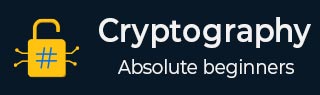
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代密码学
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力攻击
- 密码系统 - 密码分析技术
- 密码学类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥吊销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel分组密码
- 分组密码的工作模式
- 分组密码的工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13算法
- 密码学 - 置换密码
- 密码学 - 加密置换密码
- 密码学 - 解密置换密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码加密
- 密码学 - 简单替换密码解密
- 密码学 - 维吉尼亚密码
- 密码学 - 维吉尼亚密码的实现
- 现代密码
- Base64编码与解码
- 密码学 - XOR加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 单字母替换密码的破解
- 密码学 - 多字母替换密码
- 密码学 - Playfair密码
- 密码学 - Hill密码
- 多字母替换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 置换技术
- 密码学 - 栅栏密码
- 密码学 - 列置换密码
- 密码学 -隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准 (DES)
- 密码学 - 三重DES
- 密码学 - 双重DES
- 高级加密标准 (AES)
- 密码学 - AES结构
- 密码学 - AES变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混淆变换
- 密码学 - 轮密钥加变换
- 密码学 - AES密钥扩展算法
- 密码学 - Blowfish算法
- 密码学 - SHA算法
- 密码学 - RC4算法
- 密码学 - Camellia加密算法
- 密码学 - ChaCha20加密算法
- 密码学 - CAST5加密算法
- 密码学 - SEED加密算法
- 密码学 - SM4加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码算法
- 密码学 - RSA算法
- 密码学 - RSA加密
- 密码学 - RSA解密
- 密码学 - 创建RSA密钥
- 密码学 - 破解RSA密码
- 密码学 - ECDSA算法
- 密码学 - DSA算法
- 密码学 - Diffie-Hellman算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施 (PKI)
- 哈希
- MD5(消息摘要算法5)
- SHA-1(安全哈希算法1)
- SHA-256(安全哈希算法256位)
- SHA-512(安全哈希算法512位)
- SHA-3(安全哈希算法3)
- 哈希密码
- Bcrypt哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码协议
- 密码学 - SSL/TLS协议
- 密码学 - SSH协议
- 密码学 - IPsec协议
- 密码学 - PGP协议
- 图像和文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用的密码技术
- 自定义构建密码算法(混合密码学)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 vs SHA1
- 密码学 - RSA vs DSA
- 密码学 - RSA vs Diffie-Hellman
- 密码学 vs 密码学
- 密码学 - 密码学 vs 密码分析
- 密码学 - 经典 vs 量子
- 密码学 vs 隐写术
- 密码学 vs 加密
- 密码学 vs 网络安全
- 密码学 - 流密码 vs 分组密码
- 密码学 - AES vs DES 密码
- 密码学 - 对称 vs 非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - 文件解密
文件解密是一种解密方法,它将文件转换回明文或可读数据。使用此方法可确保授权人员可以访问您的数据,他们可以使用解密密钥读取内容。
在本章中,我们将看到不同的解密技术来解密文件数据。让我们深入探讨。
文件解密的基本方法
解密是将加密数据更改回其原始可读形式的过程。以下是文件解密的一些基本方法:
对称密钥解密
单个密钥用于加密和解密。要解密文件,请使用与加密文件时相同的密钥。解密过程通常涉及使用解密密钥将加密算法的逆运算添加到加密数据中。
from cryptography.fernet import Fernet # Generate a key key = Fernet.generate_key() cipher_suite = Fernet(key) # Read the file with open('plain_text.txt', 'rb') as f: plaintext = f.read() # Encrypt the file encrypted_text = cipher_suite.encrypt(plaintext) # Write the encrypted file with open('encrypted_file.txt', 'wb') as f: f.write(encrypted_text) # Print message after file is encrypted print("File encrypted successfully.") # Decrypt the file decrypted_text = cipher_suite.decrypt(encrypted_text) # Write the decrypted file with open('decrypted_file.txt', 'wb') as f: f.write(decrypted_text) # Print message after file is decrypted print("File decrypted successfully.")
输出
File encrypted successfully. File decrypted successfully.
