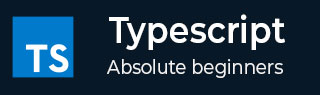
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript 与 JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 杂项
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 布尔值
TypeScript 的布尔类型表示逻辑值,例如 true 或 false。逻辑值用于控制程序中执行流程。由于 JavaScript 提供了布尔原始类型和对象类型,因此 TypeScript 添加了类型注解。我们可以创建布尔原始类型以及布尔对象。
我们可以使用 Boolean() 构造函数和 new 关键字创建布尔对象。
要将非布尔值转换为布尔值,我们应该使用 Boolean() 函数或双重否定 (!!) 运算符。我们不应将 Boolean 构造函数与 new 关键字一起使用。
语法
要在 TypeScript 中声明布尔值(原始值),我们可以使用关键字“boolean”。
let varName: boolean = true;
在上述语法中,我们声明一个布尔变量 varName 并为其赋值 true。
要创建布尔对象,我们使用 Boolean() 构造函数和 new 关键字。
const varName = new Boolean(value);
在上述语法中,value 是要转换为布尔对象的表达式。使用 new 的 Boolean() 构造函数返回一个包含布尔值的对象。
例如,
const isTrue = new Boolean(true);
在上面的示例中,isTrue 持有值 true,而 isFalse 持有值 false。
类型注解
TypeScript 中的类型注解是可选的,因为 TypeScript 可以自动推断变量的类型。我们可以使用 boolean 关键字来注解布尔变量的类型。
let isPresent : boolean = true; // with type annotation let isPresent = true // without type annotation
与变量一样,函数参数和返回类型也可以进行注解。
真值和假值
在 TypeScript 中,假值是被评估为 false 的值。有六个假值:
- false
- 0(零)
- 空字符串("")
- null
- undefined
- NaN
所有其他值都是真值。
将非布尔值转换为布尔值
所有上述讨论的假值都被转换为 false,真值被转换为 true。要将非布尔值转换为布尔值,我们可以使用 Boolean() 函数和双重否定 (!!) 运算符。
使用 Boolean() 函数
TypeScript 中的 Boolean() 函数将非布尔值转换为布尔值。它返回一个原始布尔值。
let varName = Boolean(value);
value 是要转换为布尔值的表达式。
示例
在下面的示例中,我们使用 Boolean() 函数将非布尔值转换为布尔值。
const myBoolean1 = Boolean(10); console.log(myBoolean1); // true const myBoolean2 = Boolean(""); console.log(myBoolean2); // false
编译后,以上代码将在 JavaScript 中生成相同的代码。执行 JavaScript 代码将产生以下输出:
true false
使用双重否定 (!!) 运算符
TypeScript 中的双重否定 (!!) 运算符将非布尔值转换为布尔值。它返回一个原始布尔值。
let varName = !!(value);
value 是要转换为布尔值的表达式。
示例
在下面的示例中,我们使用双重否定 (!!) 运算符将非布尔值转换为布尔值。
const myBoolean1 = !!(10); console.log(myBoolean1); // true const myBoolean2 = !!(""); console.log(myBoolean2); // false
编译后,以上代码将在 JavaScript 中生成相同的代码。执行 JavaScript 代码将产生以下输出:
true false
布尔运算
TypeScript 中的布尔运算或逻辑运算可以使用三个逻辑运算符来执行:逻辑 AND、OR 和 NOT 运算符。这些运算返回一个布尔值(true 或 false)。
示例:逻辑 AND (&&)
在下面的示例中,我们定义了两个布尔变量 x 和 y。然后执行这些变量的逻辑 AND (&&)。
let x: boolean = true; let y: boolean = false; let result: boolean = x && y; console.log(result); // Output: false
编译后,它将生成以下 JavaScript 代码。
var x = true; var y = false; var result = x && y; console.log(result); // Output: false
以上示例代码的输出如下:
false
示例:逻辑 OR (||)
在下面的示例中,我们执行两个布尔变量 x 和 y 的逻辑 OR (||) 运算。
let x: boolean = true; let y: boolean = false; let result: boolean = x || y; console.log(result); // Output: true
编译后,它将生成以下 JavaScript 代码。
var x = true; var y = false; var result = x || y; console.log(result); // Output: true
以上示例代码的输出如下:
true
示例:逻辑 NOT (!)
在下面的示例中,执行布尔变量 isPresent 的逻辑 NOT (!) 运算。
let isPresent: boolean = false; let isAbsent:boolean = !isPresent; console.log(isAbsent); // Output: true
编译后,它将在 JavaScript 中生成相同的代码。
以上示例代码的输出如下:
true
使用布尔值的条件表达式
示例:If 语句
下面的示例展示了如何在 if else 语句中使用布尔值的条件表达式。
let age: number = 25; let isAdult: boolean = age >= 18; if (isAdult) { console.log('You are an adult.'); } else { console.log('You are not an adult.'); }
编译后,它将生成以下 JavaScript 代码:
let age = 25; let isAdult = age >= 18; if (isAdult) { console.log('You are an adult.'); } else { console.log('You are not an adult.'); }
以上示例代码的输出如下:
You are an adult.
示例:条件语句(三元运算符)
尝试以下示例:
let score: number = 80; let isPassing: boolean = score >= 70; let result: string = isPassing ? 'Pass' : 'Fail'; console.log(result); // Output: Pass
编译后,它将生成以下 JavaScript 代码:
let score = 80; let isPassing = score >= 70; let result = isPassing ? 'Pass' : 'Fail'; console.log(result); // Output: Pass
以上示例代码的输出如下:
Pass
TypeScript Boolean 与 boolean
Boolean 与 boolean 类型不同。Boolean 不引用原始值。而 boolean 是 TypeScript 中的原始数据类型。您应该始终使用小写字母的 boolean。
布尔对象
如上所述,我们可以使用 Boolean 构造函数和 new 关键字创建一个包含布尔值的布尔对象。Boolean 包装类为我们提供了不同的属性和方法来处理布尔值。
布尔属性
以下是布尔对象属性的列表:
序号 | 属性 & 描述 |
---|---|
1 | constructor 返回对创建对象的 Boolean 函数的引用。 |
2 | prototype prototype 属性允许您向对象添加属性和方法。 |
在以下部分,我们将有一些示例来说明布尔对象的属性。
布尔方法
以下是布尔对象方法及其描述的列表。
序号 | 方法 & 描述 |
---|---|
1 | toSource() 返回一个包含布尔对象源代码的字符串;您可以使用此字符串创建等效的对象。 |
2 | toString() 根据对象的值返回“true”或“false”字符串。 |
3 | valueOf() 返回布尔对象的原始值。 |
在以下部分,我们将有一些示例来演示布尔方法的使用。