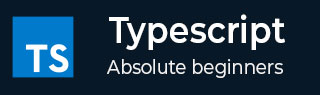
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型保护
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 函数类型
TypeScript 中的函数类型允许我们定义函数的类型。
函数类型描述了函数的形状,包括参数和返回值。
函数类型包括以下内容:
函数参数的类型
函数的返回值类型
为函数添加类型
为函数添加类型是指为其参数和返回值指定类型。类似于我们为变量添加类型的方式,我们也可以为函数参数和返回值添加类型注解,就像我们为变量定义类型一样。
让我们看看使用一个简单的函数如何为函数添加类型:
function add (x: number, y: number): number { return x + y; } let addNums = function (x: number, y: number): number { return x + y; }
在第一个示例中,我们为参数 x 和 y 以及函数的返回值添加了类型。TypeScript 可以使用参数的类型推断返回值类型。这意味着我们可以选择省略返回值类型。
在第二个示例中,我们将一个函数赋值给变量 addNums。虽然我们没有明确定义 addNums 的类型,但 TypeScript 可以推断其类型。
现在让我们检查一下 TypeScript 编译器如何推断赋值给函数的变量的类型。
TypeScript 推断变量的类型
在下面的示例中,我们将一个函数赋值给变量 add:
let add = function (a: number, b: number): number { return a + b; }
TypeScript 编译器将变量 add 的类型推断为:
(a: number, b: number) => number
查看下面的截图,TypeScript 编辑器推断变量 add 的类型。

这里,“(a: number, b: number) => number”实际上是一个函数类型表达式。
函数类型表达式是声明保存函数的变量的便捷方式。
TypeScript 函数类型表达式
TypeScript 中的函数类型可以使用函数类型表达式来定义。函数类型表达式在语法上类似于箭头函数。
语法
在函数类型表达式中,我们指定参数的类型和返回值类型。语法如下:
(para1: type1, para2: type2, ...) => returnType
其中:
(para1: type1, para2: type2, ...):这定义了函数的参数及其类型。我们可以添加多个参数,用逗号分隔。
=>:胖箭头将参数列表与返回值类型分隔开。
returnType:这是函数返回值的类型。
演示 TypeScript 中函数类型的示例:
(name: string) => void
此函数类型描述为一个函数,它带有一个名为 name 的字符串类型参数,并且没有返回值(由 void 指示)。
(a: number, b: number) => number
在上面的函数类型中,它接受两个名为 a 和 b 的数字类型参数,并返回一个数字类型的值。
示例
让我们使用函数类型表达式声明一个名为 addFun 的函数类型变量:
let addFun: (x: number, y: number) => number = function (x:number, y: number): number { return x + y } console.log(addFun(2, 3));
在上面的示例中,我们将一个函数添加到 addFun,该函数接受两个数字作为参数并返回它们的和。类型注解 “(x: number, y: number) => number”阐明了此行为。
编译此 TypeScript 代码后,它将生成以下 JavaScript 代码。
let addFun = function (x, y) { return x + y; }; console.log(addFun(2, 3));
以上示例代码的输出如下:
5
示例
让我们来看另一个例子:
const greet: (name: string) => string = (name) => { return `Hello, ${name}!`; }; console.log(greet("Shahid"));
在下面的示例中,我们定义了一个名为 greet 的常量,其类型为 (name: string) => string。此函数接受一个字符串作为参数并返回一个字符串。
上面的 TypeScript 代码将编译为以下 JavaScript 代码。
const greet = (name) => { return `Hello, ${name}!`; }; console.log(greet("Shahid"));
以上示例代码的输出如下:
Hello, Shahid!
声明函数类型变量
在 TypeScript 中,我们可以使用函数类型表达式声明具有特定函数类型的变量。
让我们声明一个函数类型的变量:
let add: (a: number, b: number) => number
这声明了一个函数类型的变量 add。该函数接受两个数字作为参数并返回一个数字。
示例
在下面的示例中,我们使用函数表达式声明函数类型的变量 greet。然后用一个函数赋值给变量,该函数接受一个字符串类型的参数并返回一个字符串。
let greet: (name: string) => string; greet = (name) => { return `Hello, ${name}!`; }; console.log(greet('John'));
编译后,它将生成以下 JavaScript 代码:
let greet; greet = (name) => { return `Hello, ${name}!`; }; console.log(greet('John'));
输出如下:
Hello, John!
将函数参数指定为函数
我们可以指定函数类型的参数。在下面的示例中,参数 fn 指定为函数类型。它接受一个字符串类型的参数,并且不返回任何值。
函数 greet 接受一个名为 fn 的参数,其类型为函数。
function greet (fn: (s: string) => void) { fn ("Welcome to Tutorials Point!") } function print (str: string) { console.log(str); } greet(print);
编译后,它将生成以下 JavaScript 代码。
function greet(fn) { fn("Welcome to Tutorials Point!"); } function print(str) { console.log(str); } greet(print);
以上示例代码的输出如下:
Welcome to Tutorials Point!
使用类型别名表示函数类型
我们可以创建一个类型别名来表示特定的函数类型并在代码中使用它:
type funcType = (s: string) => void; function greet (fn: funcType) { fn ("Welcome to Tutorials Point!") } function print (str: string) { console.log(str); } greet(print);
在这里,我们为函数类型 “(s: string) => void”定义了一个类型别名 funcType。接下来,我们在函数 greet 的定义中使用 funcType 作为参数类型。
编译后,它将生成以下 JavaScript 代码。
function greet(fn) { fn("Welcome to Tutorials Point!"); } function print(str) { console.log(str); } greet(print);
以上示例的输出如下:
Welcome to Tutorials Point!