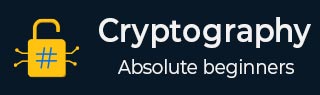
- 密码学教程
- 密码学 - 首页
- 密码学 - 起源
- 密码学 - 历史
- 密码学 - 原理
- 密码学 - 应用
- 密码学 - 优点与缺点
- 密码学 - 现代
- 密码学 - 传统密码
- 密码学 - 加密的需求
- 密码学 - 双重强度加密
- 密码系统
- 密码系统
- 密码系统 - 组成部分
- 密码系统的攻击
- 密码系统 - 彩虹表攻击
- 密码系统 - 字典攻击
- 密码系统 - 暴力破解攻击
- 密码系统 - 密码分析技术
- 密码学的类型
- 密码系统 - 类型
- 公钥加密
- 现代对称密钥加密
- 密码学散列函数
- 密钥管理
- 密码系统 - 密钥生成
- 密码系统 - 密钥存储
- 密码系统 - 密钥分发
- 密码系统 - 密钥撤销
- 分组密码
- 密码系统 - 流密码
- 密码学 - 分组密码
- 密码学 - Feistel 分组密码
- 分组密码的工作模式
- 分组密码的工作模式
- 电子密码本 (ECB) 模式
- 密码分组链接 (CBC) 模式
- 密码反馈 (CFB) 模式
- 输出反馈 (OFB) 模式
- 计数器 (CTR) 模式
- 经典密码
- 密码学 - 逆向密码
- 密码学 - 凯撒密码
- 密码学 - ROT13 算法
- 密码学 - 换位密码
- 密码学 - 加密换位密码
- 密码学 - 解密换位密码
- 密码学 - 乘法密码
- 密码学 - 仿射密码
- 密码学 - 简单替换密码
- 密码学 - 简单替换密码的加密
- 密码学 - 简单替换密码的解密
- 密码学 - 维吉尼亚密码
- 密码学 - 实现维吉尼亚密码
- 现代密码
- Base64 编码与解码
- 密码学 - XOR 加密
- 替换技术
- 密码学 - 单字母替换密码
- 密码学 - 破解单字母替换密码
- 密码学 - 多字母替换密码
- 密码学 - Playfair 密码
- 密码学 - 希尔密码
- 多字母替换密码
- 密码学 - 一次性密码本密码
- 一次性密码本密码的实现
- 密码学 - 换位技术
- 密码学 - 栅栏密码
- 密码学 - 列置换密码
- 密码学 - 隐写术
- 对称算法
- 密码学 - 数据加密
- 密码学 - 加密算法
- 密码学 - 数据加密标准
- 密码学 - 三重 DES
- 密码学 - 双重 DES
- 高级加密标准
- 密码学 - AES 结构
- 密码学 - AES 变换函数
- 密码学 - 字节替换变换
- 密码学 - 行移位变换
- 密码学 - 列混合变换
- 密码学 - 轮密钥加变换
- 密码学 - AES 密钥扩展算法
- 密码学 - Blowfish 算法
- 密码学 - SHA 算法
- 密码学 - RC4 算法
- 密码学 - Camellia 加密算法
- 密码学 - ChaCha20 加密算法
- 密码学 - CAST5 加密算法
- 密码学 - SEED 加密算法
- 密码学 - SM4 加密算法
- IDEA - 国际数据加密算法
- 公钥(非对称)密码学算法
- 密码学 - RSA 算法
- 密码学 - RSA 加密
- 密码学 - RSA 解密
- 密码学 - 创建 RSA 密钥
- 密码学 - 破解 RSA 密码
- 密码学 - ECDSA 算法
- 密码学 - DSA 算法
- 密码学 - Diffie-Hellman 算法
- 密码学中的数据完整性
- 密码学中的数据完整性
- 消息认证
- 密码学数字签名
- 公钥基础设施
- 散列
- MD5(消息摘要算法 5)
- SHA-1(安全散列算法 1)
- SHA-256(安全散列算法 256 位)
- SHA-512(安全散列算法 512 位)
- SHA-3(安全散列算法 3)
- 散列密码
- Bcrypt 散列模块
- 现代密码学
- 量子密码学
- 后量子密码学
- 密码学协议
- 密码学 - SSL/TLS 协议
- 密码学 - SSH 协议
- 密码学 - IPsec 协议
- 密码学 - PGP 协议
- 图像与文件加密
- 密码学 - 图像
- 密码学 - 文件
- 隐写术 - 图像
- 文件加密和解密
- 密码学 - 文件加密
- 密码学 - 文件解密
- 物联网中的密码学
- 物联网安全挑战、威胁和攻击
- 物联网安全的加密技术
- 物联网设备的通信协议
- 常用加密技术
- 自定义构建加密算法(混合加密)
- 云密码学
- 量子密码学
- 密码学中的图像隐写术
- DNA 密码学
- 密码学中的一次性密码 (OTP) 算法
- 区别
- 密码学 - MD5 与 SHA1
- 密码学 - RSA 与 DSA
- 密码学 - RSA 与 Diffie-Hellman
- 密码学与密码学
- 密码学 - 密码学与密码分析
- 密码学 - 经典与量子
- 密码学与隐写术
- 密码学与加密
- 密码学与网络安全
- 密码学 - 流密码与分组密码
