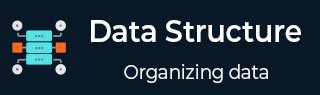
- 数据结构与算法
- DSA - 首页
- DSA - 概述
- DSA - 环境设置
- DSA - 算法基础
- DSA - 渐近分析
- 数据结构
- DSA - 数据结构基础
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表数据结构
- DSA - 双向链表数据结构
- DSA - 循环链表数据结构
- 栈与队列
- DSA - 栈数据结构
- DSA - 表达式解析
- DSA - 队列数据结构
- 搜索算法
- DSA - 搜索算法
- DSA - 线性搜索算法
- DSA - 二分搜索算法
- DSA - 插值搜索
- DSA - 跳跃搜索算法
- DSA - 指数搜索
- DSA - 斐波那契搜索
- DSA - 子列表搜索
- DSA - 哈希表
- 排序算法
- DSA - 排序算法
- DSA - 冒泡排序算法
- DSA - 插入排序算法
- DSA - 选择排序算法
- DSA - 归并排序算法
- DSA - 希尔排序算法
- DSA - 堆排序
- DSA - 桶排序算法
- DSA - 计数排序算法
- DSA - 基数排序算法
- DSA - 快速排序算法
- 图数据结构
- DSA - 图数据结构
- DSA - 深度优先遍历
- DSA - 广度优先遍历
- DSA - 生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树的遍历
- DSA - 二叉搜索树
- DSA - AVL树
- DSA - 红黑树
- DSA - B树
- DSA - B+树
- DSA - 伸展树
- DSA - 字典树 (Trie)
- DSA - 堆数据结构
- 递归
- DSA - 递归算法
- DSA - 使用递归实现汉诺塔
- DSA - 使用递归实现斐波那契数列
- 分治法
- DSA - 分治法
- DSA - 最大最小问题
- DSA - Strassen矩阵乘法
- DSA - Karatsuba算法
- 贪心算法
- DSA - 贪心算法
- DSA - 旅行商问题(贪心法)
- DSA - Prim最小生成树
- DSA - Kruskal最小生成树
- DSA - Dijkstra最短路径算法
- DSA - 地图着色算法
- DSA - 分数背包问题
- DSA - 带截止期限的作业排序
- DSA - 最优合并模式算法
- 动态规划
- DSA - 动态规划
- DSA - 矩阵链乘法
- DSA - Floyd-Warshall算法
- DSA - 0-1背包问题
- DSA - 最长公共子序列算法
- DSA - 旅行商问题(动态规划法)
- 近似算法
- DSA - 近似算法
- DSA - 顶点覆盖算法
- DSA - 集合覆盖问题
- DSA - 旅行商问题(近似算法)
- 随机化算法
- DSA - 随机化算法
- DSA - 随机化快速排序算法
- DSA - Karger最小割算法
- DSA - Fisher-Yates洗牌算法
- DSA有用资源
- DSA - 问答
- DSA - 快速指南
- DSA - 有用资源
- DSA - 讨论
集合覆盖问题
集合覆盖算法为许多现实世界中的资源分配问题提供了解决方案。例如,考虑一家航空公司为每架飞机分配机组人员,以确保他们有足够的人员来满足旅程的要求。他们会考虑航班时间、持续时间、中途停留以及机组人员的可用性,以便将他们分配到航班。这就是集合覆盖算法发挥作用的地方。
给定一个包含一些元素的全集 U,所有这些元素都被分成子集。将这些子集的集合视为 S = {S1, S2, S3, S4... Sn},集合覆盖算法找到最少的子集数量,使得它们覆盖全集中的所有元素。
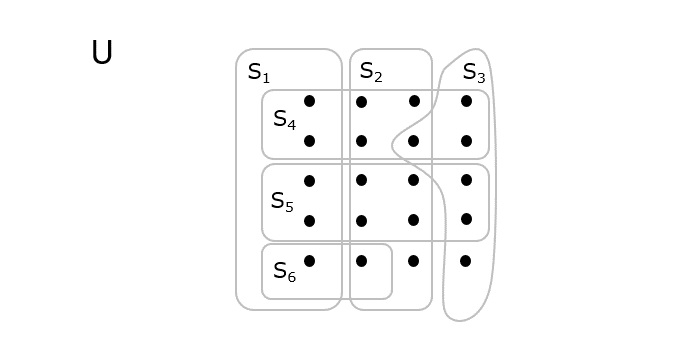
如上图所示,点表示存在于全集 U 中的元素,这些元素被分成不同的集合 S = {S1, S2, S3, S4, S5, S6}。为了覆盖所有元素,需要选择的最小集合数将是最优输出 = {S1, S2, S3}。
集合覆盖算法
集合覆盖算法将集合的集合作为输入,并返回包含所有全集元素所需的最小集合数。
集合覆盖问题是一个 NP-Hard 问题,并且是一个 2-逼近贪心算法。
算法
步骤 1 - 初始化 Output = {},其中 Output 表示输出元素集。
步骤 2 - 当 Output 集不包含全集中的所有元素时,执行以下操作:
使用公式 $\frac{Cost\left ( S_{i} \right )}{S_{i}-Output}$ 查找全集中的每个子集的成本效益。
找到每次迭代中成本效益最低的子集。将子集添加到 Output 集。
步骤 3 - 重复步骤 2,直到宇宙中没有剩余元素。达到的输出是最终的 Output 集。
伪代码
APPROX-GREEDY-SET_COVER(X, S) U = X OUTPUT = ф while U ≠ ф select Si Є S which has maximum |Si∩U| U = U – S OUTPUT = OUTPUT∪ {Si} return OUTPUT
分析
假设元素总数等于集合总数(|X| = |S|),则代码运行时间为 O(|X|3)
示例
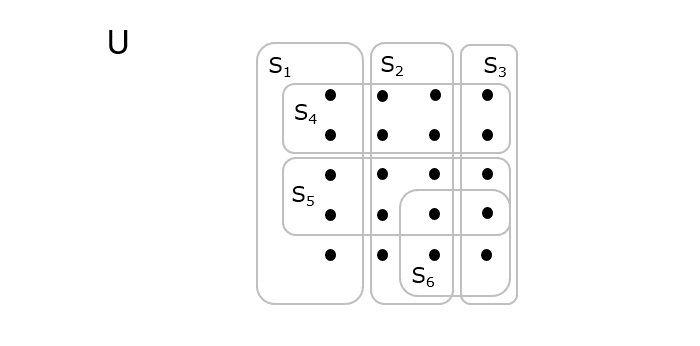
让我们来看一个更详细地描述集合覆盖问题近似算法的例子
