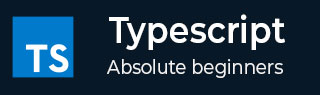
- TypeScript 基础
- TypeScript - 首页
- TypeScript - 路线图
- TypeScript - 概述
- TypeScript - 环境设置
- TypeScript - 基本语法
- TypeScript vs. JavaScript
- TypeScript - 特性
- TypeScript - 变量
- TypeScript - let & const
- TypeScript - 运算符
- TypeScript 基本类型
- TypeScript - 类型
- TypeScript - 类型注解
- TypeScript - 类型推断
- TypeScript - 数字
- TypeScript - 字符串
- TypeScript - 布尔值
- TypeScript - 数组
- TypeScript - 元组
- TypeScript - 枚举
- TypeScript - Any
- TypeScript - Never
- TypeScript - 联合类型
- TypeScript - 字面量类型
- TypeScript - 符号
- TypeScript - null vs. undefined
- TypeScript - 类型别名
- TypeScript 控制流
- TypeScript - 决策
- TypeScript - If 语句
- TypeScript - If Else 语句
- TypeScript - 嵌套 If 语句
- TypeScript - Switch 语句
- TypeScript - 循环
- TypeScript - For 循环
- TypeScript - While 循环
- TypeScript - Do While 循环
- TypeScript 函数
- TypeScript - 函数
- TypeScript - 函数类型
- TypeScript - 可选参数
- TypeScript - 默认参数
- TypeScript - 匿名函数
- TypeScript - 函数构造器
- TypeScript - Rest 参数
- TypeScript - 参数解构
- TypeScript - 箭头函数
- TypeScript 接口
- TypeScript - 接口
- TypeScript - 扩展接口
- TypeScript 类和对象
- TypeScript - 类
- TypeScript - 对象
- TypeScript - 访问修饰符
- TypeScript - 只读属性
- TypeScript - 继承
- TypeScript - 静态方法和属性
- TypeScript - 抽象类
- TypeScript - 访问器
- TypeScript - 鸭子类型
- TypeScript 高级类型
- TypeScript - 交叉类型
- TypeScript - 类型守卫
- TypeScript - 类型断言
- TypeScript 类型操作
- TypeScript - 从类型创建类型
- TypeScript - Keyof 类型运算符
- TypeScript - Typeof 类型运算符
- TypeScript - 索引访问类型
- TypeScript - 条件类型
- TypeScript - 映射类型
- TypeScript - 模板字面量类型
- TypeScript 泛型
- TypeScript - 泛型
- TypeScript - 泛型约束
- TypeScript - 泛型接口
- TypeScript - 泛型类
- TypeScript 其他
- TypeScript - 三斜线指令
- TypeScript - 命名空间
- TypeScript - 模块
- TypeScript - 环境声明
- TypeScript - 装饰器
- TypeScript - 类型兼容性
- TypeScript - Date 对象
- TypeScript - 迭代器和生成器
- TypeScript - Mixins
- TypeScript - 实用程序类型
- TypeScript - 装箱和拆箱
- TypeScript - tsconfig.json
- 从 JavaScript 到 TypeScript
- TypeScript 有用资源
- TypeScript - 快速指南
- TypeScript - 有用资源
- TypeScript - 讨论
TypeScript - 箭头函数
箭头函数是 TypeScript 中编写匿名函数的简洁方式。它们在 ES6(ECMAScript 2015)中引入。与传统的函数声明相比,它们提供了更短、更易读的语法。箭头函数也称为 lambda 函数。Lambda 指的是编程中的匿名函数。
语法
您可以使用胖箭头(=>)定义箭头函数。胖箭头用于分隔函数参数和函数体(语句)。
(param1, param2, ..., paramN) => statement
箭头函数有三个部分:
参数 - 函数可以选择具有参数,param1、param2、...、paramN。
胖箭头表示法/lambda 表示法(=>) - 它也称为“转到”运算符。
语句 - 表示函数的指令集
示例
让我们通过一些 TypeScript 中的示例来了解箭头函数/lambda 函数:
示例:具有单个语句的箭头函数
我们可以按如下方式编写具有单个语句的箭头函数:
(x: number, y: number): number => x +y;
对于单个语句,可以选择不写 return 关键字来返回值和括号(花括号)。但如果使用括号,则需要编写 return 关键字才能返回值。
(x: number, y: number): number => {return x +y;}
让我们看下面的例子:
const add = (x: number, y: number): number => x +y; console.log(add(20,30));
编译后,它将生成以下 JavaScript 代码。
const add = (x, y) => x + y; console.log(add(20, 30));
它将产生以下结果:
50
示例:具有多个语句的箭头函数
您可以使用花括号编写多个语句。您还需要编写 return 语句才能返回值。
在下面的示例中,我们定义了一个接受两个参数并返回其乘积的箭头函数。
const multiply = (x: number, y: number): number => { let res: number = x * y; return res; }; console.log(multiply(5,6));
编译后,它将生成以下 JavaScript 代码。
const multiply = (x, y) => { let res = x * y; return res; }; console.log(multiply(5, 6));
它将产生以下输出:
30
示例:没有参数的箭头函数
箭头函数的参数是可选的。
在下面的示例中,我们定义了一个不带参数并返回字符串值的箭头函数。
const greet = () => { return "Hello World!";} console.log(greet());
编译后,它将生成相同的 JavaScript 代码。
上面代码示例的输出如下:
Hello World!
