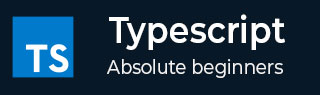
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 字面量类型
在 TypeScript 中,字面量类型是基本数据类型的子类型。字面量类型允许您指定变量可以包含的确切值。
TypeScript 中有三种类型的字面量类型。
字符串字面量类型
数字字面量类型
布尔字面量类型
它们都允许您在变量中存储特定值,而不是存储通用的字符串、数字或布尔值。
语法
您可以遵循以下语法在 TypeScript 中使用字面量类型。
type lit_type = type_1 | type_2 | type_3 | ...
在上述语法中,“type”是创建类型别名的关键字。“lit_type”是类型的名称。“type_1”、“type_2”和“type_3”是字符串、布尔值或数字类型的数值。
字符串字面量类型
字符串字面量类型允许您定义一组特定值,变量或函数参数应包含其中的任何值。
示例
在下面的代码中,我们创建了“Direction”类型,其中包含字符串格式的四个方向。move()函数参数的类型是 Direction,因此它接受四个方向中的任何值。
如果您尝试传递四个方向以外的任何值作为参数,它将抛出错误。
// Defining a custom-type Direction type Direction = "North" | "East" | "South" | "West"; // Defining a function move that takes a single argument of type Direction. function move(direction: Direction) { console.log(`Moving in the direction: ${direction}`); } move("North"); move("East"); // move("Northeast"); // Error: Argument of type '"Northeast"' is not assignable to parameter of type 'Direction'.
编译后,它将生成以下 JavaScript 代码。
// Defining a function move that takes a single argument of type Direction. function move(direction) { console.log(`Moving in the direction: ${direction}`); } move("North"); move("East"); // move("Northeast"); // Error: Argument of type '"Northeast"' is not assignable to parameter of type 'Direction'.
上述代码的输出如下:
Moving in the direction: North Moving in the direction: East
数字字面量类型
数字字面量类型类似于字符串字面量,但允许您指定确切的数值作为允许的类型。
示例
在下面的代码中,“SmallPrimes”类型包含到 11 的小素数作为值。“prime”变量的类型是“SmallPrimes”。因此,它只能包含 2、3、5、7 或 11 中的任何值。
type SmallPrimes = 2 | 3 | 5 | 7 | 11; let prime: SmallPrimes; prime = 7; console.log(prime); // 7 // prime = 4; // Error: Type '4' is not assignable to type 'SmallPrimes'.
编译后,它将生成以下 JavaScript 代码。
let prime; prime = 7; console.log(prime); // 7 // prime = 4; // Error: Type '4' is not assignable to type 'SmallPrimes'.
上述代码的输出如下:
7
组合字面量类型
您可以使用联合类型组合不同类型的字面量(字符串、数字、布尔值),以允许变量保存一组指定的值。
示例
在下面的代码中,“MixedLiterals”类型包含“click”、404 和 true 值。
action 变量可以包含“MixedLiterals”类型三个值中的任何一个值。
// Mixed type literals type MixedLiterals = "Click" | 404 | true; let action: MixedLiterals; action = "Click"; // Valid console.log(action); action = 404; // Valid console.log(action); action = true; // Valid console.log(action); // action = "Other"; // Error: Type '"Other"' is not assignable to type 'MixedLiterals'.
编译后,它将生成以下 JavaScript 代码。
let action; action = "Click"; // Valid console.log(action); action = 404; // Valid console.log(action); action = true; // Valid console.log(action); // action = "Other"; // Error: Type '"Other"' is not assignable to type 'MixedLiterals'.
上述代码的输出如下:
Click 404 true
字面量类型的用例
字面量类型有多个用例。但是,我们将研究字面量类型的一些实时用例。
配置 - 用于定义变量或函数参数的特定配置,这些变量或函数参数仅采用特定值。
状态管理 - 类型字面量可用于状态管理。
API 响应处理 - 用于根据 API 响应状态处理 API 响应。
TypeScript 中的字面量类型通过允许您指定完全可接受的值来增强应用程序的类型安全性。它还有助于开发人员维护代码复杂性并提高可读性。