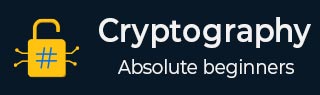
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密需求
- 密码学 - 双重加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码工作模式
- 分组密码工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 换位密码
- 密码学 - 加密换位密码
- 密码学 - 解密换位密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码加密
- 密码学 - 简单替换密码解密
- 密码学 - 维吉尼亚密码
- 密码学 - 实现维吉尼亚密码
- 现代密码
- Base64 编码和解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单表替换密码
- 密码学 - 破解单表替换密码
- 密码学 - 多表替换密码
- 密码学 - Playfair 密码
- 密码学 - 希尔密码
- 多表替换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 换位技术
- 密码学 - 栅栏密码
- 密码学 - 列置换密码
- 密码学 - 隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准
- 密码学 - 三重 DES
- 密码学 - 双重 DES
- 高级加密标准
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混合变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码学算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施
- 哈希
- MD5(消息摘要算法 5)
- SHA-1(安全哈希算法 1)
- SHA-256(安全哈希算法 256 位)
- SHA-512(安全哈希算法 512 位)
- SHA-3(安全哈希算法 3)
- 哈希密码
- Bcrypt 哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像和文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用加密技术
- 自定义构建加密算法(混合加密)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - RSA 解密
在本文中,我们必须使用不同的技术来实现 RSA 密码的解密。如您所知,解密是在非对称密钥密码学中使用私钥将加密消息(密文)更改回其原始形式(明文)的过程。或者我们可以说解密是找到加密消息的原始消息的技术。
解密步骤
我们可以使用以下步骤解密使用 RSA 加密加密的消息:
- 接收密文 - 接收者收到一条使用其公开密钥加密的加密消息(密文)。
- 使用私钥 - 接收者使用秘密密钥(私钥)来解密密文。此秘密密钥是在特殊设置期间与公钥一起创建的。
- 执行解密 - 为了解密消息,接收者使用涉及秘密密钥和大数的复杂数学运算,有效地逆转加密过程。这将导致原始消息的数字表示。
- 转换为明文 - 然后将数字表示转换回原始消息内容。
Python 中的解密实现
RSA 解密可以通过以下几种方法创建:
- 使用模算术和数论
- 使用 RSA 模块
- 使用 cryptography 模块
为了帮助您更好地理解 RSA 解密、Python 编程和函数,我们将在下面的部分中深入讨论每种方法。
使用模算术和数论
在这个程序中,我们将使用模算术和数论来解密消息。在我们的代码中,decrypt() 函数将私钥和密文消息作为输入。解密密文涉及将密文中每个数字提高到“d”指数指定的幂。应用“mod n”计算(一种数学运算)。使用“chr”函数将结果数字转换回字符。组合这些字符以获得解密的消息(明文)。
示例
以下是使用模算术和数论进行 RSA 解密的 Python 程序:
import random def gcd(a, b): while b != 0: a, b = b, a % b return a def extended_gcd(a, b): if a == 0: return (b, 0, 1) else: g, y, x = extended_gcd(b % a, a) return (g, x - (b // a) * y, y) def mod_inverse(a, m): g, x, y = extended_gcd(a, m) if g != 1: raise Exception('Modular inverse does not exist') else: return x % m def generate_keypair(): p = random.randint(100, 1000) q = random.randint(100, 1000) n = p * q phi = (p - 1) * (q - 1) while True: e = random.randint(2, phi - 1) if gcd(e, phi) == 1: break d = mod_inverse(e, phi) return ((e, n), (d, n)) def encrypt(public_key, plaintext): e, n = public_key cipher = [pow(ord(char), e, n) for char in plaintext] return cipher def decrypt(private_key, ciphertext): d, n = private_key plain = [chr(pow(char, d, n)) for char in ciphertext] return ''.join(plain) # our keys public_key, private_key = generate_keypair() # plaintext message = "Hello, world!" encrypted_message = encrypt(public_key, message) print("Encrypted message:", encrypted_message) decrypted_message = decrypt(private_key, encrypted_message) print("Decrypted message:", decrypted_message)
以下是上述示例的输出:
输入/输出
Encrypted message: [5133, 206, 9012, 9012, 7041, 3509, 7193, 3479, 7041, 6939, 9012, 1255, 3267] Decrypted message: Hello, world!
