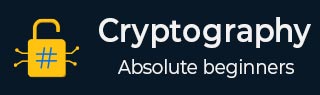
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统的攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学的类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码的工作模式
- 分组密码的工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 换位密码
- 密码学 - 加密换位密码
- 密码学 - 解密换位密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码的加密
- 密码学 - 简单替换密码的解密
- 密码学 - 维吉尼亚密码
- 密码学 - 维吉尼亚密码的实现
- 现代密码
- Base64 编码与解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 单字母替换密码的破解
- 密码学 - 多字母替换密码
- 密码学 - Playfair 密码
- 密码学 - 希尔密码
- 多字母替换密码
- 密码学 - 一次性密码本
- 一次性密码本的实现
- 密码学 - 换位技术
- 密码学 - 栅栏密码
- 密码学 - 列置换密码
- 密码学 - 隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准
- 密码学 - 三重DES
- 密码学 - 双重DES
- 高级加密标准
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混淆变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码学算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施
- 哈希
- MD5(消息摘要算法 5)
- SHA-1(安全哈希算法 1)
- SHA-256(安全哈希算法 256 位)
- SHA-512(安全哈希算法 512 位)
- SHA-3(安全哈希算法 3)
- 哈希密码
- Bcrypt 哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码学协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像与文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用的密码学技术
- 自定义构建密码学算法(混合密码学)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 之间的区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - ROT13 算法
到目前为止,您已经了解了反向密码和凯撒密码算法。现在,让我们讨论 ROT13 算法及其实现。
因此,我们将讨论 ROT13 密码算法。旋转 13 个位置被称为 ROT13。它是凯撒密码加密算法的一种特殊情况。它是最简单的加密方法之一。由于加密和解密的算法相同,因此它是一种对称密钥加密技术。
因为加密密钥将字母 A 到 Z 显示为数字 0 到 25,所以密文与明文字母相差 13 个空格。例如,A 变为 N,B 变为 O,依此类推。由于加密和解密过程相同,因此它们可以以编程方式完成。
ROT13 算法的解释
ROT13 密码指的是缩写形式旋转 13 个位置。在此特定凯撒密码中,移位始终为 13。通过将每个字母移动 13 个位置来加密或解密消息。
让我们查看下图以了解 ROT13 算法的工作原理 -
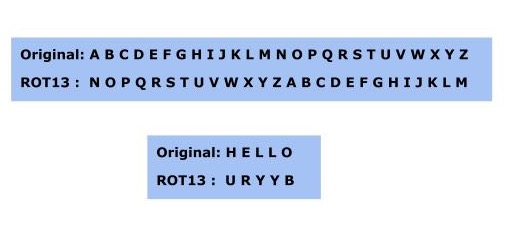
您可以看到,每个字母都向右移动了 13 个空格。例如,“B”将变为“O”,“D”将变为“Q”,依此类推。
ROT13 的特点
以下是 ROT13 的一些主要特征 -
由于 ROT13 是对称的,因此加密和解密都使用相同的算法和密钥。
它适用于每个字母,将每个字母替换为字母表中向前(或向后,基于解密)13 个位置的字母。
ROT13 中的旋转值为 13 是固定的。
应用 ROT13 时,如果旋转超过“Z”,则返回到字母表的开头“A”。
ROT13 非常易于实现和使用。
它无法抵御任何有决心的攻击者,因为它很容易通过简单的频率分析破解。
使用 Python 实现
因此,我们可以通过不同的方式实现此算法 -
使用 For 循环和 ord() 函数
我们首先将给定的消息加密并使用 for 循环生成 ROT13 代码。在此程序中,for 循环将用于迭代给定文本中的每个字符。如果字符是字母,我们将保留它(大写或小写)并将其移动 13 个位置。非字母字符将保持不变。我们还将使用 Python 的 ord() 函数,该函数用于将单个 Unicode 字符更改为其整数表示。
示例
以下是 ROT13 密码的简单 Python 代码。请参阅以下代码 -
# Encryption Function def rot13_encrypt(text): encrypted_text = '' for char in text: if char.isalpha(): shifted = ord(char) + 13 if char.islower(): if shifted > ord('z'): shifted -= 26 else: if shifted > ord('Z'): shifted -= 26 encrypted_text += chr(shifted) else: encrypted_text += char return encrypted_text # Decryption Function def rot13_decrypt(text): decrypted_text = '' for char in text: if char.isalpha(): shifted = ord(char) - 13 # Decryption involves shifting back by 13 if char.islower(): if shifted < ord('a'): shifted += 26 else: if shifted < ord('A'): shifted += 26 decrypted_text += chr(shifted) else: decrypted_text += char return decrypted_text # function execution message = "Hello, Tutorialspoint!" encrypted_msg = rot13_encrypt(message) print("The Encrypted message:", encrypted_msg) decrypted_msg = rot13_decrypt( encrypted_msg) print("The Decrypted message:", decrypted_msg)
以下是上述示例的输出 -
输入/输出
The Encrypted message: Uryyb, Ghgbevnyfcbvag! The Decrypted message: Hello, Tutorialspoint!
