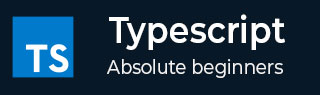
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 循环
您可能会遇到需要多次执行代码块的情况。通常,语句是按顺序执行的:函数中的第一个语句首先执行,然后是第二个语句,依此类推。
编程语言提供了各种控制结构,允许更复杂的执行路径。
循环语句允许我们多次执行语句或语句组。下面是在大多数编程语言中循环语句的一般形式。
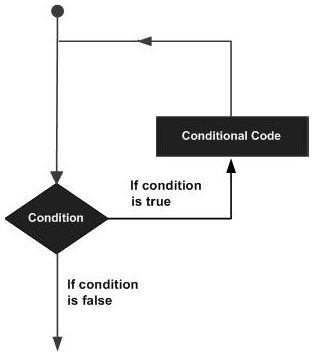
TypeScript 提供不同类型的循环来处理循环需求。下图说明了循环的分类 -
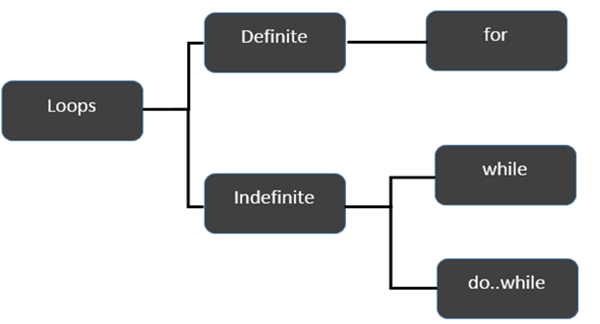
确定循环
迭代次数确定/固定的循环称为确定循环。for 循环是确定循环的一种实现。
序号 | 循环和描述 |
---|---|
1. | for 循环
for 循环是确定循环的一种实现。 |
不确定循环
当循环中的迭代次数不确定或未知时,使用不确定循环。
不确定循环可以使用 -
序号 | 循环和描述 |
---|---|
1. | while 循环
while 循环在每次指定的条件计算结果为真时执行指令。 |
2. | do… while
do…while 循环与 while 循环类似,只是 do...while 循环在第一次执行循环时不会计算条件。 |
示例:while 与 do..while
var n:number = 5 while(n > 5) { console.log("Entered while") } do { console.log("Entered do…while") } while(n>5)
此示例最初声明了一个 while 循环。只有当传递给 while 的表达式计算结果为真时,才会进入循环。在此示例中,n 的值不大于零,因此表达式返回 false,并且跳过循环。
另一方面,do…while 循环会执行一次语句。这是因为初始迭代不考虑布尔表达式。但是,对于后续迭代,while 会检查条件并将控制权移出循环。
编译后,它将生成以下 JavaScript 代码 -
var n = 5; while (n > 5) { console.log("Entered while"); } do { console.log("Entered do…while"); } while (n > 5);
以上代码将产生以下输出 -
Entered do…while
break 语句
break 语句用于将控制权移出构造。在循环中使用break 会导致程序退出循环。其语法如下 -
语法
break
流程图
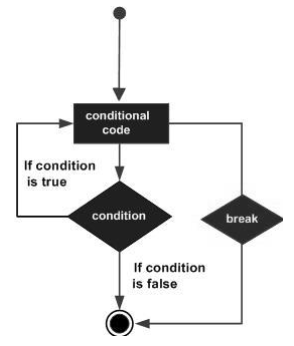
示例
现在,看看下面的示例代码 -
var i:number = 1 while(i<=10) { if (i % 5 == 0) { console.log ("The first multiple of 5 between 1 and 10 is : "+i) break //exit the loop if the first multiple is found } i++ } //outputs 5 and exits the loop
编译后,它将生成以下 JavaScript 代码 -
var i = 1; while (i <= 10) { if (i % 5 == 0) { console.log("The first multiple of 5 between 1 and 10 is : " + i); break; //exit the loop if the first multiple is found } i++; } //outputs 5 and exits the loop
它将产生以下输出 -
The first multiple of 5 between 1 and 10 is : 5
continue 语句
continue 语句跳过当前迭代中的后续语句,并将控制权返回到循环的开头。与 break 语句不同,continue 不会退出循环。它终止当前迭代并开始后续迭代。
语法
continue
流程图
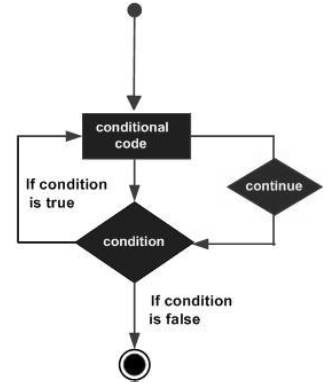
示例
continue 语句的示例如下 -
var num:number = 0 var count:number = 0; for(num=0;num<=20;num++) { if (num % 2==0) { continue } count++ } console.log (" The count of odd values between 0 and 20 is: "+count) //outputs 10
以上示例显示了 0 到 20 之间奇数值的数量。如果数字为偶数,则循环退出当前迭代。这是使用continue 语句实现的。
编译后,它将生成以下 JavaScript 代码。
var num = 0; var count = 0; for (num = 0; num <= 20; num++) { if (num % 2 == 0) { continue; } count++; } console.log(" The count of odd values between 0 and 20 is: " + count); //outputs 10
输出
The count of odd values between 0 and 20 is: 10
无限循环
无限循环是一个无限运行的循环。for 循环和while 循环可用于创建无限循环。
语法:使用 for 循环创建无限循环
for(;;) { //statements }
示例:使用 for 循环创建无限循环
for(;;) { console.log(“This is an endless loop”) }
语法:使用 while 循环创建无限循环
while(true) { //statements }
示例:使用 while 循环创建无限循环
while(true) { console.log(“This is an endless loop”) }