使用 rsa 模块
可以使用 Python 的 rsa 包轻松高效地实现 RSA 算法。首先,如果您尚未安装 rsa 模块,则可以使用 pip 安装它 - pip install rsa。因此,我们首先需要生成密钥,然后我们将解密加密的消息。
生成 RSA 密钥
安装模块后,您可以使用此 rsa 模块生成 RSA 密钥对。方法如下:
示例
import rsa # create a key pair with 1024 bits key length (public_key, private_key) = rsa.newkeys(1024)
代码将生成一对 RSA 密钥:一个用于加密(公钥),另一个用于解密(私钥)。
解密消息
拥有密钥对后,您可以使用它们来解密消息。
# Encrypting a message message = b"Hello, world!" encrypted_message = rsa.encrypt(message, public_key) # Decrypting the message decrypted_message = rsa.decrypt(encrypted_message, private_key) print("Encrypted message:", encrypted_message) print("Decrypted message:", decrypted_message.decode())
完整代码
以下是供您参考的 Python 代码。因此,运行代码并查看输出:
示例
import rsa # create a key pair with 1024 bits key length (public_key, private_key) = rsa.newkeys(1024) # Encrypting a message message = b"Hello, world!" encrypted_message = rsa.encrypt(message, public_key) # Decrypting the message decrypted_message = rsa.decrypt(encrypted_message, private_key) print("Encrypted message:", encrypted_message) print("Decrypted message:", decrypted_message.decode())
以下是上述示例的输出:
输入/输出
Encrypted message: b'+.}a\n\xda8\x83\xf7D\x0eI\xc1\xde\x9cZ\xd7\xf2\xdd}\xb0\xd4N\x9e\x1cjm\xe3\x12\xea\x95\n8\x87a\x16Y\xb6\x7f\x9ar\xf3\xd6\xe6le\xda1\xa7{\xa1\xa4\t/q\xea\x19*q\xc5[\x87\x1b\xc0\x8f%\x83\xa1KFx\xe6m\x97\x8ef\x84\xaf\xe7\xdc\xcf\xd0\xe4\x80H!\xce\xef\x13\xc5\x93\xc3a\xc0Pv\x07tQx_\xbc\xaeQ[x:\xf3\x19\xa2\xea]\xf2\x1e\x9e9\x10\xed\xf2\x83\x15W}\xbb\x89\xd7\xf6\xee' Decrypted message: Hello, world!
使用 cryptography 模块
使用 cryptography 模块,我们可以创建 RSA 密钥对。此代码创建一个私有 RSA 密钥并提取其对应的公钥。密钥转换为 PEM 格式并保存在不同的文件中。我们可以使用这些密钥来加密和解密消息。给定的代码显示了如何使用私钥进行解密。
示例
以下是使用 cryptography 模块进行 RSA 解密的 Python 实现:
from cryptography.hazmat.backends import default_backend from cryptography.hazmat.primitives import serialization from cryptography.hazmat.primitives.asymmetric import rsa from cryptography.hazmat.primitives import hashes from cryptography.hazmat.primitives.asymmetric import padding # Generate an RSA private key private_key = rsa.generate_private_key( public_exponent=65537, key_size=2048, backend=default_backend() ) # Get the public key from the private key public_key = private_key.public_key() # Serialize the keys to PEM format private_key_pem = private_key.private_bytes( encoding=serialization.Encoding.PEM, format=serialization.PrivateFormat.PKCS8, encryption_algorithm=serialization.NoEncryption() ) public_key_pem = public_key.public_bytes( encoding=serialization.Encoding.PEM, format=serialization.PublicFormat.SubjectPublicKeyInfo ) # Save the keys to files or use them as needed with open('private_key.pem', 'wb') as f: f.write(private_key_pem) with open('public_key.pem', 'wb') as f: f.write(public_key_pem) # Encrypting a message message = b"Hello, world!" encrypted_message = public_key.encrypt( message, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) # Decrypting the message decrypted_message = private_key.decrypt( encrypted_message, padding.OAEP( mgf=padding.MGF1(algorithm=hashes.SHA256()), algorithm=hashes.SHA256(), label=None ) ) print("Encrypted message:", encrypted_message) print("Decrypted message:", decrypted_message.decode())
以下是上述示例的输出:
输入/输出
Encrypted message: b'\xae\xdd\xaa\x16\xd5\x9e\x06N\xf7\x96\xd6\xd6\x02\x8b\xcb\xdf\x12eds\t\x1a\xa9\xb6\xe3\xc4vZ\x05\xbe\xbf$\xef\xaa\xcfqo\xc0\x8fI\x80\xb3\xea\xb6\x04\xf8\xc6\x05\xaa\x1dH\xc1J{=\x15\xe1\xef\xcbnA\xfb\x98m.\xa7\x9c\x05\x0f\x07z\x02(\x10\xae\xaf\xccv\x8a\x8f\x97\'f-\xccFU\xf8\x188\xe7\xe5I\xcc\x88\n_\xa6w\x86\x9b\x03\xc7\x96t\xca\xdb\x102O#\x94\xd0\xbe\xba\x8d\xce\x80V*l\xb5"\xdf~\x86\xe6\xf6\x08\x18\xcf\xd8\x866\x88\xe3x\x17\xebhD\xdb\xa8\x02\xa1\xd9{\xaf~\xe4\xd7[\x9c+\xcc\t\x15\x8ej\xe2\xe5b\xdf\xeb\xd0|\x82\xea\xce,p.\xf5\xd6\x0e\x01\xb9\xcb\x17\xe6\x05\xea\xe4\xb0\xa4\xc8\x9a\xc4\x822\xd1v\x8a\xa6\x85{x\xf9\x81r\xa2S\xe1\xe7l\xccwE\x16f\x1e\xfbE\x1e\xaf|\xc3\x91\x93\x99|\x99\xba\xcf\x00\xb3D\xfaq\xc3<\x8dj\x06\\L\x06{\x10\xaf7\xfa^\xa5\x87E\xbb\x8c\x9e\xd7eU\x07\xf3lk' Decrypted message: Hello, world!