使用列表推导式
在此示例中,列表推导式用于执行 ROT13 加密。使用列表,我们将使用末尾的 for char in text 部分迭代输入文本中的每个字符。对于输入文本中的每个字符,我们将使用条件表达式查找加密值。
示例
以下是 ROT13 算法的简单 Python 代码。请参阅以下程序 -
# Encryption function def rot13_encrypt(text): encrypted_text = ''.join([chr(((ord(char) - 65 + 13) % 26) + 65) if 'A' <= char <= 'Z' else chr(((ord(char) - 97 + 13) % 26) + 97) if 'a' <= char <= 'z' else char for char in text]) return encrypted_text # Decryption function def rot13_decrypt(text): decrypted_text = ''.join([chr(((ord(char) - 65 - 13) % 26) + 65) if 'A' <= char <= 'Z' else chr(((ord(char) - 97 - 13) % 26) + 97) if 'a' <= char <= 'z' else char for char in text]) return decrypted_text # Function execution message = "Hello, Everyone!" encrypted_msg = rot13_encrypt(message) print("The Encrypted message:", encrypted_msg) decrypted_msg = rot13_decrypt( encrypted_msg) print("The Decrypted message:", decrypted_msg)
以下是上述示例的输出 -
输入/输出
The Encrypted message: Uryyb, Rirelbar! The Decrypted message: Hello, Everyone!
使用字典
在此示例中,我们将使用两个字典来实现 ROT13 的程序。因此,第一个字典将字母表中的大写字母映射到它们在字母表中的索引。第二个字典将移位的索引映射回大写字母,从“Z”到“A”。加密函数在使用第一个字典识别每个字母的索引并添加移位值后,使用第二个字典将结果索引发送回字母。在解密函数中,我们将反转此过程。
示例
以下是用两个字典实现的 ROT13 算法的简单 Python 程序。查看以下代码 -
# Dictionary to lookup the index dictionary1 = {'A': 1, 'B': 2, 'C': 3, 'D': 4, 'E': 5, 'F': 6, 'G': 7, 'H': 8, 'I': 9, 'J': 10, 'K': 11, 'L': 12, 'M': 13, 'N': 14, 'O': 15, 'P': 16, 'Q': 17, 'R': 18, 'S': 19, 'T': 20, 'U': 21, 'V': 22, 'W': 23, 'X': 24, 'Y': 25, 'Z': 26} # Dictionary to lookup alphabets dictionary2 = {0: 'Z', 1: 'A', 2: 'B', 3: 'C', 4: 'D', 5: 'E', 6: 'F', 7: 'G', 8: 'H', 9: 'I', 10: 'J', 11: 'K', 12: 'L', 13: 'M', 14: 'N', 15: 'O', 16: 'P', 17: 'Q', 18: 'R', 19: 'S', 20: 'T', 21: 'U', 22: 'V', 23: 'W', 24: 'X', 25: 'Y'} # Encryption Function def encrypt(msg, shift): cipher = '' for letter in msg: # check for space if letter != ' ': num = (dictionary1[letter] + shift) % 26 cipher += dictionary2[num] else: cipher += ' ' return cipher # Decryption Function def decrypt(msg, shift): decipher = '' for letter in msg: # checks for space if letter != ' ': num = (dictionary1[letter] - shift + 26) % 26 decipher += dictionary2[num] else: decipher += ' ' return decipher msg = "Hey Tutorialspoint" shift = 13 result = encrypt(msg.upper(), shift) print("The Encrypted message: ", result) msg = "URL GHGBEVNYFCBVAG" shift = 13 result = decrypt(msg.upper(), shift) print("The Decrypted message: ", result)
以下是上述示例的输出 -