- 密码学 - AES 与 DES 密码
- 密码学 - 对称与非对称
- 密码学有用资源
- 密码学 - 快速指南
- 密码学 - 讨论
密码学 - 简单替换密码的解密
在上一章中,我们看到了简单替换密码的不同加密程序。现在我们将讨论解密方法,使用 Python、Java 和 C++ 使用简单替换密码解密给定的消息。
如您所知,解密是加密的反向过程,因此我们将反转我们在上一章中为加密创建的所有程序。
使用 Python 实现
我们可以使用以下方法实现简单替换密码的解密:
使用指定的偏移量
使用字典映射
直接使用 ASCII 值
在接下来的部分中,我们将尝试详细描述这些方法中的每一种,以便您可以更好地理解 Python 密码学和简单替换密码解密。
方法 1:使用指定的偏移量
要为之前的加密程序创建解密函数,我们可以使用相同的方法,但反转偏移方向。以下函数通过以与原始偏移相反的方向移动密文中每个字母来反转加密过程。它的工作原理是减去偏移量而不是加上它。所以基本上我们在撤销加密。
以下是使用此方法在 Python 中实现解密过程:
示例
# function for decryption def substitution_decrypt(encrypted_text, shift): decrypted_text = "" for char in encrypted_text: if char.isalpha(): ascii_val = ord(char) if char.isupper(): # Reverse the shift for uppercase letters shifted_ascii = ((ascii_val - 65 - shift) % 26) + 65 else: # Reverse the shift for lowercase letters shifted_ascii = ((ascii_val - 97 - shift) % 26) + 97 decrypted_char = chr(shifted_ascii) decrypted_text += decrypted_char else: decrypted_text += char return decrypted_text # our cipher text encrypted_text = "Mjqqt, jajwdtsj!" shift = 5 decrypted_text = substitution_decrypt(encrypted_text, shift) print("Cipher Text: ", encrypted_text) print("Decrypted text:", decrypted_text)
以下是上述示例的输出:
输入/输出
Cipher Text: Mjqqt, jajwdtsj! Decrypted text: Hello, everyone!
方法 2:使用字典映射
在这里,我们将使用字典映射来实现简单替换密码解密。正如我们在上一章中使用字典映射来加密明文一样。现在我们将使用相同的技术,但反向。我们需要反转此映射。而不是将每个字母映射到下一个字母,我们将密文中每个字母映射回明文中的原始字母。
这需要创建一个反向字典,其中键是密文中的字符,值是明文中的相应字符。
以下是使用反向字典映射实现简单替换密码解密的 Python 代码:
# function for decryption def substitution_decrypt(encrypted_text, key): # a reverse dictionary mapping for decryption reverse_mapping = {key[i]: chr(97 + i) for i in range(26)} # Decrypt the using reverse mapping decrypted_text = ''.join(reverse_mapping.get(char, char) for char in encrypted_text) return decrypted_text # our cipher text encrypted_text = "ifmmp, efbs gsjfoe!" key = "bcdefghijklmnopqrstuvwxyza" # Example substitution key decrypted_text = substitution_decrypt(encrypted_text, key) print("Cipher Text: ", encrypted_text) print("Decrypted text:", decrypted_text)
以下是上述示例的输出:
输入/输出
Cipher Text: ifmmp, efbs gsjfoe! Decrypted text: hello, dear friend!
