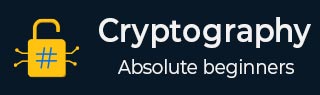
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组件
- 密码系统攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码操作模式
- 分组密码操作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 换位密码
- 密码学 - 加密换位密码
- 密码学 - 解密换位密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码加密
- 密码学 - 简单替换密码解密
- 密码学 - 维吉尼亚密码
- 密码学 - 实现维吉尼亚密码
- 现代密码
- Base64 编码与解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 破解单字母替换密码
- 密码学 - 多字母替换密码
- 密码学 - Playfair 密码
- 密码学 - 希尔密码
- 多字母替换密码
- 密码学 - 一次性密码本
- 一次性密码本的实现
- 密码学 - 换位技术
- 密码学 - 栅栏密码
- 密码学 - 列移位密码
- 密码学 - 隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准
- 密码学 - 三重DES
- 密码学 - 双重DES
- 高级加密标准
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混合变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码学算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施
- 散列
- MD5(消息摘要算法 5)
- SHA-1(安全散列算法 1)
- SHA-256(安全散列算法 256 位)
- SHA-512(安全散列算法 512 位)
- SHA-3(安全散列算法 3)
- 散列密码
- Bcrypt 散列模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码学协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像与文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用加密技术
- 自定义构建加密算法(混合加密)
- 云加密
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - 反向密码
什么是反向密码?
可以使用反向密码算法通过反向打印来加密消息。因此,我们可以将“Hi this is Robot”加密为“toboR is siht iH”。如果要解码,只需反转反转后的消息即可获得原始消息。加密和解密的过程相同。
因此,我们可以看到,反向密码是一种非常弱的密码。从密文中可以看出它只是反向顺序。
但是,我们将利用反向密码程序作为我们的初始加密程序,因为它的代码易于理解。

算法
以下是加密和解密的反向密码算法:
加密算法
明文消息将是加密技术的输入,密文消息将是输出。因此,步骤如下:
步骤 1:以明文消息作为输入。
步骤 2:然后反转明文消息中的字符顺序以创建密文消息。
步骤 3:返回密文消息作为输出。
解密算法
此外,密文消息将是解密方法的输入,明文消息将是结果。因此,此算法的步骤如下:
步骤 1:以密文消息作为输入。
步骤 2:然后反转密文消息中的字符顺序以获取原始明文消息。
步骤 3:最后返回明文消息作为输出。
示例
反向密码是最基本的密码类型之一。可以通过简单地反转明文的字符顺序来对其进行加密。密文是结果。例如,反向密码用于将消息“HAT”加密为“TAH”。使用反向密码,消息“HELLO WORLD”被加密为“DLROW OLLEH”。加密和解码消息所使用的方法之间的唯一区别是反转序列。因此,消息“TAH”将被解码为“HAT”。解密消息“DLROW OLLEH”将是“HELLO WORLD”。
反向密码的优势在于它易于使用和理解。孩子们可以使用它向朋友发送“秘密信息”。如果加密的目的是使我们的通信足够安全以至于第三方无法读取,那么反向密码的性能不佳,因为它是一种易于破解的简单密码。
Explore our latest online courses and learn new skills at your own pace. Enroll and become a certified expert to boost your career.