输入/输出
The Encrypted message: URL GHGBEVNYFCBVAG The Decrypted message: HEY TUTORIALSPOINT
使用 C++ 实现
为了实现 ROT13 算法,我们将使用 C++ 编程语言。使用 rot13Func 函数,我们将加密给定的字符串消息。迭代给定输入文本中的每个字母。如果它是字母,则将其字符更改 13 个位置以获取匹配的 ROT13 字符。主函数调用 rot13Func 函数并输出加密文本。
示例
#include <iostream> #include <string> using namespace std; // Function to perform rot13Func encryption string rot13Func(string text) { for (char& c : text) { if (isalpha(c)) { char base = islower(c) ? 'a' : 'A'; c = (c - base + 13) % 26 + base; } } return text; } int main() { string plaintext = "Hello this world is so beautiful"; cout << "The Plaintext Message is: " << plaintext << endl; string encrypted_text = rot13Func(plaintext); cout << "Encrypted text: " << encrypted_text << endl; return 0; }
以下是上述示例的输出 -
输入/输出
The Plaintext Message is: Hello this world is so beautiful Encrypted text: Uryyb guvf jbeyq vf fb ornhgvshy
使用 Java 实现
在此实现中,我们将使用 Java 编程语言来创建 ROT13 算法。rot13Func 方法使用字符串文本作为输入,使用 ROT13 对其进行加密。迭代输入文本中的每个字符。如果它是字母,则将其字符更改 13 个位置以获取匹配的 ROT13 字符。在收到输入消息后,main 方法调用 rot13 函数并输出加密文本。
示例
public class ROT13Class { // Function to perform ROT13 encryption public static String rot13Func(String text) { StringBuilder result = new StringBuilder(); for (char c : text.toCharArray()) { if (Character.isLetter(c)) { char base = Character.isLowerCase(c) ? 'a' : 'A'; c = (char) (((c - base + 13) % 26) + base); } result.append(c); } return result.toString(); } public static void main(String[] args) { String plaintext = "The world is so beautiful!"; System.out.println("The Plain Text Message: " + plaintext); String encryptedText = rot13Func(plaintext); System.out.println("The Encrypted text: " + encryptedText); } }
以下是上述示例的输出 -
输入/输出
The Plain Text Message: The world is so beautiful! The Encrypted text: Gur jbeyq vf fb ornhgvshy!
缺点
由于 ROT13 密码实际上是凯撒密码的一种特殊情况应用,因此它并不安全。尽管 ROT13 密码可以通过简单地将字母移动 13 个位置来破解,但凯撒密码只能通过频率分析或尝试所有 25 个密钥来破解。因此,它在现实生活中毫无用处。
ROT13 算法分析
ROT13 密码算法被认为是凯撒密码的一种特殊情况。它不是一种非常安全的算法,可以很容易地通过频率分析或仅尝试可能的 25 个密钥来破解,而 ROT13 可以通过移动 13 个位置来破解。因此,它没有任何实际用途。
总结
在本文中,我们检查了 Python 密码学 ROT13 算法。ROT13 是一种快速有效的方法,可以通过将字母表中的每个字母移动 13 个位置来加密给定的消息。它主要用于基本加密任务。我们在本章中实现了不同的方法,因此您现在可以使用 Python 中的 ROT13 算法加密和解密消息。