S1 = {1, 2, 3, 4} cost(S1) = 5 S2 = {2, 4, 5, 8, 10} cost(S2) = 10 S3 = {1, 3, 5, 7, 9, 11, 13} cost(S3) = 20 S4 = {4, 8, 12, 16, 20} cost(S4) = 12 S5 = {5, 6, 7, 8, 9} cost(S5) = 15
步骤 1
输出集 Output = ф
找到输出集中没有元素时每个集合的成本效益,
S1 = cost(S1) / (S1 – Output) = 5 / (4 – 0) S2 = cost(S2) / (S2 – Output) = 10 / (5 – 0) S3 = cost(S3) / (S3 – Output) = 20 / (7 – 0) S4 = cost(S4) / (S4 – Output) = 12 / (5 – 0) S5 = cost(S5) / (S5 – Output) = 15 / (5 – 0)
本次迭代中,S1 的成本效益最低,因此,添加到输出集的子集 Output = {S1},其元素为 {1, 2, 3, 4}。
步骤 2
找到输出集中新元素的每个集合的成本效益,
S2 = cost(S2) / (S2 – Output) = 10 / (5 – 4) S3 = cost(S3) / (S3 – Output) = 20 / (7 – 4) S4 = cost(S4) / (S4 – Output) = 12 / (5 – 4) S5 = cost(S5) / (S5 – Output) = 15 / (5 – 4)
本次迭代中,S3 的成本效益最低,因此,添加到输出集的子集 Output = {S1, S3},其元素为 {1, 2, 3, 4, 5, 7, 9, 11, 13}。
步骤 3
找到输出集中新元素的每个集合的成本效益,
S2 = cost(S2) / (S2 – Output) = 10 / |(5 – 9)| S4 = cost(S4) / (S4 – Output) = 12 / |(5 – 9)| S5 = cost(S5) / (S5 – Output) = 15 / |(5 – 9)|
本次迭代中,S2 的成本效益最低,因此,添加到输出集的子集 Output = {S1, S3, S2},其元素为 {1, 2, 3, 4, 5, 7, 8, 9, 10, 11, 13}。
步骤 4
找到输出集中新元素的每个集合的成本效益,
S4 = cost(S4) / (S4 – Output) = 12 / |(5 – 11)| S5 = cost(S5) / (S5 – Output) = 15 / |(5 – 11)|
本次迭代中,S4 的成本效益最低,因此,添加到输出集的子集 Output = {S1, S3, S2, S4},其元素为 {1, 2, 3, 4, 5, 7, 8, 9, 10, 11, 12, 13, 16, 20}。
步骤 5
找到输出集中新元素的每个集合的成本效益,
S5 = cost(S5) / (S5 – Output) = 15 / |(5 – 14)|
本次迭代中,S5 的成本效益最低,因此,添加到输出集的子集 Output = {S1, S3, S2, S4, S5},其元素为 {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 16, 20}。
最终覆盖有限全集中的所有元素的输出为 Output = {S1, S3, S2, S4, S5}。
实现
以下是上述方法在各种编程语言中的实现:
#include <stdio.h> #define MAX_SETS 100 #define MAX_ELEMENTS 1000 int setCover(int X[], int S[][MAX_ELEMENTS], int numSets, int numElements, int output[]) { int U[MAX_ELEMENTS]; for (int i = 0; i < numElements; i++) { U[i] = X[i]; } int selectedSets[MAX_SETS]; for (int i = 0; i < MAX_SETS; i++) { selectedSets[i] = 0; // Initialize all to 0 (not selected) } int outputIdx = 0; while (outputIdx < numSets) { // Ensure we don't exceed the maximum number of sets int maxIntersectionSize = 0; int selectedSetIdx = -1; // Find the set Si with the maximum intersection with U for (int i = 0; i < numSets; i++) { if (selectedSets[i] == 0) { // Check if the set is not already selected int intersectionSize = 0; for (int j = 0; j < numElements; j++) { if (U[j] && S[i][j]) { intersectionSize++; } } if (intersectionSize > maxIntersectionSize) { maxIntersectionSize = intersectionSize; selectedSetIdx = i; } } } // If no set found, break from the loop if (selectedSetIdx == -1) { break; } // Mark the selected set as "selected" in the array selectedSets[selectedSetIdx] = 1; // Remove the elements covered by the selected set from U for (int j = 0; j < numElements; j++) { U[j] = U[j] - S[selectedSetIdx][j]; } // Add the selected set to the output output[outputIdx++] = selectedSetIdx; } return outputIdx; } int main() { int X[MAX_ELEMENTS] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int S[MAX_SETS][MAX_ELEMENTS] = { {1, 1, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 1, 1, 1, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 1, 1, 1, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 1, 1, 1, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 1, 1, 1} }; int numSets = 5; int numElements = 10; int output[MAX_SETS]; int numSelectedSets = setCover(X, S, numSets, numElements, output); printf("Selected Sets: "); for (int i = 0; i < numSelectedSets; i++) { printf("%d ", output[i]); } printf("\n"); return 0; }
输出
Selected Sets: 1 2 3 4 0
#include <iostream> #include <vector> using namespace std; #define MAX_SETS 100 #define MAX_ELEMENTS 1000 // Function to find the set cover using the Approximate Greedy Set Cover algorithm int setCover(int X[], int S[][MAX_ELEMENTS], int numSets, int numElements, int output[]) { int U[MAX_ELEMENTS]; for (int i = 0; i < numElements; i++) { U[i] = X[i]; } int selectedSets[MAX_SETS]; for (int i = 0; i < MAX_SETS; i++) { selectedSets[i] = 0; // Initialize all to 0 (not selected) } int outputIdx = 0; while (outputIdx < numSets) { // Ensure we don't exceed the maximum number of sets int maxIntersectionSize = 0; int selectedSetIdx = -1; // Find the set Si with maximum intersection with U for (int i = 0; i < numSets; i++) { if (selectedSets[i] == 0) { // Check if the set is not already selected int intersectionSize = 0; for (int j = 0; j < numElements; j++) { if (U[j] && S[i][j]) { intersectionSize++; } } if (intersectionSize > maxIntersectionSize) { maxIntersectionSize = intersectionSize; selectedSetIdx = i; } } } // If no set found, break from the loop if (selectedSetIdx == -1) { break; } // Mark the selected set as "selected" in the array selectedSets[selectedSetIdx] = 1; // Remove the elements covered by the selected set from U for (int j = 0; j < numElements; j++) { U[j] = U[j] - S[selectedSetIdx][j]; } // Add