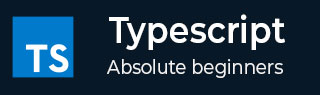
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型操作符
- TypeScript - Typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - any 类型
TypeScript 中的 any 类型是一种特殊的类型,可以表示任何值。它通常用于变量类型未知或尚未定义的情况。在将 JavaScript 代码转换为 TypeScript 时,any 类型非常有用。
any 类型在传统意义上并不是一种类型。它更像是一个占位符,告诉 TypeScript 编译器忽略特定变量、函数等的类型检查。
可以表示任何值
使用 any 类型声明的变量可以保存任何数据类型的值 -
let x: any; x = "Hello"; x = 23; x = true;
这里变量 x 使用 any 类型声明。这允许我们将任何值(字符串、数字、布尔值等)赋予该变量。
当使用 typeof 运算符检查时,any 类型变量的类型为 undefined -
let x: any; console.log(typeof x) // undefined
编译后,它将生成以下 JavaScript 代码 -
let x; console.log(typeof x); // undefined
以上示例代码的输出如下 -
undefined
示例
让我们通过以下 TypeScript 示例了解 any 类型 -
let x: any; console.log(typeof x); x = "Hello"; console.log(typeof x); x = 23; console.log(typeof x); x = true; console.log(typeof x);
这里变量声明为 any 类型。然后用不同类型的值(字符串、数字和布尔值)对其进行赋值。
编译后,它将生成以下 JavaScript 代码 -
let x; console.log(typeof x); x = "Hello"; console.log(typeof x); x = 23; console.log(typeof x); x = true; console.log(typeof x);
以上示例代码的输出如下 -
undefined string number boolean
any 类型的函数参数
您还可以定义一个参数为 any 类型的函数 -
function func(para: any){ }
这里函数 func 可以接受任何类型的参数 - 数字、字符串、布尔值等。
示例
让我们举个例子,
function greet(name: any): string{ return `Hello Mr. ${name}`; } console.log(greet('Shahid')); console.log(greet(123));
函数 greet() 定义了一个 any 类型的参数。因此它可以接受任何类型的参数(数字、字符串等)。
我们调用了 greet() 函数,传递了字符串和数字类型的两个不同值。您可以注意到它对这两种类型的参数都有效。
编译后,以上 TypeScript 代码将生成以下 JavaScript 代码 -
function greet(name) { return `Hello Mr. ${name}`; } console.log(greet('Shahid')); console.log(greet(123));
以上示例代码的输出如下 -
Hello Mr. Shahid Hello Mr. 123
any 类型的对象
也可以定义 any 类型的对象。此类对象可以具有任何类型的属性。让我们举个例子 -
const student: any = { name: "John Doe", age: 32, isEnrolled: true, }
这里 student 对象使用 any 声明。它包含三个不同类型的属性。
示例
尝试以下示例,
const student: any = { name: "John Doe", age: 32, isEnrolled: true, } console.log(student.name); console.log(student.age); console.log(student.isEnrolled);
编译后,它将生成以下 JavaScript 代码 -
const student = { name: "John Doe", age: 32, isEnrolled: true, }; console.log(student.name); console.log(student.age); console.log(student.isEnrolled);
以上示例代码的输出如下 -
John Doe 32 true
为什么要使用 any 类型?
使用 any 类型的一个原因是,当您处理未进行类型检查的代码时。例如,如果您使用现有的 JavaScript 代码,则 any 类型在将 JavaScript 代码转换为 TypeScript 时非常有用。如果您使用的是未用 TypeScript 编写的第三方库,则可能需要在 TypeScript 代码中对变量使用 any 类型。
使用 any 类型的另一个原因是,当您不确定值的类型是什么时。例如,接受用户输入的函数可以使用 any 类型来处理来自用户的任何类型的数据。
建议在新 TypeScript 代码中避免使用 any 类型。建议在使用 any 类型时要谨慎。如果您过于频繁地使用 any 类型,您的代码将变得不太类型安全。
类型断言
使用类型断言缩小 any 变量的类型 -
let value: any = "hello world"; let lenStr: number = (value as string).length; console.log(lenStr);
在以上代码中,变量 value 定义为 any 类型。然后将其缩小到字符串类型。
编译后,它将生成以下 JavaScript 代码 -
let value = "hello world"; let lenStr = value.length; console.log(lenStr);
以上代码的输出如下 -
11
注意
如果不谨慎使用 any 类型,可能会导致错误。
在以下示例中,我们尝试访问不存在的属性 enrYear。这将导致运行时错误,因为我们已将 student 对象定义为 any 类型。请注意,它不会显示编译时错误。
const student: any = { name: "John Doe", age: 32, isEnrolled: true, } console.log(student.name); console.log(student.age); console.log(student.enrYear);
编译后,它将生成以下 JavaScript 代码 -
const student = { name: "John Doe", age: 32, isEnrolled: true, }; console.log(student.name); console.log(student.age); console.log(student.enrYear);
以上示例代码的输出如下 -
John Doe 32 undefined
any vs. unknown
当使用 any 类型声明变量时,您可以访问其不存在的属性。
示例:使用 any 声明的变量
let user: any; user.isEnrolled;
以上 TypeScript 代码在编译时不会显示任何错误。但它会引发以下运行时错误。
Cannot read properties of undefined (reading 'isEnrolled')
示例:使用 unknown 声明的变量
let user:unknown; user.isEnrolled;
以上代码将显示以下编译时错误 -
'user' is of type 'unknown'.