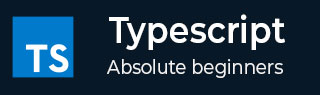
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 实用类型
TypeScript 允许我们从现有类型创建新类型,我们可以使用实用类型进行这种转换。
TypeScript 中存在各种实用类型,我们可以根据类型转换的需求使用任何实用类型。
让我们用 TypeScript 的例子来讨论不同的实用类型。
TypeScript 中的 Partial 类型
Partial 实用类型将当前类型的全部属性转换为可选属性。partial 的含义是全部、部分或无。因此,它使所有属性都可选,用户可以在使用对象重构代码时使用它。
示例
在下面的示例中,我们创建了包含一些可选属性的类型。之后,我们使用 Partial 实用类型创建了一个 partialType 对象。用户可以看到我们没有初始化 partialType 对象的所有属性,因为所有属性都是可选的。
type Type = { prop1: string; prop2: string; prop3: number; prop4?: boolean; }; let partialType: Partial<Type> = { prop1: "Default", prop4: false, }; console.log("The value of prop1 is " + partialType.prop1); console.log("The value of prop2 is " + partialType.prop2);
编译后,它将生成以下 JavaScript 代码:
var partialType = { prop1: "Default", prop4: false }; console.log("The value of prop1 is " + partialType.prop1); console.log("The value of prop2 is " + partialType.prop2);
输出
上述代码将产生以下输出:
The value of prop1 is Default The value of prop2 is undefined
TypeScript 中的 Required 类型
Required 实用类型允许我们转换类型,使其所有属性都成为必需的。当我们使用 Required 实用类型时,它会将所有可选属性变为必需属性。
示例
在这个例子中,Type 包含prop3可选属性。使用 Required 实用操作符转换 Type 后,prop3 也变成了必需属性。如果我们在创建对象时没有为prop3赋值,则会产生编译错误。
type Type = { prop1: string; prop2: string; prop3?: number; }; let requiredType: Required<Type> = { prop1: "Default", prop2: "Hello", prop3: 40, }; console.log("The value of prop1 is " + requiredType.prop1); console.log("The value of prop2 is " + requiredType.prop2);
编译后,它将生成以下 JavaScript 代码:
var requiredType = { prop1: "Default", prop2: "Hello", prop3: 40 }; console.log("The value of prop1 is " + requiredType.prop1); console.log("The value of prop2 is " + requiredType.prop2);
输出
上述代码将产生以下输出:
The value of prop1 is Default The value of prop2 is Hello
TypeScript 中的 Pick 类型
Pick 实用类型允许我们挑选其他类型的属性类型并创建一个新类型。用户需要使用字符串格式的类型键来选择具有其类型的键以包含在新类型中。如果用户想要选择具有其类型的多个键,则应使用联合操作符。
示例
在下面的示例中,我们从 type1 中选择了 color 和 id 属性,并使用 Pick 实用操作符创建了新类型。用户可以看到,当他们尝试访问newObj的 size 属性时,会报错,因为newObj对象的类型不包含 size 属性。
type type1 = { color: string; size: number; id: string; }; let newObj: Pick<type1, "color" | "id"> = { color: "#00000", id: "5464fgfdr", }; console.log(newObj.color); // This will generate a compilation error as a type of newObj doesn't contain the size property // console.log(newObj.size);
编译后,它将生成以下 JavaScript 代码:
var newObj = { color: "#00000", id: "5464fgfdr" }; console.log(newObj.color); // This will generate a compilation error as a type of newObj doesn't contain the size property // console.log(newObj.size);
输出
上述代码将产生以下输出:
#00000
TypeScript 中的 Omit 类型
Omit 从类型中删除键并创建一个新类型。它是 Pick 的反义词。我们使用Omit实用程序操作符的任何键都会从类型中删除这些键并返回一个新类型。
示例
在这个例子中,我们使用Omit实用类型从type1中省略了 color 和 id 属性,并创建了omitObj对象。当用户尝试访问omitObj的 color 和 id 属性时,会报错。
type type1 = { color: string; size: number; id: string; }; let omitObj: Omit<type1, "color" | "id"> = { size: 20, }; console.log(omitObj.size); // This will generate an error // console.log(omitObj.color); // console.log(omitObj.id)
编译后,它将生成以下 JavaScript 代码:
var omitObj = { size: 20 }; console.log(omitObj.size); // This will generate an error // console.log(omitObj.color); // console.log(omitObj.id)
输出
上述代码将产生以下输出:
20
TypeScript 中的 Readonly 类型
我们可以使用Readonly实用类型使所有类型都成为只读属性,从而使所有属性都不可变。因此,我们不能在第一次初始化后为只读属性赋值。
示例
在这个例子中,keyboard_type 包含三个不同的属性。我们使用了Readonly实用类型使 keyboard 对象的所有属性都成为只读属性。只读属性意味着我们可以访问它来读取值,但我们不能修改或重新赋值。
