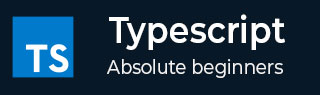
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境搭建
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - any
- TypeScript - never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - if 语句
- TypeScript - if else 语句
- TypeScript - 嵌套 if 语句
- TypeScript - switch 语句
- TypeScript - 循环
- TypeScript - for 循环
- TypeScript - while 循环
- TypeScript - do while 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 接口扩展
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 存取器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型保护
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜杠指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - 日期对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixin
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - let & const
TypeScript声明变量的规则与JavaScript相同。最初,只使用'var'关键字声明变量,但在JavaScript的ES6版本中引入了'let'和'const'关键字来声明变量。因此,你也可以在TypeScript中使用它们。
在本课中,你将学习如何使用'let'和'const'关键字声明变量,以及这些变量与使用'var'关键字声明的变量有何不同。
使用let关键字声明变量
当我们在TypeScript中使用'let'关键字声明变量时,作用域规则和提升规则与JavaScript中的相同。
语法
在TypeScript中使用'let'关键字声明变量的语法如下:
let var_name: var_type = value;
在上例语法中,'let'是一个关键字。
'var_name'是变量名的有效标识符。
'var_type'是变量的类型。
'value'是要存储在变量中的值。
示例
在下面的代码中,我们定义了字符串类型的'car_name'变量,它包含“Brezza”值。'car_price'变量包含数字值1000000。
// Define a variable in TypeScript let car_name: string = "Brezza"; let car_price: number = 1000000; console.log(car_name); console.log(car_price);
编译后,它将生成以下JavaScript代码。
// Define a variable in TypeScript let car_name = "Brezza"; let car_price = 1000000; console.log(car_name); console.log(car_price);
输出
上述示例代码的输出如下:
Brezza 1000000
变量作用域
使用'let'关键字声明的变量具有块级作用域。这意味着你无法像使用'var'关键字声明的变量那样访问块外的变量。
让我们通过下面的例子来学习。
在下面的代码中,'bool'变量包含true值,因此'if'语句的代码将始终执行。在'if'块中,我们声明了'result'变量,只能在'if'块内访问它。如果你尝试在'if'块外访问它,TypeScript编译器将抛出错误。
let bool: boolean = true; if (bool) { let result: number = 10; console.log(result); // It can have accessed only in this block } // console.log(result); Can't access variable outside of the block.
let变量不能重新声明
你不能重新声明使用'let'关键字声明的变量。
让我们看看下面的例子。
在下面的代码中,你可以看到,如果我们尝试重新声明相同的变量,TypeScript编译器将抛出错误。
let animal: string = "cat"; // let animal: string = "dog"; // Error: Cannot redeclare block-scoped variable 'animal' console.log(animal); // Output: cat
编译后,它将生成以下JavaScript代码。
let animal = "cat"; // let animal: string = "dog"; // Error: Cannot redeclare block-scoped variable 'animal' console.log(animal); // Output: cat
上述示例代码的输出如下:
cat
不同块中具有相同名称的变量
使用'let'关键字声明的变量具有块级作用域。因此,如果具有相同名称的变量位于不同的块中,则它们被视为不同的变量。
让我们看看下面的例子。
在下面的代码中,我们在'if'和'else'两个块中声明了'num'变量,并用不同的值初始化它们。
let bool: boolean = false; // If the boolean is true, the variable num will be 1, otherwise it will be 2 if (bool) { let num: number = 1; console.log(num); } else { let num: number = 2; console.log(num); }
编译后,它将生成以下JavaScript代码。
let bool = false; // If the boolean is true, the variable num will be 1, otherwise it will be 2 if (bool) { let num = 1; console.log(num); } else { let num = 2; console.log(num); }
上述示例代码的输出如下:
2
使用'const'关键字声明变量
'const'关键字与'var'和'let'具有相同的语法来声明变量。它用于声明常量变量。此外,你需要在定义'const'变量时初始化它们,并且以后不能更改它们。
语法
在TypeScript中使用'const'关键字声明变量的语法如下:
const var_name: var_type = value;
在上例语法中,'const'是一个关键字。
示例
在下面的代码中,我们使用'const'关键字定义了'lang'和'PI'变量,它们分别包含'TypeScript'和'3.14'值。
// Define a constant variable in TypeScript const lang: string = 'TypeScript'; const PI: number = 3.14; console.log(`Language: ${lang}`); console.log(`Value of PI: ${PI}`);
编译后,它将生成以下JavaScript代码。
// Define a constant variable in TypeScript const lang = 'TypeScript'; const PI = 3.14; console.log(`Language: ${lang}`); console.log(`Value of PI: ${PI}`);
上述示例代码的输出如下:
Language: TypeScript Value of PI: 3.14
使用'const'关键字声明的变量在作用域和重新声明方面与使用'let'关键字声明的变量具有相同的规则。
在下面的代码中,你可以看到,如果你尝试在相同的作用域中重新声明'const'变量,或者尝试在声明后更改它的值,它都会抛出错误。
if (true) { const PI: number = 3.14; console.log(PI); // const PI: number = 3.14; Error: Cannot redeclare block-scoped variable 'PI'. // PI = 3.15; Error: Cannot assign to 'PI' because it is a constant. }
编译后,它将生成以下JavaScript代码。
if (true) { const PI = 3.14; console.log(PI); // const PI: number = 3.14; Error: Cannot redeclare block-scoped variable 'PI'. // PI = 3.15; Error: Cannot assign to 'PI' because it is a constant. }
上述示例代码的输出如下:
3.14
你学习了如何使用'let'和'const'语句声明变量。由于其块级作用域特性,避免了在不同作用域中声明的变量的值被覆盖,因此始终建议使用'let'关键字声明变量。