaScript 代码。
let bool = true; // let bool1: Flatten<boolean> = "s"; // Error as string can't be assigned to boolean console.log(bool);
输出
true
这样,您就可以在 TypeScript 中使用条件类型。您可以使用泛型类型并推断它们以使类型转换更灵活。