type keyboard_type = { keys: number; isBackLight: boolean; size: number; }; let keyboard: Readonly<keyboard_type> = { keys: 70, isBackLight: true, size: 20, }; console.log("Is there backlight in the keyboard? " + keyboard.isBackLight); console.log("Total keys in the keyboard are " + keyboard.keys); // keyboard.size = 30 // this is not allowed as all properties of the keyboard are read-only
编译后,它将生成以下 JavaScript 代码:
var keyboard = { keys: 70, isBackLight: true, size: 20 }; console.log("Is there backlight in the keyboard? " + keyboard.isBackLight); console.log("Total keys in the keyboard are " + keyboard.keys); // keyboard.size = 30 // this is not allowed as all properties of the keyboard are read-only
输出
上述代码将产生以下输出:
Is there backlight in the keyboard? true Total keys in the keyboard are 70
TypeScript 中的 ReturnType 类型
ReturnType 实用类型允许我们根据函数的返回类型为任何变量设置类型。例如,如果我们使用任何库函数并且不知道函数的返回类型,我们可以使用ReturnType实用程序操作符。
示例
在这个例子中,我们创建了func()函数,它接受一个字符串作为参数并返回相同的字符串。我们使用了 typeof 操作符在ReturnType实用程序操作符中识别函数的返回类型。
function func(param1: string): string { return param1; } // The type of the result variable is a string let result: ReturnType<typeof func> = func("Hello"); console.log("The value of the result variable is " + result);
编译后,它将生成以下 JavaScript 代码:
function func(param1) { return param1; } // The type of the result variable is a string var result = func("Hello"); console.log("The value of the result variable is " + result);
输出
上述代码将产生以下输出:
The value of the result variable is Hello
TypeScript 中的 Record 类型
Record实用类型创建一个对象。我们需要使用Record实用类型定义对象的键,它也接受类型并使用该类型的对象定义对象键。
示例
在下面的示例中,我们定义了 Employee 类型。之后,为了创建一个new_Employee对象,我们使用了 Record 作为类型实用程序。用户可以看到,Record 实用程序在new_Employee对象中创建了 Employee 类型的 Emp1 和 Emp2 对象。
此外,用户还可以看到我们如何访问new_Employee对象的 Emp1 和 Emp2 对象的属性。
type Employee = { id: string; experience: number; emp_name: string; }; let new_Employee: Record<"Emp1" | "Emp2", Employee> = { Emp1: { id: "123243yd", experience: 4, emp_name: "Shubham", }, Emp2: { id: "2434ggfdg", experience: 2, emp_name: "John", }, }; console.log(new_Employee.Emp1.emp_name); console.log(new_Employee.Emp2.emp_name);
编译后,它将生成以下 JavaScript 代码:
var new_Employee = { Emp1: { id: "123243yd", experience: 4, emp_name: "Shubham" }, Emp2: { id: "2434ggfdg", experience: 2, emp_name: "John" } }; console.log(new_Employee.Emp1.emp_name); console.log(new_Employee.Emp2.emp_name);
输出
上述代码将产生以下输出:
Shubham John
TypeScript 中的 NonNullable 类型
NonNullable实用程序操作符从属性类型中删除 null 和 undefined 值。它确保对象中的每个变量都存在且具有定义的值。
示例
在这个例子中,我们创建了 var_type,它也可以是 null 或 undefined。之后,我们使用NonNullable实用程序操作符使用了 var_type,我们可以观察到我们不能为变量赋值 null 或 undefined。
type var_type = number | boolean | null | undefined; let variable2: NonNullable<var_type> = false; let variable3: NonNullable<var_type> = 30; console.log("The value of variable2 is " + variable2); console.log("The value of variable3 is " + variable3); // The below code will generate an error // let variable4: NonNullable<var_type> = null;
编译后,它将生成以下 JavaScript 代码:
var variable2 = false; var variable3 = 30; console.log("The value of variable2 is " + variable2); console.log("The value of variable3 is " + variable3); // The below code will generate an error // let variable4: NonNullable = null;
输出
上述代码将产生以下输出:
The value of variable2 is false The value of variable3 is 30