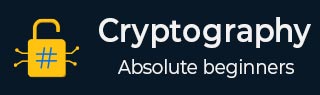
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代密码学
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学哈希函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码的工作模式
- 分组密码的工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密文反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 反向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 转置密码
- 密码学 - 转置密码加密
- 密码学 - 转置密码解密
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码加密
- 密码学 - 简单替换密码解密
- 密码学 - 维吉尼亚密码
- 密码学 - 维吉尼亚密码的实现
- 现代密码
- Base64 编码与解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 单字母替换密码的破解
- 密码学 - 多字母替换密码
- 密码学 - Playfair 密码
- 密码学 - Hill 密码
- 多字母替换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 转置技术
- 密码学 - 栅栏密码
- 密码学 - 列置换密码
- 密码学 - 密码隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准 (DES)
- 密码学 - 三重 DES
- 密码学 - 双重 DES
- 高级加密标准 (AES)
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混淆变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施 (PKI)
- 哈希
- MD5(消息摘要算法 5)
- SHA-1(安全哈希算法 1)
- SHA-256(安全哈希算法 256 位)
- SHA-512(安全哈希算法 512 位)
- SHA-3(安全哈希算法 3)
- 密码哈希
- Bcrypt 哈希模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像和文件加密
- 密码学 - 图像
- 密码学 - 文件
- 密码隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的密码技术
- 物联网设备的通信协议
- 常用的密码技术
- 自定义构建密码算法(混合密码学)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典密码学与量子密码学
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称密码与非对称密码
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - 转置密码解密
在上一章中,我们学习了转置密码加密。现在我们将学习转置密码的解密及其使用不同方法的实现。
解密过程只是反转加密算法的步骤。我们使用相同的密钥来查找读取列以恢复实际消息的正确顺序。
转置密码解密算法
以下是转置密码解密的算法:
输入
密文 - 加密的消息。
密钥 - 用于加密的相同密钥。
步骤
首先,我们需要根据密钥长度将密文分成多行。这意味着我们将为密钥中的每个字符创建一个行。
现在,我们将创建一个空的网格,其行数与密钥长度相同,列数等于密文长度除以密钥长度的结果。
接下来,我们必须根据密钥填充网格。因此,迭代密文字符以确定其列索引。
并将字符添加到网格中的相应单元格中。
从第一列开始,向下读取字符,到达底部后移动到下一列。对所有列继续此过程。这会根据原始转置顺序重新排列字符。
打印明文消息。
示例
输入
密文:OLHEL LWRDO
密钥:SECRET
例如,如果我们知道密钥是“SECRET”,并且原始消息是根据密钥中字母的顺序进行转置的。我们可以像下面这样重新排列列:
密钥 | S E C R E T |
列 | 5, 1, 2, 4, 6, 3 |
现在,我们将根据密钥重新排序转置消息“OLHEL LWRDO”的列,如下所示:
S | O | L |
E | H | E |
C | L | W |
R | H | R |
E | E | D |
T | L | O |
最后,我们逐行读取字符以获取原始消息:
原始消息:HELLO WORLD
因此,使用密钥“SECRET”对加密消息“OLHEL LWRDO”解密后的消息是“HELLO WORLD”。
太棒了!我们已经使用转置密码解密了我们的消息。
使用 Python 实现
可以使用不同的方法实现转置密码的解密:
使用 Math 模块
使用 Pyperclip 模块
因此,让我们在以下部分逐一查看这两种方法:
使用 Math 模块
此 Python 代码解密使用转置密码加密的消息。我们将使用 math 模块执行数学运算,主要是计算解密消息所需的列数。math.ceil() 用于将消息长度除以密钥的结果向上取整。因此,此代码有效地解密转置密码消息。
示例
以下是使用 Math 模块的转置密码解密算法的 Python 代码。请参见下面的程序:
import math def transposition_decrypt(key, message): num_of_columns = math.ceil(len(message) / key) num_of_rows = key num_of_shaded_boxes = (num_of_columns * num_of_rows) - len(message) plaintext = [''] * num_of_columns col = 0 row = 0 for symbol in message: plaintext[col] += symbol col += 1 if (col == num_of_columns) or (col == num_of_columns - 1 and row >= num_of_rows - num_of_shaded_boxes): col = 0 row += 1 return ''.join(plaintext) ciphertext = 'Toners raiCntisippoh' key = 6 plaintext = transposition_decrypt(key, ciphertext) print("Cipher Text: ", ciphertext) print("The plain text is: ", plaintext)
以下是上述示例的输出:
输入/输出
Cipher Text: Toners raiCntisippoh The plain text is: Transposition Cipher
在上面的输出中,您可以看到密文消息是 Toners raiCntisippoh,解密后的消息是 Transposition Cipher。
使用 Pyperclip 模块
在此示例中,我们将使用 Python 的 pyperclip 模块,它用于复制和粘贴剪贴板功能。因此,我们将使用此模块将解密的消息复制到剪贴板。
此代码与上面的代码类似,但在本代码中,我们使用 pyperclip 模块将解密的消息复制到剪贴板。
示例
以下是使用 pyperclip 模块的转置密码解密算法的 Python 代码。请检查下面的代码:
import math import pyperclip def transposition_decrypt(key, message): num_of_columns = math.ceil(len(message) / key) num_of_rows = key num_of_shaded_boxes = (num_of_columns * num_of_rows) - len(message) plaintext = [''] * num_of_columns col = 0 row = 0 for symbol in message: plaintext[col] += symbol col += 1 if (col == num_of_columns) or (col == num_of_columns - 1 and row >= num_of_rows - num_of_shaded_boxes): col = 0 row += 1 return ''.join(plaintext) ciphertext = 'Toners raiCntisippoh' key = 6 plaintext = transposition_decrypt(key, ciphertext) print("Cipher Text:", ciphertext) print("The plain text is:", plaintext) # Copy the decrypted plaintext to the clipboard pyperclip.copy(plaintext) print("The Decrypted Message is Copied to the Clipboard")
以下是上述示例的输出:
输入/输出
Cipher Text: Toners raiCntisippoh The plain text is: Transposition Cipher The Decrypted Message is Copied to the Clipboard
在上面的输出中,我们可以看到明文已复制到剪贴板,并且显示消息“解密后的消息已复制到剪贴板”。
使用 Java 实现
我们将使用 Java 编程语言来实现转置密码的解密。基本上,我们将使用 Java 在此实现中反转加密过程。
请参见 Java 中的以下代码:
示例
public class TranspositionCipher { // Function to decrypt using transposition cipher public static String decrypt(String ciphertext, int key) { StringBuilder plaintext = new StringBuilder(); int cols = (int) Math.ceil((double) ciphertext.length() / key); char[][] matrix = new char[cols][key]; // Fill matrix with ciphertext characters int index = 0; for (int j = 0; j < key; ++j) { for (int i = 0; i < cols; ++i) { if (index < ciphertext.length()) matrix[i][j] = ciphertext.charAt(index++); else matrix[i][j] = ' '; } } // Read matrix row-wise to get plaintext for (int i = 0; i < cols; ++i) { for (int j = 0; j < key; ++j) { plaintext.append(matrix[i][j]); } } return plaintext.toString(); } public static void main(String[] args) { String ciphertext = "Hohiebtlre,isrei ll s yafwdlT v uuo "; int key = 4; System.out.println("The Encrypted text: " + ciphertext); String decryptedText = decrypt(ciphertext, key); System.out.println("Decrypted text: " + decryptedText); } }
以下是上述示例的输出:
输入/输出
The Encrypted text: Hohiebtlre,isrei ll s yafwdlT v uuo Decrypted text: Hello, This is very beautiful world
使用 C++ 实现
现在,我们将使用 C++ 编程语言来实现转置密码的解密算法。在其中,我们将通过 decrypt 函数反转加密过程。它通过计算密文长度和密钥来找到所需的列数。它将密文中的字符按列添加到矩阵中。之后,它使用矩阵的逐行读取来构建明文。
以下是使用 C++ 实现转置密码解密的代码:
示例
#include <iostream> #include <string> #include <cmath> // Function to decrypt using transposition cipher std::string decrypt(std::string ciphertext, int key) { std::string plaintext = ""; int cols = (ciphertext.length() + key - 1) / key; char matrix[cols][key]; // Fill matrix with ciphertext characters int index = 0; for (int j = 0; j < key; ++j) { for (int i = 0; i < cols; ++i) { if (index < ciphertext.length()) matrix[i][j] = ciphertext[index++]; else matrix[i][j] = ' '; } } // Read matrix row-wise to get plaintext for (int i = 0; i < cols; ++i) { for (int j = 0; j < key; ++j) { plaintext += matrix[i][j]; } } return plaintext; } int main() { std::string ciphertext = "Houiptme,tao il oliFllTrsnay"; int key = 4; std::cout << "The Encrypted text: " << ciphertext << std::endl; std::string decrypted_text = decrypt(ciphertext, key); std::cout << "The Decrypted text: " << decrypted_text << std::endl; return 0; }
以下是上述示例的输出:
输入/输出
The Encrypted text: Houiptme,tao il oliFllTrsnay The Decrypted text: Hello, Tutorialspoint Family
总结
在本章中,我们已经了解了如何使用 Python、C++ 和 Java 中的转置密码解密算法将密文解密回其原始形式。