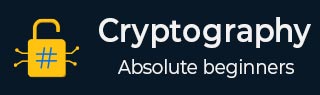
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力攻击
- 密码系统 - 密码分析技术
- 密码学类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码的工作模式
- 分组密码的工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 转置密码
- 密码学 - 加密转置密码
- 密码学 - 解密转置密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码的加密
- 密码学 - 简单替换密码的解密
- 密码学 - 维吉尼亚密码
- 密码学 - 维吉尼亚密码的实现
- 现代密码
- Base64 编码与解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单表代换密码
- 密码学 - 单表代换密码的破解
- 密码学 - 多表代换密码
- 密码学 - Playfair 密码
- 密码学 - Hill 密码
- 多表代换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 转置技术
- 密码学 - 栅栏密码
- 密码学 - 列移位转置
- 密码学 -隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准 (DES)
- 密码学 - 三重 DES
- 密码学 - 双重 DES
- 高级加密标准 (AES)
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混淆变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施 (PKI)
- 哈希
- MD5(消息摘要算法 5)
- SHA-1(安全哈希算法 1)
- SHA-256(安全哈希算法 256 位)
- SHA-512(安全哈希算法 512 位)
- SHA-3(安全哈希算法 3)
- 哈希密码
- Bcrypt 哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像与文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的密码技术
- 物联网设备的通信协议
- 常用密码技术
- 自定义构建密码算法(混合密码学)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学 vs 密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
自定义构建密码算法(混合密码学)
混合密码学结合了两种或多种加密技术。它结合了非对称加密和对称加密,以利用各自的功能。该方案使用公钥密码学来共享密钥,并使用对称加密来高效地加密消息。
混合加密方案结合了非对称加密方法的优点和对称加密方法的有效性。
要加密消息,首先生成一个对称密钥。然后,想要向其发送消息的人共享她的公钥,同时保持私钥私密。之后,使用接收者的公钥加密对称密钥并将其传输给他们。
要解密通信,接收者使用她的私钥解密加密的对称密钥以获得解密密钥,然后她使用该密钥来解码消息。
查看下面的图片以直观地了解混合密码学
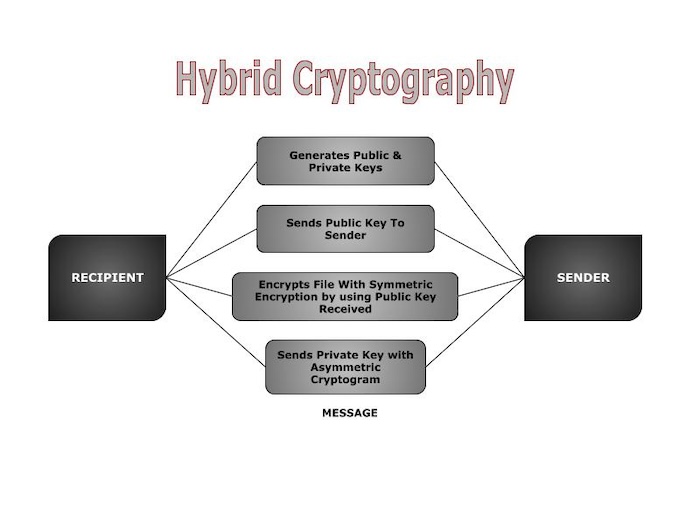
以下是我们在上图中提到的步骤的解释:
- 生成公钥和私钥 - 接收者首先创建一对密钥:公钥和私钥。
- 将公钥发送给发送者 - 接收者将其公钥发送给发送者。公钥可以公开分发,因为它仅用于加密。
- 使用接收到的公钥通过对称加密加密文件 - 发送者希望安全地将文件发送给接收者。发送者在使用对称加密加密文件的同时,也使用了接收者的公钥。这意味着只有拥有相应私钥的接收者才能解密文件。
- 使用非对称密码发送私钥 - 发送者现在将把接收者的私钥发送给他,但它是使用非对称加密加密的。此加密的私钥确保只有拥有相应私钥的预期接收者才能解密它。
混合密码学的实现
我们将借助不同的编程语言(如 Python、Java 和 C++)来实现混合密码学。因此,请参考以下部分的代码:
使用 Python
此实现中的自定义加密基于移动连接密钥中每个字符的 ASCII 值。对于偶数索引的字符,每个字符都按公钥的长度移动,对于奇数索引的字符,每个字符都按对称密钥的长度移动。这是一种简单的加密类型,它仍然使用组合密钥和应用自定义转换的方法。因此,请参见下面的 Python 实现:
def hybrid_encrypt(message, public_key, symmetric_key): # Reverse the message reversed_message = message[::-1] # Combine reversed message with symmetric key combined_key = reversed_message + symmetric_key # change combined key to list of characters key_characters = list(combined_key) # Apply custom encryption encrypted_characters = [] for i, char in enumerate(key_characters): if i % 2 == 0: encrypted_char = chr(ord(char) + len(public_key)) # Shift ASCII value by length of public key else: encrypted_char = chr(ord(char) - len(symmetric_key)) # Shift ASCII value by length of symmetric key encrypted_characters.append(encrypted_char) # Combine encrypted characters encrypted_message = ''.join(encrypted_characters) return encrypted_message # our message, public key and symmetric key message = "hellotutorialspoint" public_key = "qwer" symmetric_key = "private" # function execution encrypted_message = hybrid_encrypt(message, public_key, symmetric_key) print("Our Encrypted Message:", encrypted_message)
输出
Our Encrypted Message: xgmhtlpZmksmymsep^livbzZx^
使用 Java
此 Java 程序演示了使用给定密钥对明文消息应用的混合加密技术。main 方法初始化明文消息和密钥,然后调用 encryptMessage 函数来加密明文。
import java.util.*; class HybridEncryption { public static void main(String[] args) { String plaintext = "hellotutorialspoint"; String key = "qwer"; System.out.println("Our Encrypted Message is: " + encryptMessage(plaintext, key)); } public static String encryptMessage(String message, String key) { int a = 0, b = 1, c = 0, m = 0, k = 0, j = 0; String encryptedText = "", temp = ""; // Reverse the message StringBuilder reversedMessage = new StringBuilder(message).reverse(); // Combine the reversed message with the key StringBuilder combinedString = reversedMessage.append(key); // change the combined string to a character array char[] charArray = combinedString.toString().toCharArray(); String evenChars = "", oddChars = ""; // Separate characters into even and odd positions for (int i = 0; i < charArray.length; i++) { if (i % 2 == 0) { oddChars += charArray[i]; }else { evenChars += charArray[i]; } } char[] evenArray = new char[evenChars.length()]; char[] oddArray = new char[oddChars.length()]; // create a Fibonacci series and apply Caesar cipher while (m <= key.length()) { if (m == 0) m = 1; else { a = b; b = c; c = a + b; for (int i = 0; i < evenChars.length(); i++) { int p = evenChars.charAt(i); int cipher = 0; if (Character.isDigit(p)) { cipher = p - c; if (cipher < '0') cipher = cipher + 9; }else { cipher = p - c; if (cipher < 'a') { cipher = cipher + 26; } } evenArray[i] = (char)cipher; } for (int i = 0; i < oddChars.length(); i++) { int p = oddChars.charAt(i); int cipher = 0; if (Character.isDigit(p)) { cipher = p + c; if (cipher > '9') cipher = cipher - 9; }else { cipher = p + c; if (cipher > 'z') { cipher = cipher - 26; } } oddArray[i] = (char)cipher; } m++; } } // Combine even and odd characters based on their positions for (int i = 0; i < charArray.length; i++) { if (i % 2 == 0) { charArray[i] = oddArray[k]; k++; }else { charArray[i] = evenArray[j]; j++; } } // Generate the encrypted text for (char d : charArray) { encryptedText = encryptedText + d; } // Return the encrypted text return encryptedText; } }
输出
Our Encrypted Message is: wkllspoxlorqxqriobknzbu
使用 C++
此 C++ 代码显示了一种自定义加密算法,该算法结合了反向字符串操作和凯撒密码来加密消息。
#include <iostream> #include <string> #include <algorithm> // Include the algorithm header for the reverse function using namespace std; string hybridEncryption(string password, string key) { int a = 0, b = 1, c = 0, m = 0, k = 0, j = 0; string cipherText = "", temp = ""; // Declare a password string string reversedPassword = password; // Reverse the String reverse(reversedPassword.begin(), reversedPassword.end()); // Use the reverse function reversedPassword = reversedPassword + key; // For future Purpose temp = reversedPassword; string charArray = temp; string evenChars = "", oddChars = ""; // Declare EvenArray for storing // even index of charArray char *evenArray; // Declare OddArray for storing // odd index of charArray char *oddArray; // Storing the positions in their // respective arrays for (int i = 0; i < charArray.length(); i++) { if (i % 2 == 0) { oddChars = oddChars + charArray[i]; }else { evenChars = evenChars + charArray[i]; } } evenArray = new char[evenChars.length()]; oddArray = new char[oddChars.length()]; // Generate a Fibonacci Series // Upto the Key Length while (m <= key.length()) { // As it always starts with 1 if (m == 0) m = 1; else { // Logic For Fibonacci Series a = b; b = c; c = a + b; for (int i = 0; i < charArray.length(); i++) { // Caesar Cipher Algorithm Start // for even positions int p = charArray[i]; int cipher = 0; if (p == '0' || p == '1' || p == '2' || p == '3' || p == '4' || p == '5' || p == '6' || p == '7' || p == '8' || p == '9') { cipher = p - c; if (cipher < '0') cipher = cipher + 9; }else { cipher = p - c; if (cipher < 'a') { cipher = cipher + 26; } } evenArray[i] = (char)cipher; // Caesar Cipher Algorithm End } for (int i = 0; i < charArray.length(); i++) { // Caesar Cipher Algorithm // Start for odd positions int p = charArray[i]; int cipher = 0; if (p == '0' || p == '1' || p == '2' || p == '3' || p == '4' || p == '5' || p == '6' || p == '7' || p == '8' || p == '9') { cipher = p + c; if (cipher > '9') cipher = cipher - 9; }else { cipher = p + c; if (cipher > 'z') { cipher = cipher - 26; } } oddArray[i] = (char)cipher; // Caesar Cipher Algorithm End } m++; } } // Storing content of even and // odd array to the string array for (int i = 0; i < charArray.length(); i++) { if (i % 2 == 0) { charArray[i] = oddArray[k]; k++; }else { charArray[i] = evenArray[j]; j++; } } // Generating a Cipher Text // by charArray (Caesar Cipher) for (char d : charArray) { cipherText = cipherText + d; } // Return the Cipher Text return cipherText; } // Driver code int main() { string pass = "hellotutorialspoint"; string key = "qwer"; cout <<"Our Encrypted Message is: "<< hybridEncryption(pass, key); return 0; }
输出
Our Encrypted Message is: wqqklfrlsmvpoidxlfuorlw
混合密码学的优点
混合加密是对称加密和非对称加密的组合,它比以前的方法提供了更高的安全性。数据的传输变得安全。在传输过程中加密数据可以提供安全优势,就像所有设备上都能确保数据安全一样。
广告