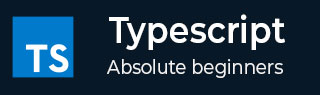
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript 与 JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null 与 undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策制定
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 只读属性
TypeScript 提供了 readonly 关键字,用于将类、类型或接口中的属性设为只读。 只读属性 可以从类外部访问,但其值不能被修改。
通过使用只读属性,您可以确保没有人可以在对象外部修改属性,但他们可以读取属性的值。
语法
要在 TypeScript 中定义只读属性,我们用 readonly 关键字作为属性名的前缀。
readonly propName: type;
其中,
在上述语法中,**'readonly'** 是一个关键字。
**'propName'** 是只读属性的属性名。
**'type'** 是只读属性的属性类型。
封装实体可以在 TypeScript 中是 类 或 接口。
您可以用所需的只读属性名称替换 propertyName,用适当的数据类型替换 type。
带接口的只读属性
接口用于定义对象的原型。我们可以在接口中定义只读属性。让我们通过下面的例子来理解它。
示例
在下面的代码中,我们定义了包含 model、year 和 fuel 属性的 Car 接口。这里,fuel 属性是只读的,它只能在创建对象时初始化,但在创建对象后不能更改。
之后,我们创建了 Car 类型的对象并初始化了所有属性。
您可以尝试更改 car 对象的 fuel 属性的值,并观察错误。
// Defining the interface for the car interface Car { model: string; year: number; readonly fuel: string; } // Defining the car object let car: Car = { model: 'BMW', year: 2019, fuel: 'petrol' } console.log(car.model); console.log(car.year); console.log(car.fuel); // Error: Cannot assign to 'fuel' because it is a read-only property. // car.fuel = 'diesel';
编译后,它将生成以下 JavaScript 代码。
// Defining the car object let car = { model: 'BMW', year: 2019, fuel: 'petrol' }; console.log(car.model); console.log(car.year); console.log(car.fuel); // Error: Cannot assign to 'fuel' because it is a read-only property. // car.fuel = 'diesel';
输出
上面示例代码的输出如下:
BMW 2019 petrol
带类的只读属性
类也可以包含类似于接口的只读属性。只读属性的值可以在创建对象时在 constructor() 方法中初始化。您不能使用类实例更改类的只读属性的值。
示例
在下面的代码中,我们定义了包含 model 和 price 属性的 car 类。它还包含 'type' 只读属性。
在 constructor() 方法中,我们初始化了所有属性的值,包括 'type' 只读属性。
display() 方法打印所有属性的值。
之后,我们创建了 car 类的对象。现在,您可以尝试更改 car 对象的 'type' 属性的值,它将抛出错误,因为它时只读的。
// Defining the class for car class Car { // Properties model: string; price: number; readonly type: string = 'Car'; // Constructor constructor(model: string, price: number, type: string) { this.model = model; this.price = price; this.type = type; } // Method display(): void { console.log(`Model: ${this.model}, Price: ${this.price}, Type: ${this.type}`); } } // Creating object of Car class let car = new Car('BMW', 1000000, 'Sedan'); car.display(); // Try to change the value of readonly property // car.type = 'SUV'; // Error: Cannot assign to 'type' because it is a read-only property.
编译后,它将生成以下 JavaScript 代码。
// Defining the class for car class Car { // Constructor constructor(model, price, type) { this.type = 'Car'; this.model = model; this.price = price; this.type = type; } // Method display() { console.log(`Model: ${this.model}, Price: ${this.price}, Type: ${this.type}`); } } // Creating object of Car class let car = new Car('BMW', 1000000, 'Sedan'); car.display(); // Try to change the value of readonly property // car.type = 'SUV'; // Error: Cannot assign to 'type' because it is a read-only property.
输出
上面代码示例的输出如下:
Model: BMW, Price: 1000000, Type: Sedan
带类型别名的只读属性
类型别名用于为对象定义类型。它也可以包含类似于接口的只读属性。
示例
在下面的代码中,'Point' 是一个包含 'x' 和 'y' 属性的类型别名,并且两者都是只读的。
之后,我们定义了类型为 'Point' 的 P1 点,并在定义时初始化了 'X' 和 'Y' 属性的值。
接下来,尝试更改 'P1' 点的任何属性的值。它将抛出错误,因为 P1 的两个属性都是只读的。
// Readonly Properties with type alias type Point = { readonly x: number; readonly y: number; } let p1: Point = { x: 10, y: 20 }; // p1.x = 5; // Error console.log(p1.x, p1.y);
编译后,它将生成以下 JavaScript 代码。
let p1 = { x: 10, y: 20 }; // p1.x = 5; // Error console.log(p1.x, p1.y);
输出
输出如下:
10 20
Const 与只读属性
'const' 关键字类似于 'readonly' 关键字,但有一些细微的差别。
- 'const' 关键字用于声明常量变量,而 'readonly' 关键字用于声明对象的只读属性。
- 您需要在声明时为使用 'const' 关键字声明的变量赋值,但对于 'readonly' 属性,您可以在创建对象时赋值。
- 'const' 变量的值在声明后不能更改,而 'readonly' 属性的值不能在对象或类外部更改。
何时使用 Readonly
- **数据完整性:**当您希望确保对象的某些属性在初始赋值后不会更改时。
- **函数式编程:**它有助于将不变性作为基石的编程范式。
- **API 合同:**在创建公开给外部用户的对象时,您需要保证内部状态不会意外更改。