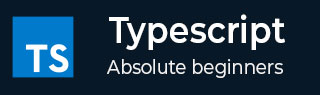
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 字符串
在 TypeScript 中,字符串表示一系列字符。字符串类型是 TypeScript 中的基本数据类型。字符串用于保存可以以文本形式表示的数据。与 JavaScript 一样,TypeScript 也支持字符串原生类型和 String 对象。
String 对象允许您处理一系列字符。它用许多辅助方法包装字符串原生数据类型。
您可以调用原生字符串上的方法,因为 TypeScript 会自动包装字符串原生类型并在包装器对象上调用方法。属性也适用相同规则。
创建字符串
TypeScript 中的字符串可以作为来自字符串字面量的原生类型创建,也可以使用 String() 构造函数作为对象创建。
您可以使用以下语法在 TypeScript 中创建一个String 对象:
cost str = new String(value);
这里,str 是新创建的 String 对象,value 是一系列字符。
您可以使用单引号、双引号和反引号创建字符串原生类型。
let str1: string = 'a string primitive'; let str2: string = "another string"; let str3: string = `yet another string`;
字符串原生类型也可以使用不带 new 关键字的 String() 函数创建。
let str: string = String(value);
‘string’ 是原生类型,但 ‘String’ 是包装器对象。尽可能优先使用 ‘string’。
字符串属性
以下是 String 对象中可用方法及其说明的列表:
序号 | 属性 & 说明 |
---|---|
1. | constructor
返回创建对象的 String 函数的引用。 |
2. | length
返回字符串的长度。 |
3. | prototype
prototype 属性允许您向对象添加属性和方法。 |
字符串方法
以下是 String 对象中可用方法及其说明的列表:
序号 | 方法 & 说明 |
---|---|
1. | charAt()
返回指定索引处的字符。 |
2. | charCodeAt()
返回一个数字,指示给定索引处字符的 Unicode 值。 |
3. | concat()
组合两个字符串的文本并返回一个新字符串。 |
4. | indexOf()
返回调用 String 对象中指定值第一次出现的索引,如果未找到则返回 -1。 |
5. | lastIndexOf()
返回调用 String 对象中指定值最后一次出现的索引,如果未找到则返回 -1。 |
6. | localeCompare()
返回一个数字,指示引用字符串在排序顺序中是在给定字符串之前、之后还是与之相同。 |
7. | match() 用于将正则表达式与字符串匹配。 |
8. | replace()
用于查找正则表达式和字符串之间的匹配项,并将匹配的子字符串替换为新的子字符串。 |
9. | search()
执行在正则表达式和指定字符串之间查找匹配项的操作。 |
10. | slice()
提取字符串的一部分并返回一个新字符串。 |
11. | split()
通过将字符串分割成子字符串,将 String 对象分割成字符串数组。 |
12. | substr()
返回字符串中从指定位置开始的指定数量的字符。 |
13. | substring()
返回字符串中两个索引之间的字符。 |
14. | toLocaleLowerCase()
字符串中的字符转换为小写,同时尊重当前区域设置。 |
15. | toLocaleUpperCase()
字符串中的字符转换为大写,同时尊重当前区域设置。 |
16. | toLowerCase()
返回转换为小写的调用字符串值。 |
17. | toString()
返回表示指定对象的字符串。 |
18. | toUpperCase()
返回转换为大写的调用字符串值。 |
19. | valueOf()
返回指定对象的原生值。 |
示例
让我们借助 TypeScript 中的一些示例来了解字符串类型。
示例:创建字符串原生类型
在下面的示例中,我们分别使用单引号、双引号和反引号创建原生字符串 str1、str2 和 str3。
let str1: string = 'a string primitive'; console.log(str1); let str2: string = "another string"; console.log(str2); let str3: string = `yet another string`; console.log(str3);
编译后,它将生成以下 JavaScript 代码。
let str1 = 'a string primitive'; console.log(str1); let str2 = "another string"; console.log(str2); let str3 = `yet another string`; console.log(str3);
上述示例代码的输出如下:
a string primitive another string yet another string
示例:创建字符串对象
这里我们使用带有 new 关键字的 String() 构造函数创建一个 String 对象。
const email = new String('[email protected]'); console.log(email); console.log(typeof email);
编译后,它将生成以下 JavaScript 代码。
const email = new String('[email protected]'); console.log(email); console.log(typeof email);
上述示例代码的输出如下:
[email protected] object
示例:连接 TypeScript 字符串
要连接两个字符串,我们可以使用 + 运算符。在这里,我们连接两个字符串 str1 和 str2,并在控制台中显示结果。
let str1: string = "Hello "; let str2: string = "World!"; let str3: string = str1 + str2; console.log(str3);
编译后,它将生成以下 JavaScript 代码。
let str1 = "Hello "; let str2 = "World!"; let str3 = str1 + str2; console.log(str3);
上述示例代码的输出如下:
Hello World!
示例:使用索引访问字符串元素
在下面的示例中,我们访问字符串中第 1 个和第 6 个索引的字符。
let message: string = "Hello World!"; console.log("Character at index 1 is => " + message[1]); console.log("Character at index 6 is => " + message[6]);
编译后,它将生成以下 JavaScript 代码。
let message = "Hello World!"; console.log("Character at index 1 is => " + message[1]); console.log("Character at index 6 is => " + message[6]);
上述示例代码的输出如下:
Character at index 1 is => e Character at index 6 is => W
示例:TypeScript 中的 String 与 string
在 TypeScript 中,类型 'String' 是一个包装器对象,类型 'string' 是一个原生类型。这两种类型不能相互赋值。
在下面的示例中,我们尝试将一个字符串对象赋值给 string 原生类型的变量。
let str: string; str = new String('shahid');
上述示例代码将显示以下错误:
Type 'String' is not assignable to type 'string'. 'string' is a primitive, but 'String' is a wrapper object. Prefer using 'string' when possible.
始终建议使用字符串原生类型。