使用 Java 实现 RSA 解密
下面的 Java 代码使用 RSA 加密算法来解密给定的消息。该代码使用 Java 的不同库来解密消息。
示例
使用 Java 实现 RSA 解密过程如下
import java.math.BigInteger; import java.security.SecureRandom; public class RSA { private BigInteger prKey; private BigInteger pubKey; private BigInteger mod; // Generate public and private keys public RSA(int bitLength) { SecureRandom random = new SecureRandom(); BigInteger p = BigInteger.probablePrime(bitLength / 2, random); BigInteger q = BigInteger.probablePrime(bitLength / 2, random); mod = p.multiply(q); BigInteger phi = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE)); pubKey = BigInteger.probablePrime(bitLength / 4, random); while (phi.gcd(pubKey).intValue() > 1) { pubKey = pubKey.add(BigInteger.ONE); } prKey = pubKey.modInverse(phi); } // Encrypt plaintext public BigInteger encrypt(String message) { BigInteger plaintext = new BigInteger(message.getBytes()); return plaintext.modPow(pubKey, mod); } // Decrypt ciphertext public String decrypt(BigInteger encryptedMessage) { BigInteger decrypted = encryptedMessage.modPow(prKey, mod); return new String(decrypted.toByteArray()); } public static void main(String[] args) { RSA rsa = new RSA(1024); // Message to encrypt String plaintext = "HELLO TUTORIALSPOINT"; // Encrypt the message BigInteger ciphertext = rsa.encrypt(plaintext); System.out.println("Encrypted Message: " + ciphertext); // Decrypt the ciphertext String decryptedMessage = rsa.decrypt(ciphertext); System.out.println("Decrypted Message: " + decryptedMessage); } }
以下是上述示例的输出:
输入/输出
Encrypted Message: 81613159022598431502618861082122488243352277400520456503112436392671015741839175478780136032663342240432362810242747991048788272007296529186453061811896882040496871941396445756607265971854347817972502178724652319503362063137755270102568091525122886513678255187855721468502781772407941859987159924896465083062 Decrypted Message: HELLO TUTORIALSPOINT
使用 C++ 实现 RSA 解密
下面是 RSA 算法的一个简单实现,它使用 C++ 的帮助来解密消息。给定消息解密的代码如下:
示例
#include<iostream> #include<math.h> using namespace std; // find gcd int gcd(int a, int b) { int t; while(1) { t= a%b; if(t==0) return b; a = b; b= t; } } int main() { //2 random prime numbers double p = 13; double q = 11; double n=p*q;//calculate n double track; double phi= (p-1)*(q-1);//calculate phi //public key //e stands for encrypt double e=7; //for checking that 1 < e < phi(n) and gcd(e, phi(n)) = 1; i.e., e and phi(n) are coprime. while(e<phi) { track = gcd(e,phi); if(track==1) break; else e++; } //private key //d stands for decrypt //choosing d such that it satisfies d*e = 1 mod phi double d1=1/e; double d=fmod(d1,phi); double message = 9; double c = pow(message,e); //encrypt the message double m = pow(c,d); c=fmod(c,n); m=fmod(m,n); cout<<"\n"<<"Encrypted message = "<<c; cout<<"\n"<<"Decrypted message = "<<m; return 0; }
以下是上述示例的输出:
输入/输出
Encrypted message = 48 Decrypted Message = 9
总结
RSA 通过创建唯一的公钥和私钥对来工作,从而建立安全的通信通道。这些密钥用于加密和解密消息,确保只有授权用户才能解密信息。
在 Python 中,RSA 解密可以通过多种方法实现。使用模算术和数论,而 RSA 模块提供了更专业的的功能。cryptography 模块还支持 RSA 加密和解密,提供了一套全面的功能。开发人员可以选择最符合其需求的方法。
我们还开发了使用 Java 和 C++ 进行 RSA 解密的代码。