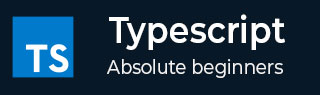
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any 类型
- TypeScript - never 类型
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - Symbol
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策语句
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - Function 构造函数
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - keyof 类型操作符
- TypeScript - typeof 类型操作符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - never 类型
never 类型
TypeScript 中的 never 类型表示永远不会出现的数值。例如,抛出错误的函数的返回类型是 never。
function funcName(): never{ // it throws an exception or never returns }
你不能将值赋给 never 类型的变量 –
let x: never; x = 10; // throw error
上面的 TypeScript 代码片段将抛出以下错误 –
Type 'number' is not assignable to type 'never'.
你可以使用 never 类型作为永远不会返回或总是抛出异常的函数的返回类型。
一个永远不会停止执行的函数 –
function infiniteLoop(): never{ for(;;){} }
另一个永远不会停止执行的函数示例 –
function infiniteLoop(): never{ while (true) { } }
当被任何永远不会为真的类型守卫缩小时,变量也会获得 never 类型。
当我们已经穷尽所有可能的值并且我们没有任何东西可以赋值时,使用 never 类型。
function check(value: string | number) { if (typeof value === 'string') { return "value is string"; } else { return "value is number"; } // here, not a string or number // "value" can't occur here, so it's type "never" }
never 类型是每个类型的子类型,并且可以赋值给每个类型;但是,没有类型是 never 的子类型,或者可以赋值给 never(除了 never 本身)。甚至 any 也不能赋值给 never。
你可以用 never 类型注释变量,但这很不常见 –
function showError(): never{ throw new Error("Error message from function with never as return type."); } let x: never = showError();
在上面的 Typescript 代码片段中,变量 x 用 never 类型注释。变量 x 被赋值 showError() 函数,该函数本身的返回类型为 never。
void vs. never
在 TypeScript 中,void 类型的变量可以存储 undefined 作为值。另一方面,never 不能存储任何值。
let val1: void = undefined; let val2: never = undefined; // error: Type 'undefined' is not assignable to type 'never'.
我们知道,如果 TypeScript 中的函数不返回值,则返回 undefined。我们通常用 void 来注释此类函数的返回类型。看下面的例子,
function greet(): void{ console.log("Welcome to tutorials Point"); } let msg: void = greet(); console.log(msg);
在上面的例子中,我们定义了一个不返回值的函数 greet()。当我们调用该函数时,返回值是 undefined。
编译后,它将生成以下 JavaScript 代码。
function greet() { console.log("Welcome to tutorials Point"); } let msg = greet(); console.log(msg);
上述代码的输出如下:
Undefined
广告