请参见下面的输出图像,其中显示了plain_text.txt、encrypted_file.txt和decrypted_file.txt文件。
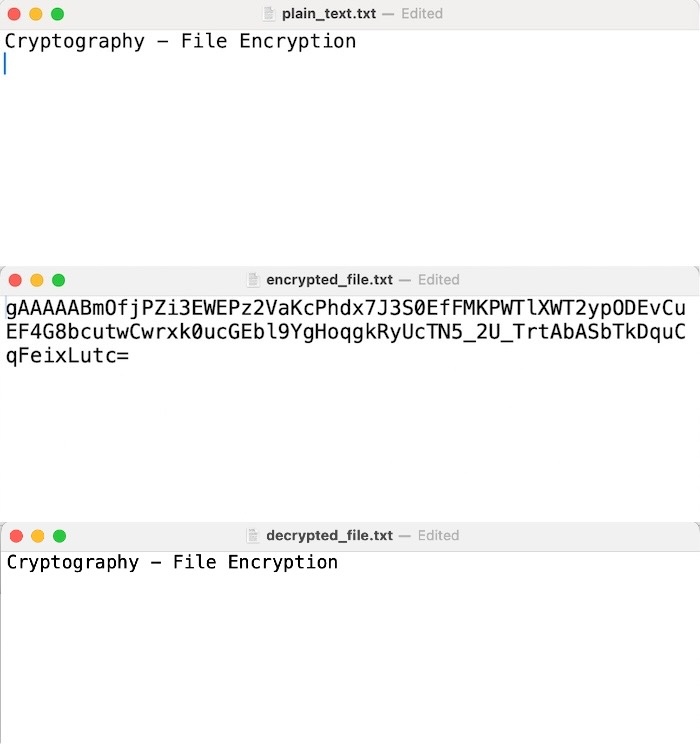
非对称密钥解密
非对称密钥加密需要两个密钥:公钥和私钥。公钥用于加密,私钥用于解密。要解密非对称加密的文件,您需要私钥。非对称加密通常用于安全通信和密钥交换。
from cryptography.hazmat.primitives import serialization from cryptography.hazmat.primitives.asymmetric import rsa, padding from cryptography.hazmat.primitives import hashes # Generate key pair private_key = rsa.generate_private_key( public_exponent=65537, key_size=2048 ) public_key = private_key.public_key() # Save private key with open("private.pem", "wb") as f: f.write( private_key.private_bytes( encoding=serialization.Encoding.PEM, format=serialization.PrivateFormat.TraditionalOpenSSL, encryption_algorithm=serialization.NoEncryption() ) ) # Save public key with open("public.pem", "wb") as f: f.write( public_key.public_bytes( encoding=serialization.Encoding.PEM, format=serialization.PublicFormat.SubjectPublicKeyInfo ) ) def encrypt_file(file_path, public_key_path, output_path): # Load public key with open(public_key_path, "rb") as f: public_key = serialization.load_pem_public_key(f.read()) # Encrypt file with open(file_path, "rb") as f: plaintext = f.read() ciphertext = public_key.encrypt( plaintext, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) # Save encrypted file with open(output_path, "wb") as f: f.write(ciphertext) def decrypt_file(file_path, private_key_path, output_path): # Load private key with open(private_key_path, "rb") as f: private_key = serialization.load_pem_private_key( f.read(), password=None ) # Decrypt file with open(file_path, "rb") as f: ciphertext = f.read() plaintext = private_key.decrypt( ciphertext, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) # Save decrypted file with open(output_path, "wb") as f: f.write(plaintext) # Encrypt file encrypt_file("plain_text.txt", "public.pem", "encrypted_file.bin") # Decrypt file decrypt_file("encrypted_file.bin", "private.pem", "decrypted_plaintext.txt")
输出
使用“python program.py”运行上述代码后,它将创建公钥和私钥,然后使用公钥加密名为plain_text.txt的文件,然后使用私钥解密加密的文件。
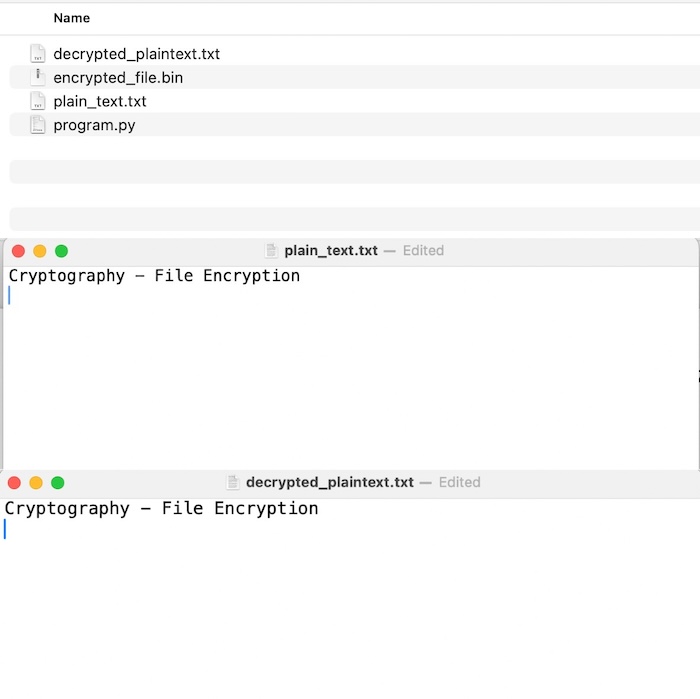
基于密码的解密
密码或密码短语会创建一个用于加密和解密的密钥。相同的密码用于加密和解密数据。要解密受基于密码的加密保护的文件,您必须输入正确的密码。
from cryptography.fernet import Fernet # get password from user password = input("Enter password: ").encode() # derive key from password key = Fernet.generate_key() # create Fernet cipher suite with the derived key cipher_suite = Fernet(key) # read the encrypted file with open('encrypted_file.txt', 'rb') as f: encrypted_text = f.read() # decrypt the file try: decrypted_text = cipher_suite.decrypt(encrypted_text) # write the decrypted file with open('decrypted_file.txt', 'wb') as f: f.write(decrypted_text) print("File decrypted successfully.") except Exception as e: print("Error decrypting file:", str(e))
输入/输出
Enter password: 12345 Error decrypting file:
密钥派生
某些加密方法使用密钥派生函数 (KDF) 从密码或密码短语生成密钥。然后使用生成的密钥进行加密和解密。密钥派生确保使用相同的密码创建相同的密钥,从而允许安全解密。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
初始化向量 (IV) 的使用
初始化向量 (IV) 用于加密算法中,以防止密文与明文相同。解密文件时,通常需要同时提供 IV 和解密密钥。IV 通常包含在加密文件中或与其一起发送。