实现
因此,我们可以通过使用 Python 的不同模块和函数以不同的方式实现此算法。让我们在下面的部分中一一了解这些方法:
使用 Python
使用 C++
使用 Java
使用 Python
由于我们必须使用 Python 实现反向密码算法,因此我们将使用 Python 的字符串操作功能。我们将使用切片在函数内部反转给定的明文消息。
表达式 message[::-1] 中使用了 Python 的切片功能来更改消息的字符顺序。
在 Python 中,可以通过使用符号 [::-1] 来反转字符串,这意味着从字符串的末尾开始,以 -1 的步长向后移动。
以下是使用 Python 的 str 操作实现反向密码:
示例
def reverse_cipher(message): # Reverse the message using slicing reversed_message = message[::-1] return reversed_message # function execution message = "Hiii! this is a reverse cipher algorithm" encrypted_message = reverse_cipher(message) print("The Original Message: ", message) print("The Encrypted message:", encrypted_message)
以下是上述示例的输出:
输入/输出
The Original Message: Hiii! this is a reverse cipher algorithm The Encrypted message: mhtirogla rehpic esrever a si siht !iiiH
使用 C++
此代码是用 C++ 实现的,基本上展示了反向密码的工作原理。它首先定义密钥消息。之后,它使用 encrypt 函数对消息进行加密,该函数反转消息的行为,并将结果存储在 encryptedMessage 中。然后,使用 decrypt 函数对加密的消息进行解密,该函数将字符恢复到其原始形式,并将结果记录在 decryptedMessage 中。为了展示如何使用反向密码方法进行加密和解密,它最后显示了原始消息、加密的消息和解密的消息。
以下是使用 C++ 实现反向密码:
示例
#include <iostream> #include <string> std::string encrypt(std::string plaintext) { std::string ciphertext = ""; for (int i = plaintext.length() - 1; i >= 0; i--) { ciphertext += plaintext[i]; } return ciphertext; } std::string decrypt(std::string ciphertext) { std::string plaintext = ""; for (int i = ciphertext.length() - 1; i >= 0; i--) { plaintext += ciphertext[i]; } return plaintext; } int main() { std::string message = "Hello, This is a Reverse Cipher example!"; std::string encryptedMessage = encrypt(message); std::string decryptedMessage = decrypt(encryptedMessage); std::cout << "Our Original Message: " << message << std::endl; std::cout << "The Encrypted message: " << encryptedMessage << std::endl; std::cout << "The Decrypted message: " << decryptedMessage << std::endl; return 0; }
以下是上述示例的输出:
输入/输出
Our Original Message: Hello, This is a Reverse Cipher example! The Encrypted message: !elpmaxe rehpiC esreveR a si sihT ,olleH The Decrypted message: Hello, This is a Reverse Cipher example!
使用 Java
提供的 Java 代码的主要功能是使用 ReverseCipher 类对消息进行加密并实现反向密码方法。它首先解释主要消息“Hello, Tutorialspoint!”。然后,它使用 encrypt 方法对消息进行加密,该方法转换消息。然后,它解析给定的消息,将字符重定向回原始消息。最后,它将原始消息、加密的消息和解密的消息打印到控制台,显示加密和解密过程。
以下是使用 Java 实现反向密码:
示例
// Class for reverse cipher public class ReverseCipher { public static String encrypt(String plaintext) { StringBuilder ciphertext = new StringBuilder(); for (int i = plaintext.length() - 1; i >= 0; i--) { ciphertext.append(plaintext.charAt(i)); } return ciphertext.toString(); } public static String decrypt(String ciphertext) { StringBuilder plaintext = new StringBuilder(); for (int i = ciphertext.length() - 1; i >= 0; i--) { plaintext.append(ciphertext.charAt(i)); } return plaintext.toString(); } public static void main(String[] args) { String message = "Hello, Tutorialspoint!"; String encryptedMsg = encrypt(message); String decryptedMsg = decrypt(encryptedMsg); System.out.println("The Original message is: " + message); System.out.println("The Encrypted message is: " + encryptedMsg); System.out.println("The Decrypted message is: " + decryptedMsg); } }
以下是上述示例的输出:
输入/输出
The Original message is: Hello, Tutorialspoint! The Encrypted message is: !tniopslairotuT ,olleH The Decrypted message is: Hello, Tutorialspoint!
特征
以下是反向密码算法的一些特征:
反向密码用于根据给定的模式反转明文字符串以将其转换为密文。
加密和解密的工作方式相同。
在解密过程中,用户只需要反转密文即可获得明文。
缺点
反向密码算法最大的缺点是它非常弱。因此,黑客可以轻松破解密文以了解实际或原始消息。因此,反向密码不被认为是维护安全通信通道的良好选择。
因此,反向密码可用于教育目的或非常基本的加密需求。并且此算法不适合保护机密信息或通信通道,因为它很容易受到攻击。总结
总体而言,反向密码是一种非常简单的密码算法或方法,借助它,消息的字符会被反转以创建密文。因为它易于理解和实现。在安全性方面,它也非常弱。加密和解密都具有相同的文本反转过程。
但是,此算法不是保护非常敏感信息的可靠方法。因为它很容易破解和攻击,这意味着它非常容易受到攻击。因此,它可以用于教育目的。