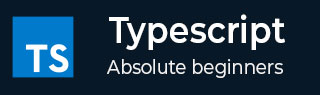
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 杂项
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 日期对象
在 TypeScript 中,'Date' 对象是一个内置对象,允许开发人员创建和管理日期和时间。它直接继承自 JavaScript,因此包含与 JavaScript 中的 Date 对象相同的方法。但是,您需要确保 TypeScript 中 Date 对象的类型和 Date 方法的返回值类型。
语法
您可以按照以下语法在 TypeScript 中使用 Date 对象。
let date: Date = new Date(); // Date Constructor // OR date = new Date(milliseconds); // Passes milliseconds // OR date = new Date(date_String); // Passes date string // OR date = new Date(year, month, day, hour, minute, second, milli_second);
在上面的语法中,我们解释了使用 Date() 构造函数的 4 种不同方法,它们接受不同的参数。
参数
milliseconds:它是自 1971 年 1 月 1 日以来的毫秒总数。
date_String:它是一个日期字符串,包含年份、月份、日期等。
year, month, day, hour, minute, second, milli_second:Date() 构造函数根据这些参数创建一个新的日期。
返回值
Date 构造函数根据传递的参数返回日期字符串。如果我们不传递任何参数,它将返回带有当前时间的日期字符串。
示例
让我们通过一些 TypeScript 示例来进一步了解日期对象。
示例:创建新的日期
在下面的代码中,首先,我们使用 Date() 构造函数创建了一个日期,并且没有传递任何参数。它返回当前日期和时间。
接下来,我们传递了毫秒作为参数,它根据毫秒总数返回日期。
接下来,我们将日期字符串格式作为 Date() 构造函数的参数传递,它根据日期字符串返回一个新日期。
接下来,我们将年份、月份、日期等作为 Date() 构造函数的单独参数传递,它使用这些参数创建一个新的日期字符串。
let date: Date = new Date(); // Date Constructor console.log(date); // Output: Current Date and Time date = new Date(10000000000); // Passes milliseconds console.log(date); // Output: 1970-04-26T17:46:40.000Z date = new Date('2020-12-24T10:33:30'); // Passes date string console.log(date); // Output: 2020-12-24T10:33:30.000Z date = new Date(2020, 11, 24, 10, 33, 30, 0); // Passes year, month, day, hour, minute, second, millisecond console.log(date); // Output: 2020-12-24T10:33:30.000Z
编译后,它将生成以下 JavaScript 代码。
let date = new Date(); // Date Constructor console.log(date); // Output: Current Date and Time date = new Date(10000000000); // Passes milliseconds console.log(date); // Output: 1970-04-26T17:46:40.000Z date = new Date('2020-12-24T10:33:30'); // Passes date string console.log(date); // Output: 2020-12-24T10:33:30.000Z date = new Date(2020, 11, 24, 10, 33, 30, 0); // Passes year, month, day, hour, minute, second, millisecond console.log(date); // Output: 2020-12-24T10:33:30.000Z
输出
上述示例代码的输出将显示当前日期和提供的自定义日期。
示例:访问日期和时间组件
您可以使用 getFullYear() 方法从日期字符串中获取年份。类似地,getMonth() 和 getDate() 方法分别用于从日期字符串中获取月份和日期。
let someDate = new Date(); console.log(`Year: ${someDate.getFullYear()}`); // Outputs current year console.log(`Month: ${someDate.getMonth()}`); // Outputs current month (0-indexed) console.log(`Day: ${someDate.getDate()}`); // Outputs current day
编译后,它将生成相同的 JavaScript 代码。
let someDate = new Date(); console.log(`Year: ${someDate.getFullYear()}`); // Outputs current year console.log(`Month: ${someDate.getMonth()}`); // Outputs current month (0-indexed) console.log(`Day: ${someDate.getDate()}`); // Outputs current day
输出
上述示例代码的输出将显示当前日期的年份、月份和日期。
示例:向当前日期添加天数
我们可以使用不同的方法来操作日期字符串。这里,我们使用了 setDate() 方法将日期字符串中的天数增加 7 天。
getDate() 方法返回日期,我们向其添加 7。然后,我们将新的一天传递给 setDate() 方法的参数。
setDate() 方法会自动管理更改月份或年份。例如,如果当前日期是 30,并且您想向其添加 7 天,它将返回带有下一月的新日期。
let date = new Date(); date.setDate(date.getDate() + 7); // Adds 7 days to the current date console.log(date); // Outputs the date 7 days in the future
编译后,它将生成相同的 JavaScript 代码。
let date = new Date(); date.setDate(date.getDate() + 7); // Adds 7 days to the current date console.log(date); // Outputs the date 7 days in the future
输出
它的输出将显示比当前日期早七天的日期。
示例:格式化日期
我们可以使用不同的选项来格式化日期字符串。这里,我们使用了 DateTimeFormatOptions() 方法来格式化日期字符串。
它将 'en-US' 作为第一个参数,这是一个国家代码,并将选项对象作为第二个参数。选项对象包含星期几、年份、月份和日期的格式详细信息。
let today = new Date(); let options: Intl.DateTimeFormatOptions = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; let formattedDate = new Intl.DateTimeFormat('en-US', options).format(today); console.log(formattedDate); // E.g., 'Tuesday, April 27, 2024'
编译后,它将生成以下 JavaScript 代码。
let today = new Date(); let options = { weekday: 'long', year: 'numeric', month: 'long', day: 'numeric' }; let formattedDate = new Intl.DateTimeFormat('en-US', options).format(today); console.log(formattedDate); // E.g., 'Tuesday, April 27, 2024'
输出
上述示例代码的输出将生成以美国格式格式化的今天日期。
示例:处理时区
这里,我们在下面的代码中处理不同的时区。首先,我们使用 Date 对象的 UTC() 方法根据 UTC 创建日期,并将其作为 Date() 构造函数的参数传递。
接下来,我们使用 toLocaleString() 方法根据时区更改日期字符串。toLocaleString() 方法将国家代码作为第一个参数,并将包含 timeZone 属性的对象作为第二个参数。
let utcDate = new Date(Date.UTC(2024, 0, 1, 0, 0, 0)); console.log(utcDate.toISOString()); // Outputs '2024-01-01T00:00:00.000Z' console.log(utcDate.toLocaleString('en-US', { timeZone: 'America/New_York' })); // Adjusts to Eastern Time
编译后,它将生成相同的 JavaScript 代码。
let utcDate = new Date(Date.UTC(2024, 0, 1, 0, 0, 0)); console.log(utcDate.toISOString()); // Outputs '2024-01-01T00:00:00.000Z' console.log(utcDate.toLocaleString('en-US', { timeZone: 'America/New_York' })); // Adjusts to Eastern Time
输出
其输出如下:
2024-01-01T00:00:00.000Z 12/31/2023, 7:00:00 PM
TypeScript 中的 Date 对象继承自 JavaScript,提供了一套全面的功能来管理日期和时间。通过有效地利用 Date 对象,开发人员可以执行各种日期和时间操作,这对于依赖时间数据的应用程序至关重要。