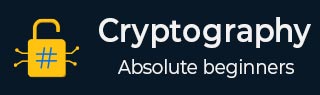
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组件
- 密码系统的攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学的类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥吊销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码的操作模式
- 分组密码的操作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 换位密码
- 密码学 - 加密换位密码
- 密码学 - 解密换位密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码的加密
- 密码学 - 简单替换密码的解密
- 密码学 - 维吉尼亚密码
- 密码学 - 维吉尼亚密码的实现
- 现代密码
- Base64 编码和解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 单字母替换密码的破解
- 密码学 - 多字母替换密码
- 密码学 - Playfair 密码
- 密码学 - Hill 密码
- 多字母替换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 换位技术
- 密码学 - 栅栏密码
- 密码学 - 列移位换位
- 密码学 -隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准
- 密码学 - 三重 DES
- 密码学 - 双重 DES
- 高级加密标准
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混淆变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码学算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施
- 哈希
- MD5(消息摘要算法 5)
- SHA-1(安全哈希算法 1)
- SHA-256(安全哈希算法 256 位)
- SHA-512(安全哈希算法 512 位)
- SHA-3(安全哈希算法 3)
- 哈希密码
- Bcrypt 哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码学协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像和文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用加密技术
- 自定义构建加密算法(混合加密)
- 云加密
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - 维吉尼亚密码的实现
在上一章中,我们看到了维吉尼亚密码,它的方法、优点和缺点。现在我们将使用不同的编程语言(如 Python、Java 和 C++)来实现维吉尼亚密码。
使用 Python 实现
这段 Python 代码实现了维吉尼亚密码,这是一种用于加密和解密文本消息的技术。generate_key() 函数以关键字作为输入,基于该关键字生成密钥,然后根据需要重复单词以确保密钥与文本长度匹配。encrypt_text() 函数使用维吉尼亚密码算法对文本进行加密,该算法根据密钥中匹配的字符对每个字符进行移位。同样,decrypt_text() 方法通过反转加密过程来解密密文并显示原始文本。
示例
以下是使用 Python 实现维吉尼亚密码的示例:
def generate_key(text, keyword): key = list(keyword) if len(text) == len(keyword): return key else: for i in range(len(text) - len(keyword)): key.append(key[i % len(keyword)]) return "".join(key) def encrypt_text(text, key): cipher_text = [] for i in range(len(text)): x = (ord(text[i]) + ord(key[i])) % 26 x += ord('A') cipher_text.append(chr(x)) return "".join(cipher_text) def decrypt_text(cipher_text, key): original_text = [] for i in range(len(cipher_text)): x = (ord(cipher_text[i]) - ord(key[i]) + 26) % 26 x += ord('A') original_text.append(chr(x)) return "".join(original_text) # Driver code text = "TUTORIALSPOINT" keyword = "KEY" key = generate_key(text, keyword) cipher_text = encrypt_text(text, key) print("The Encrypted text:", cipher_text) print("The Original/Decrypted Text:", decrypt_text(cipher_text, key))
以下是上述示例的输出:
输入/输出
The Encrypted text: DYRYVGKPQZSGXX The Original/Decrypted Text: TUTORIALSPOINT
使用 Java 实现
维吉尼亚密码是一种用于加密和解密消息的方法。代码使用文本消息的长度和一个关键字来生成密钥。然后,使用此密钥通过将字母表中的每个字符移位到密钥中其正确的位置来加密文本消息。为了解密消息,使用相同的密钥反转加密过程并将每个字符移回其原始字母位置。拥有密钥的各方可以彼此私下通信,结果是原始消息。
示例
请参阅以下维吉尼亚密码的 Java 实现:
public class VigenereCipher { static String generateKey(String text, String keyword) { int textLength = text.length(); for (int i = 0; ; i++) { if (textLength == i) i = 0; if (keyword.length() == textLength) break; keyword += (keyword.charAt(i)); } return keyword; } static String encryptText(String text, String keyword) { String ciphertext = ""; for (int i = 0; i < text.length(); i++) { // converting in range 0-25 int x = (text.charAt(i) + keyword.charAt(i)) % 26; // convert into alphabets(ASCII) x += 'A'; ciphertext += (char)(x); } return ciphertext; } // Decrypt the encrypted text and return the original text static String decryptText(String ciphertext, String keyword) { String originalText = ""; for (int i = 0 ; i < ciphertext.length() && i < keyword.length(); i++) { // converting in range 0-25 int x = (ciphertext.charAt(i) - keyword.charAt(i) + 26) % 26; // convert into alphabets(ASCII) x += 'A'; originalText += (char)(x); } return originalText; } // Driver code public static void main(String[] args) { String message = "Hello everyone"; String keyword = "Best"; String text = message.toUpperCase(); String key = keyword.toUpperCase(); String generatedKey = generateKey(text, key); String encryptedText = encryptText(text, generatedKey); System.out.println("The Encrypted Text : " + encryptedText); System.out.println("The Original/Decrypted Text : " + decryptText(encryptedText, generatedKey)); } }
以下是上述示例的输出:
输入/输出
The Encrypted Text : IIDEPXWOFVQHOI The Original/Decrypted Text : HELLOTEVERYONE
使用 C++ 实现
在本代码中,我们将使用 C++ 编程语言来实现维吉尼亚密码。在这个密码中,我们将对明文消息的字符应用一系列凯撒移位,使用一个密钥。密钥中的每个字母对应一个不同的移位值,密钥定义了对每个字符应用的移位量。在加密中,明文中的每个字符都根据密钥中给出的相应数字在字母表中向前移位。
同样,在解密中,通过将密文的字符向后移位相同数量(密钥中显示的数量),使原始明文可见。
示例
以下是使用 C++ 编程语言实现维吉尼亚密码的简单示例:
#include <iostream> #include <string> using namespace std; class VigenereCipher { public: string key; VigenereCipher(string key) { for (int i = 0; i < key.size(); ++i) { if (key[i] >= 'A' && key[i] <= 'Z') this -> key += key[i]; else if (key[i] >= 'a' && key[i] <= 'z') this -> key += key[i] + 'A' - 'a'; } } string encryptFunc(string text) { string out; for (int i = 0, j = 0; i < text.length(); ++i) { char c = text[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; out += (c + key[j] - 2 * 'A') % 26 + 'A'; j = (j + 1) % key.length(); } return out; } string decryptFunc(string text) { string out; for (int i = 0, j = 0; i < text.length(); ++i) { char c = text[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; out += (c - key[j] + 26) % 26 + 'A'; j = (j + 1) % key.length(); } return out; } }; int main() { VigenereCipher cipher("VigenereCipher"); string plaintext = "Cyber Security"; string et = cipher.encryptFunc(plaintext); string dt = cipher.decryptFunc(et); cout << "The Plaintext: " << plaintext << endl; cout << "The Encrypted Text: " << et << endl; cout << "The Decrypted Text: " << dt << endl; }
以下是上述示例的输出:
输入/输出
The Plaintext: Cyber Security The Encrypted Text: XGHIEWVGWZXAC The Decrypted Text: CYBERSECURITY
广告