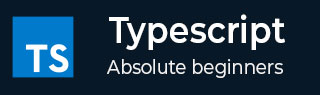
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 存取器
TypeScript 中的存取器提供了一种使用 getter 和 setter 方法访问和设置类成员值的方式。它们控制如何访问类成员以读取或写入其值。
存取器对于在 TypeScript 中实现封装很有用,封装限制了对类成员的访问,仅限于类的成员函数,防止第三方未经授权的访问。
TypeScript 支持以下方法来访问和更改类成员
getter
setter
TypeScript 中的 Getter
Getter 用于访问类成员的值并管理如何在类外部访问这些值。它们可以使用 'get' 关键字创建。
语法
您可以按照以下语法在 TypeScript 中使用 getter。
class class_name { // Define private variable here. // Defining the getter get getter_name(): return_type { // Return variable value } } let val = class_name.getter_name;
在上述语法中,method_name 方法是一个静态方法,可以接受多个参数并返回值。
要使用 getter 访问值,我们可以使用类名后跟一个点,然后是 getter 方法名。
示例
在下面的示例中,我们定义了 Person 类,其中包含 'Name' 私有变量。它还包含 constructor() 方法,该方法初始化 'Name' 变量的值。
// Defining the Person class class Person { // Defining the private field private Name: string; // Defining the constructor constructor(Name: string) { this.Name = Name; } // Defining the getter get SName(): string { return this.Name; } } // Creating an instance of the Person class const person = new Person("John"); console.log(person.SName); // Outputs: John
编译后,它将生成以下 JavaScript 代码。
// Defining the Person class class Person { // Defining the constructor constructor(Name) { this.Name = Name; } // Defining the getter get SName() { return this.Name; } } // Creating an instance of the Person class const person = new Person("John"); console.log(person.SName); // Outputs: John
输出
John
示例
在下面的代码中,Temperature 类包含 'celsius' 私有变量。constructor() 方法初始化 'celsius' 变量的值。
// Define a class Temperature with a property Celsius of type number. class Temperature { private celsius: number; // Define a constructor that initializes the Celsius property. constructor(celsius: number) { this.celsius = celsius; } // Define a getter fahrenheit that returns the temperature in Fahrenheit. get fahrenheit(): number { return (this.celsius * 9 / 5) + 32; } } // Create an instance of the Temperature class with a temperature of 25 degrees Celsius. const temp = new Temperature(25); console.log("The Fahrenheit value is: " + temp.fahrenheit); // Outputs: 77
编译后,它将生成以下 JavaScript 代码。
// Define a class Temperature with a property Celsius of type number. class Temperature { // Define a constructor that initializes the Celsius property. constructor(celsius) { this.celsius = celsius; } // Define a getter fahrenheit that returns the temperature in Fahrenheit. get fahrenheit() { return (this.celsius * 9 / 5) + 32; } } // Create an instance of the Temperature class with a temperature of 25 degrees Celsius. const temp = new Temperature(25); console.log("The Fahrenheit value is: " + temp.fahrenheit); // Outputs: 77
输出
The Fahrenheit value is: 77
TypeScript 中的 Setter
在 TypeScript 中,setter 用于设置类成员的值,而无需在类外部访问它们。它们使用 'set' 关键字来定义 setter 方法。
语法
您可以按照以下语法在 TypeScript 中使用 setter。
class class_name { // Define private variable here. // Defining the setter set setter_name(val: type) { // Set variable value } } class_name.setter_name = val;
在上述语法中,我们使用了 'set' 关键字后跟 'setter_name' 来定义一个 setter。它只接受一个值作为参数,并在 setter 方法内部使用它来更改任何私有类变量的值。
要使用 setter 方法,您需要使用类名后跟一个点,然后是 setter 方法名,并为其赋值。
示例
在下面的代码中,我们定义了 TextContainer 类,其中包含 '_content' 私有变量来存储文本。
// Define a class with a private property and a getter/setter method class TextContainer { // Define a private property private _content: string = ''; // Setter method set content(value: string) { this._content = value.trim().toLowerCase(); } // Getter method get content(): string { return this._content; } } // Create an instance of the class and set the content const text = new TextContainer(); text.content = " Hello, WORLD! "; console.log(text.content); // Outputs: hello, world!
编译后,它将生成以下 JavaScript 代码。
// Define a class with a private property and a getter/setter method class TextContainer { constructor() { // Define a private property this._content = ''; } // Setter method set content(value) { this._content = value.trim().toLowerCase(); } // Getter method get content() { return this._content; } } // Create an instance of the class and set the content const text = new TextContainer(); text.content = " Hello, WORLD! "; console.log(text.content); // Outputs: hello, world!
输出
hello, world!
在 TypeScript 中使用存取器来实现封装非常重要。您还可以在单个类中创建多个 getter 和 setter 方法。