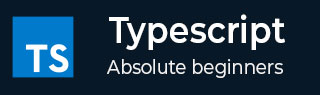
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null 与 undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - Function 构造函数
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - null 与 undefined
在 TypeScript 中,'undefined' 表示变量已声明但未赋值。另一方面,'null' 指的是一个不存在的对象,基本上是“空”或“无”。
您是否遇到过需要声明变量但稍后需要初始化它的场景?这通常发生在处理需要在获取 API 响应后初始化变量的 API 时。在这种情况下,可以使用 null 或 undefined 数据类型来表示值的缺失。
什么是 null?
在 TypeScript 中,'null' 是一个原始值,表示未为变量赋值。它被显式地赋值给变量以指示变量为空或不包含任何值。
让我们通过下面的示例了解如何在 TypeScript 中使用 'null'。
示例:null 的基本用法
在下面的代码中,我们定义了一个 'null' 类型变量。它表示变量 'a' 包含空值。在输出中,您可以看到它打印了一个 null 值。
// Using null value let a: null = null; console.log(a); // null
编译后,它将生成以下 JavaScript 代码。
// Using null value let a = null; console.log(a); // null
上述代码的输出如下:
null
示例:null 的数据类型
'null' 类型变量的数据类型是 object。
在这里,我们使用了 'typeof' 运算符来检查包含 null 值的变量的数据类型。它返回 object,您可以在输出中看到。
let a: null = null; console.log(typeof a); // Object
编译后,它将生成以下 JavaScript 代码。
let a = null; console.log(typeof a); // Object
上述代码的输出如下:
object
示例:重新初始化 null 变量
在下面的代码中,变量 'a' 的数据类型是数字或 null。最初,我们为它赋值了 null 值。之后,我们为变量 'a' 赋值了数值。
let a: number | null = null; console.log("The initial value of the variable a is: " + a); // null a = 10; console.log("The value of the variable a is: " + a); // 10
编译后,它将生成以下 JavaScript 代码。
let a = null; console.log("The initial value of the variable a is: " + a); // null a = 10; console.log("The value of the variable a is: " + a); // 10
其输出如下:
The initial value of the variable a is: null The value of the variable a is: 10
什么是 undefined?
当您声明变量但不赋值时,TypeScript 会自动为变量赋值 'undefined'。当您没有从函数中返回任何值时,它将返回 undefined 值。但是,您也可以显式地为 'undefined' 类型的变量赋值 undefined 值。
让我们通过下面的示例了解 undefined。
示例:Undefined 值
在下面的代码中,我们定义了变量 'a' 但没有用值初始化它。因此,它的值为 undefined,您可以在输出中看到。
let a: number; console.log("The value of a is " + a);
编译后,它将显示以下错误:
Variable 'a' is used before being assigned.
并且它还会生成以下 JavaScript 代码。
let a; console.log("The value of a is " + a);
上述 JavaScript 代码的输出如下:
The value of a is undefined
示例:不从函数返回值
在下面的代码中,我们定义了 greet() 函数,该函数不返回任何值。
之后,我们调用了 greet() 函数并将它的结果存储在变量 'a' 中。变量的值是 undefined,因为 greet() 函数没有返回值。
// Not returning a value from the function function greet(name: string): void { console.log(`Hello ${name}`); } let a = greet('John'); console.log(a); // undefined
编译后,它将生成以下 JavaScript 代码。
// Not returning a value from the function function greet(name) { console.log(`Hello ${name}`); } let a = greet('John'); console.log(a); // undefined
上述代码的输出如下:
Hello John Undefined
示例:使用 undefined 类型
在这里,我们使用了 'undefined' 数据类型和变量 'a',并为其赋值了 undefined 值。
变量 'a' 的类型是 undefined,而不是像 'null' 类型变量那样的 object。
let a: undefined = undefined; console.log(a); // undefined console.log(typeof a); // undefined
编译后,它将生成以下 JavaScript 代码。
let a = undefined; console.log(a); // undefined console.log(typeof a); // undefined
输出如下:
undefined undefined
Null 与 Undefined:主要区别
您已经了解了 Null 和 Undefined。现在,让我们看看它们之间的主要区别。
特性 | null | undefined |
---|---|---|
含义 | 显式无值 | 未初始化 |
典型用法 | 故意为空或缺失的值 | 变量已声明但尚未赋值 |
类型注解 | 拥有自己的类型 null | 拥有自己的类型 undefined |
默认行为 | 不会触发默认函数参数 | 触发默认函数参数 |
函数参数 | 用于表示参数不应具有值 | 表示缺少参数或可选参数 |
对象属性 | 可用于指示故意设置为无值的属性 | 用于可能未初始化的属性 |
操作处理 | 必须在逻辑中显式处理以避免错误 | 通常使用默认值或可选链处理 |
让我们看看下面的示例,这些示例显示了在哪里使用 null 和 undefined 值。
示例:对象属性
在下面的代码中,'user' 类型具有 'name'、'age' 和 'email' 属性。如果用户的年龄不可用,则 'age' 属性可以接受 null 值。'email' 属性是可选的,因此我们在定义对象时不使用它也没关系。
'user1' 对象包含具有 null 值的 'age' 属性。'user2' 值不包含 'email' 属性。因此,对于 'user2' 对象,它是 undefined。
type User = { name: string; age: number | null; email?: string; }; let user1: User = { name: "John Doe", age: null, // Explicitly no age provided email: "[email protected]" }; let user2: User = { name: "Jane Doe", age: 25 // email is optional and thus can be undefined }; console.log(user1); // Output: { name: "John Doe", age: null, email: "[email protected]" } console.log(user2); // Output: { name: "Jane Doe", age: 25 }
编译后,它将生成以下 JavaScript 代码。
let user1 = { name: "John Doe", age: null, // Explicitly no age provided email: "[email protected]" }; let user2 = { name: "Jane Doe", age: 25 // email is optional and thus can be undefined }; console.log(user1); // Output: { name: "John Doe", age: null, email: "[email protected]" } console.log(user2); // Output: { name: "Jane Doe", age: 25 }
上述代码的输出如下:
{ name: 'John Doe', age: null, email: '[email protected]' } { name: 'Jane Doe', age: 25 }
这样,您可以使用 null 或 undefined 来表示代码中值的缺失。