the selected set to the output output[outputIdx++] = selectedSetIdx; } return outputIdx; } int main() { int X[MAX_ELEMENTS] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int S[MAX_SETS][MAX_ELEMENTS] = { {1, 1, 0, 0, 0, 0, 0, 0, 0, 0}, {0, 1, 1, 1, 0, 0, 0, 0, 0, 0}, {0, 0, 0, 1, 1, 1, 0, 0, 0, 0}, {0, 0, 0, 0, 0, 1, 1, 1, 0, 0}, {0, 0, 0, 0, 0, 0, 0, 1, 1, 1} }; int numSets = 5; int numElements = 10; int output[MAX_SETS]; int numSelectedSets = setCover(X, S, numSets, numElements, output); cout << "Selected Sets: "; for (int i = 0; i < numSelectedSets; i++) { cout << output[i] << " "; } cout << endl; return 0; }
输出
Selected Sets: 1 2 3 4 0
import java.util.*; public class SetCover { public static List<Integer> setCover(int[] X, int[][] S) { Set<Integer> U = new HashSet<>(); for (int x : X) { U.add(x); } List<Integer> output = new ArrayList<>(); while (!U.isEmpty()) { int maxIntersectionSize = 0; int selectedSetIdx = -1; for (int i = 0; i < S.length; i++) { int intersectionSize = 0; for (int j = 0; j < S[i].length; j++) { if (U.contains(S[i][j])) { intersectionSize++; } } if (intersectionSize > maxIntersectionSize) { maxIntersectionSize = intersectionSize; selectedSetIdx = i; } } if (selectedSetIdx == -1) { break; } for (int j = 0; j < S[selectedSetIdx].length; j++) { U.remove(S[selectedSetIdx][j]); } output.add(selectedSetIdx); } return output; } public static void main(String[] args) { int[] X = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int[][] S = { {1, 2}, {2, 3, 4}, {4, 5, 6}, {6, 7, 8}, {8, 9, 10} }; List<Integer> selectedSets = setCover(X, S); System.out.print("Selected Sets: "); for (int idx : selectedSets) { System.out.print(idx + " "); } System.out.println(); } }
输出
Selected Sets: 1 3 4 0 2
def set_cover(X, S): U = set(X) output = [] while U: max_intersection_size = 0 selected_set_idx = -1 for i, s in enumerate(S): intersection_size = len(U.intersection(s)) if intersection_size > max_intersection_size: max_intersection_size = intersection_size selected_set_idx = i if selected_set_idx == -1: break U = U - set(S[selected_set_idx]) output.append(selected_set_idx) return output if __name__ == "__main__": X = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] S = [ {1, 2}, {2, 3, 4}, {4, 5, 6}, {6, 7, 8}, {8, 9, 10} ] selected_sets = set_cover(X, S) print("Selected Sets:", selected_sets)
输出
Selected Sets: 1 3 4 0 2