示例:具有单个参数的箭头函数
以下示例显示了一个接受单个参数并返回该参数与 10 的和的箭头函数。
const add = (x: number): number => {return x + 10;} console.log(add(5));
编译后,它将生成以下 JavaScript 代码。
const add = (x) => { return x + 10; }; console.log(add(5));
上面代码示例的输出如下:
15
示例:具有多个参数的箭头函数
在下面的示例中,我们定义了一个接受三个参数并返回其和的箭头函数。
let sum: number; const add = (a: number, b: number, c: number) => { sum = a + b + c; return sum; }; let res = add(10, 30, 45); console.log("The result is: " + res);
编译后,它将生成以下 JavaScript 代码。
let sum; const add = (a, b, c) => { sum = a + b + c; return sum; }; let res = add(10, 30, 45); console.log("The result is: " + res);
上面代码示例的输出如下:
The result is: 85
示例:具有默认参数的箭头函数
在下面的示例中,我们将参数的默认值传递给箭头函数。当您不传递相应的参数值时,这些默认值将在函数体内部使用。
const mul = (a = 10, b = 15) => a * b; console.log("mul(5, 8) = " + mul(5, 8)); console.log("mul(6) = " + mul(6)); console.log("mul() = " + mul());
编译后,它将生成相同的 JavaScript 代码。
上面代码示例的输出如下:
mul(5, 8) = 40 mul(6) = 90 mul() = 150
箭头函数的应用
现在让我们讨论一些 TypeScript 中箭头函数的应用示例。
示例:箭头函数作为其他函数中的回调函数
箭头函数(Lambda 表达式)也可用作函数中的回调,例如数组方法(例如,map、filter、reduce)。以下是一个演示它们与“map”方法一起使用的示例:
const numbers = [1, 2, 3, 4, 5]; const squaredNumbers = numbers.map(x => x * x); console.log(`Original array: ${numbers}`); console.log(`Squared array: ${squaredNumbers}`);
编译后,它将生成相同的 JavaScript 代码。
上面代码示例的输出如下:
Original array: 1,2,3,4,5 Squared array: 1,4,9,16,25
示例:类中的箭头函数
箭头函数(Lambda 表达式)可以用作 TypeScript 中的类方法或对象属性。以下是一个包含作为 lambda 表达式组成的方法的类的示例:
class Calculator { add = (x: number, y: number, z: number): number => x + y + z; } const obj = new Calculator(); console.log(`The result is: ${obj.add(4, 3, 2)}`);
编译后,它将生成以下 JavaScript 代码。
class Calculator { constructor() { this.add = (x, y, z) => x + y + z; } } const obj = new Calculator(); console.log(`The result is: ${obj.add(4, 3, 2)}`);
它将产生以下输出:
The result is: 9
示例:实现高阶函数
高阶函数(接受一个或多个函数作为参数和/或返回一个函数作为结果的函数)可以使用 TypeScript 中的 lambda 表达式方便地定义。
const applyOp = ( x: number, y: number, operation: (a: number, b: number) => number ) => { return operation(x, y); }; const result1 = applyOp(10, 20, (a, b) => a + b); console.log(result1); const result2 = applyOp(10, 20, (a, b) => a * b); console.log(result2);
编译后,它将生成以下 JavaScript 代码。
const applyOp = (x, y, operation) => { return operation(x, y); }; const result1 = applyOp(10, 20, (a, b) => a + b); console.log(result1); const result2 = applyOp(10, 20, (a, b) => a * b); console.log(result2);
上面代码示例的输出如下:
30 200
箭头函数在编写匿名函数方面非常有用。