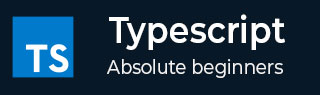
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript 与 JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null 与 undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 类型别名
在 TypeScript 中,类型别名是一种定义类型的方式。它允许你为类型赋予一个特定的名称,或者使用 'type' 关键字定义自定义类型。基本上,你可以对基本类型和非基本类型进行一些更改,并可以使用类型别名定义自定义数据类型。
语法
你可以按照以下语法在 TypeScript 中定义类型别名。
type aliasName = Type;
在上面的代码中,'type' 是一个关键字。'AliasName' 是类型别名的名称。'Type' 可以是基本类型、非基本类型或任何自定义数据类型。
基本类型的别名
类型别名的基本用法是为基本类型创建别名,也就是创建基本类型的副本。例如,在实际应用中,与其直接使用字符串或数字数据类型作为 userID 变量,我们可以创建 userID 别名并将类型存储在其中,以提高代码的可维护性。
示例
在下面的代码中,我们定义了数字类型的 'UserID' 类型别名。'user1' 变量只能包含数字值,因为其类型是 'UserID'。
// Defining the type alias type UserID = number; // Defining the variable of type alias let user1: UserID = 101; console.log(user1);
编译后,它将生成以下 JavaScript 代码。
// Defining the variable of type alias let user1 = 101; console.log(user1);
输出如下:
101
联合类型的别名
当你想定义一个可以包含多种类型值的变量时,可以使用联合类型。例如,如果你正在开发一个接受多种类型输入(如字符串和数字)的函数,使用类型别名可以使函数签名更加清晰。否则,如果在整个代码中重复联合类型,可能会使代码变得复杂。
示例
在下面的代码中,'StringOrNumber' 类型别名包含字符串和数字的联合。logMessage() 函数接受字符串或数字作为参数,因为参数类型是 'StringOrNumber'。
接下来,我们在传递字符串和数字作为参数后执行了该函数。
type StringOrNumber = string | number; function logMessage(message: StringOrNumber): void { console.log(message); } logMessage("Hello"); logMessage(123);
编译后,它将生成以下 JavaScript 代码。
function logMessage(message) { console.log(message); } logMessage("Hello"); logMessage(123);
上面代码的输出如下:
Hello 123
元组的别名
元组别名用于定义固定大小数组的结构,该数组可以按特定顺序包含特定类型的值。
示例
在下面的代码中,我们定义了 'RGBColor' 元组来存储颜色表示的 RGB 值。在这个元组别名中,所有值都是数字。
type RGBColor = [number, number, number]; let red: RGBColor = [255, 0, 0]; console.log(`Red color: ${red}`);
编译后,它将生成以下 JavaScript 代码。
let red = [255, 0, 0]; console.log(`Red color: ${red}`);
上面代码的输出如下:
Red color: 255,0,0
对象类型的别名
对象可以以键值对的形式拥有属性。有时,你需要定义多个具有相同结构的对象,可以使用对象类型别名。通过为对象类型创建别名,你可以重复使用它。
示例
在下面的代码中,我们定义了 'User' 类型,它包含字符串类型的 id 和 name 属性。
'user' 对象包含字符串类型的 id 和 name。
// Defining the type alias for the User object type User = { id: string; name: string; }; // Defining the user object using the User type alias let user: User = { id: "101", name: "Alice" }; console.log(user);
编译后,它将生成以下 JavaScript 代码。
// Defining the user object using the User type alias let user = { id: "101", name: "Alice" }; console.log(user);
上面代码的输出如下:
{ id: '101', name: 'Alice' }
函数类型的别名
在处理高阶函数或回调函数时,函数类型的别名尤其有用,它为函数应该接受和返回的内容提供了一个清晰的契约。
示例
在下面的代码中,'GreeterFunction' 定义了函数的类型。它以字符串作为参数,并返回字符串值。
'greet' 变量存储类型为 'GreeterFunction' 的函数表达式。
// Define a function type type GreeterFunction = (name: string) => string; // Define a function that matches the type const greet: GreeterFunction = name => `Hello, ${name}!`; console.log(greet("TypeScript"));
编译后,它将生成以下 JavaScript 代码。
// Define a function that matches the type const greet = name => `Hello, ${name}!`; console.log(greet("TypeScript"));
输出如下:
Hello, TypeScript!
将类型别名与泛型一起使用
泛型类型用于创建自定义类型。它以 'T' 作为参数,并根据 'T' 类型创建新的类型。
示例
在下面的代码中,'Container' 类型接受 'T' 作为参数,并定义了类型为 'T' 的对象属性值。
之后,在使用 'Container' 类型时,我们将数据类型作为参数传递。对于 'numberContainer' 变量,我们使用了 'number' 类型,对于 'stringContainer' 变量,我们使用了 'string' 数据类型。
// Defining the generic type alias type Container<T> = { value: T }; // Using the generic type alias let numberContainer: Container<number> = { value: 123 }; let stringContainer: Container<string> = { value: "Hello World" }; console.log(numberContainer); // Output: { value: 123 } console.log(stringContainer); // Output: { value: 'Hello World' }
编译后,它将生成以下 JavaScript 代码。
// Using the generic type alias let numberContainer = { value: 123 }; let stringContainer = { value: "Hello World" }; console.log(numberContainer); // Output: { value: 123 } console.log(stringContainer); // Output: { value: 'Hello World' }
上面代码的输出如下:
{ value: 123 } { value: 'Hello World' }
类型别名是定义自定义类型的方式,也允许在定义一次后重复使用复杂类型。它还有助于提高代码可读性并最大程度地降低代码复杂性。