方法 3:直接使用 ASCII 值
ASCII 是一种字符编码标准,我们为字母、数字和其他字符分配数值。在加密过程中,明文的每个字母都会使用 ASCII 值偏移固定数量。现在我们必须解密,因此我们将通过从每个字符中减去相同的固定数量来反转偏移过程。
例如,如果我们正在解密偏移了 3 的“d”(ASCII 值 100),我们将减去 3 以返回到“a”(ASCII 值 97)。
因此,以下是使用 ASCII 值直接实现简单替换密码解密的 Python 代码:
# function for decryption def substitution_decrypt(encrypted_text, shift): decrypted_text = "" for char in encrypted_text: if char.isalpha(): # Use ASCII values directly to reverse the shift for decryption ascii_val = ord(char) shifted_ascii = ascii_val - shift if char.isupper(): if shifted_ascii < 65: shifted_ascii += 26 elif shifted_ascii > 90: shifted_ascii -= 26 elif char.islower(): if shifted_ascii < 97: shifted_ascii += 26 elif shifted_ascii > 122: shifted_ascii -= 26 decrypted_text += chr(shifted_ascii) else: decrypted_text += char return decrypted_text # our cipher text encrypted_text = "Khoor, pb ghdu froohdjxh!" shift = 3 decrypted_text = substitution_decrypt(encrypted_text, shift) print("Cipher Text: ", encrypted_text) print("Decrypted text:", decrypted_text)
以下是上述示例的输出:
输入/输出
Cipher Text: Khoor, pb ghdu froohdjxh! Decrypted text: Hello, my dear colleague!
使用 Java 实现
在此示例中,我们将使用 Java 实现简单替换密码的解密。因此,我们将使用 Java 的 Hashmap 和 Map 库来实现此功能。decryptMessage() 方法的工作原理与加密方法类似,但在此我们将使用解密映射。它将每个加密的字母映射回字母表中的实际字母。我们将使用此方法解密我们在上一章中生成的密文并获取原始明文。
示例
因此,使用 Java 的 Hashmap 和 Map 库的实现如下:
import java.util.HashMap; import java.util.Map; public class SSC { private static final String alphabet = "abcdefghijklmnopqrstuvwxyz"; private static final String encrypted_alphabet = "bcdefghijklmnopqrstuvwxyza"; private static final Map<Character, Character> encryptionMap = new HashMap<>(); private static final Map<Character, Character> decryptionMap = new HashMap<>(); static { for (int i = 0; i < alphabet.length(); i++) { encryptionMap.put(alphabet.charAt(i), encrypted_alphabet.charAt(i)); decryptionMap.put(encrypted_alphabet.charAt(i), alphabet.charAt(i)); } } public static String decryptMessage(String ciphertext) { StringBuilder plaintext = new StringBuilder(); for (char c : ciphertext.toLowerCase().toCharArray()) { if (decryptionMap.containsKey(c)) { plaintext.append(decryptionMap.get(c)); } else { plaintext.append(c); } } return plaintext.toString(); } public static void main(String[] args) { String et = "ifmmp uvupsjbmtqpjou"; System.out.println("The Encrypted text: " + et); String dt = decryptMessage(et); System.out.println("The Decrypted text: " + dt); } }
以下是上述示例的输出:
输入/输出
The Encrypted text: ifmmp uvupsjbmtqpjou The Decrypted text: hello tutorialspoint
使用 C++ 实现
现在我们将使用 C++ 来实现简单替换密码的解密过程。因此,我们将反转我们在上一章中讨论过的加密过程。因此,在此我们使用 C++ 的“unordered_map”容器。它用于将每个加密的字母映射回字母表中的实际形式。我们将使用此方法解密密文并获取明文消息。
示例
请参阅以下 C++ 编程语言中简单替换密码解密函数的代码:
#include <iostream> #include <unordered_map> #include <string> using namespace std; class SSC { private: const string alphabet = "abcdefghijklmnopqrstuvwxyz"; const string encrypted_alphabet = "bcdefghijklmnopqrstuvwxyza"; unordered_map<char, char> encryptionMap; unordered_map<char, char> decryptionMap; public: SSC() { for (int i = 0; i < alphabet.length(); i++) { encryptionMap[alphabet[i]] = encrypted_alphabet[i]; decryptionMap[encrypted_alphabet[i]] = alphabet[i]; } } string decryptMessage(string ciphertext) { string plaintext = ""; for (char c : ciphertext) { if (decryptionMap.find(tolower(c)) != decryptionMap.end()) { plaintext += decryptionMap[tolower(c)]; } else { plaintext += c; } } return plaintext; } }; int main() { SSC cipher; string et = "ifmmp uifsf ipx bsf zpv!"; cout << "The Encrypted text: " << et << endl; string dt = cipher.decryptMessage(et); cout << "The Decrypted text: " << dt << endl; return 0; }
以下是上述示例的输出:
输入/输出
The Encrypted text: ifmmp uifsf ipx bsf zpv! The Decrypted text: hello there how are you!
总结
本章介绍了在 Python、Java 和 C++ 中解密简单替换密码的不同方法。我们已经看到了使用指定偏移量、字典映射、直接使用 ASCII 值以及通过映射的方法。这些方法解释了使用简单替换密码解密加密文本的不同方法,这些方法提供了使用不同编程语言的灵活性。