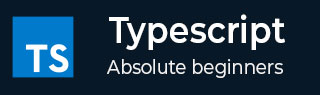
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - Function 构造函数
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型运算符
- TypeScript - typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用工具类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 鸭子类型
鸭子类型
对象的类型由其行为(例如方法和属性)而不是其类来决定的情况称为“鸭子类型”。
TypeScript 中接口的使用使得鸭子类型成为可能。其中接口描述了对象必须具有的方法和属性集才能属于该类型。
例如,如果一个接口定义了函数,那么任何具有名为“myFunc()”的方法的对象都可以被视为属于特定类型,而不管其类是什么。
鸭子类型强调通过考虑对象的方法和属性来评估对象是否适合特定任务,而不是考虑其实际类型。接口说明了对象必须具有的属性和方法集,才能被认为是特定目的的“鸭子类型”。
鸭子类型的益处
鸭子类型的主要优势之一是使代码更加灵活和可重用。代码可以与任何具有所需方法和属性的对象一起工作,而不仅仅是特定类型的对象,并且可以在各种情况下使用而无需修改。鸭子类型还通过允许在单个代码库中互换使用不同类型的对象来提高代码重用性。
TypeScript 中鸭子类型的示例
以下是如何在 TypeScript 中使用鸭子类型的示例:
定义一个接口来表示您希望对象具有的行为。例如:
interface Duck { quack(): void; }
创建一个实现该接口的类。例如:
class MallardDuck implements Duck { quack(): void { console.log("Quack!"); } }
创建该类的实例并将其用作接口定义的类型。
let duck: Duck = new MallardDuck(); duck.quack(); // Output: "Quack!"
创建另一个也实现该接口的类:
class RubberDuck implements Duck { quack(): void { console.log("Squeak!"); } }
将新的类实例用作接口定义的相同类型。
let duck: Duck = new RubberDuck(); duck.quack(); // Output: "Squeak!"
正如您所看到的,MallardDuck 和 RubberDuck 类都实现了 Duck 接口,并且 duck 变量可以赋值给这两个类的实例。类型由接口中定义的行为(方法和属性)决定,而不是由类决定。
还需要注意的是,在 TypeScript 中,您可以使用 typeof 关键字在运行时检查对象的类型,以及对象是否具有预期的方法或属性。
示例
在这个例子中,Bird 和 Plane 类实现了 Flyable 接口,该接口需要一个 fly() 方法。这两个“鸭子类型”可以在 goFly() 函数中互换使用。该函数不关心传递给它的对象的实际类型,只要它有一个可以调用的 fly() 方法即可。
interface Flyable { fly(): void; } class Bird implements Flyable { fly(): void { console.log("Bird is flying"); } } class Plane implements Flyable { fly(): void { console.log("Plane is flying"); } } function goFly(flyable: Flyable) { flyable.fly(); } let bird = new Bird(); let plane = new Plane(); goFly(bird); // Prints "Bird is flying" goFly(plane); // Prints "Plane is flying"
编译后,它将生成以下 JavaScript 代码:
var Bird = /** @class */ (function () { function Bird() { } Bird.prototype.fly = function () { console.log("Bird is flying"); }; return Bird; }()); var Plane = /** @class */ (function () { function Plane() { } Plane.prototype.fly = function () { console.log("Plane is flying"); }; return Plane; }()); function goFly(flyable) { flyable.fly(); } var bird = new Bird(); var plane = new Plane(); goFly(bird); // Prints "Bird is flying" goFly(plane); // Prints "Plane is flying"
输出
上述代码将产生以下输出:
Bird is flying Plane is flying
示例
总的来说,鸭子类型是一个强大的编程概念,它允许在 TypeScript 代码中实现更大的灵活性和可重用性,因为只要对象具有相同的方法和属性,就可以互换使用不同类型的对象。在这个例子中,Driveable 接口、Car 和 Truck 类展示了相同的情况。
interface Driveable { drive(): void; } class Car implements Driveable { drive(): void { console.log("Car is driving"); } } class Truck implements Driveable { drive(): void { console.log("Truck is driving"); } } function goDrive(driveable: Driveable) { driveable.drive(); } let car = new Car(); let truck = new Truck(); goDrive(car); // Prints "Car is driving" goDrive(truck); // Prints "Truck is driving"
编译后,它将生成以下 JavaScript 代码:
var Car = /** @class */ (function () { function Car() { } Car.prototype.drive = function () { console.log("Car is driving"); }; return Car; }()); var Truck = /** @class */ (function () { function Truck() { } Truck.prototype.drive = function () { console.log("Truck is driving"); }; return Truck; }()); function goDrive(driveable) { driveable.drive(); } var car = new Car(); var truck = new Truck(); goDrive(car); // Prints "Car is driving" goDrive(truck); // Prints "Truck is driving"
输出
上述代码将产生以下输出:
Car is driving Truck is driving
鸭子类型背后的主要思想是,代码应该编写成与任何具有所需方法和属性的对象一起工作,而不是编写成与特定对象一起工作。这可以使代码更灵活、更可重用,允许您互换使用不同类型的对象而无需更改代码。