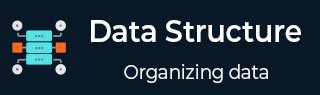
- 数据结构与算法
- DSA - 首页
- DSA - 概述
- DSA - 环境设置
- DSA - 算法基础
- DSA - 渐近分析
- 数据结构
- DSA - 数据结构基础
- DSA - 数据结构和类型
- DSA - 数组数据结构
- 链表
- DSA - 链表数据结构
- DSA - 双向链表数据结构
- DSA - 循环链表数据结构
- 栈与队列
- DSA - 栈数据结构
- DSA - 表达式解析
- DSA - 队列数据结构
- 搜索算法
- DSA - 搜索算法
- DSA - 线性搜索算法
- DSA - 二分搜索算法
- DSA - 插值搜索
- DSA - 跳跃搜索算法
- DSA - 指数搜索
- DSA - 斐波那契搜索
- DSA - 子列表搜索
- DSA - 哈希表
- 排序算法
- DSA - 排序算法
- DSA - 冒泡排序算法
- DSA - 插入排序算法
- DSA - 选择排序算法
- DSA - 归并排序算法
- DSA - 希尔排序算法
- DSA - 堆排序
- DSA - 桶排序算法
- DSA - 计数排序算法
- DSA - 基数排序算法
- DSA - 快速排序算法
- 图数据结构
- DSA - 图数据结构
- DSA - 深度优先遍历
- DSA - 广度优先遍历
- DSA - 生成树
- 树数据结构
- DSA - 树数据结构
- DSA - 树遍历
- DSA - 二叉搜索树
- DSA - AVL树
- DSA - 红黑树
- DSA - B树
- DSA - B+树
- DSA - 伸展树
- DSA - 字典树 (Trie)
- DSA - 堆数据结构
- 递归
- DSA - 递归算法
- DSA - 使用递归实现汉诺塔
- DSA - 使用递归实现斐波那契数列
- 分治法
- DSA - 分治法
- DSA - 最大最小问题
- DSA - Strassen矩阵乘法
- DSA - Karatsuba算法
- 贪心算法
- DSA - 贪心算法
- DSA - 旅行商问题(贪心法)
- DSA - Prim最小生成树
- DSA - Kruskal最小生成树
- DSA - Dijkstra最短路径算法
- DSA - 地图着色算法
- DSA - 分数背包问题
- DSA - 带截止日期的作业排序
- DSA - 最优合并模式算法
- 动态规划
- DSA - 动态规划
- DSA - 矩阵链乘法
- DSA - Floyd-Warshall算法
- DSA - 0-1背包问题
- DSA - 最长公共子序列算法
- DSA - 旅行商问题(动态规划法)
- 近似算法
- DSA - 近似算法
- DSA - 顶点覆盖算法
- DSA - 集合覆盖问题
- DSA - 旅行商问题(近似算法)
- 随机化算法
- DSA - 随机化算法
- DSA - 随机化快速排序算法
- DSA - Karger最小割算法
- DSA - Fisher-Yates洗牌算法
- DSA有用资源
- DSA - 问答
- DSA - 快速指南
- DSA - 有用资源
- DSA - 讨论
带截止日期的作业排序
作业调度算法用于在一个处理器上调度作业以最大化利润。
作业调度算法的贪心方法指出:“给定n个作业,每个作业都有起始时间和结束时间,需要以某种方式安排这些作业,以便在最大截止日期内获得最大利润”。
作业调度算法
带有截止日期和利润的作业集作为输入提供给作业调度算法,并获得作为最终输出的具有最大利润的作业调度子集。
算法
Step1 − Find the maximum deadline value from the input set of jobs. Step2 − Once, the deadline is decided, arrange the jobs in descending order of their profits. Step3 − Selects the jobs with highest profits, their time periods not exceeding the maximum deadline. Step4 − The selected set of jobs are the output.
示例
考虑以下任务及其截止日期和利润。安排这些任务,以便在执行后产生最大利润:
序号 | 1 | 2 | 3 | 4 | 5 |
---|---|---|---|---|---|
作业 | J1 | J2 | J3 | J4 | J5 |
截止日期 | 2 | 2 | 1 | 3 | 4 |
利润 | 20 | 60 | 40 | 100 | 80 |
步骤1
从给定的截止日期中找到最大截止日期值 dm。
dm = 4.
步骤2
按其利润的降序排列作业。
序号 | 1 | 2 | 3 | 4 | 5 |
---|---|---|---|---|---|
作业 | J4 | J5 | J2 | J3 | J1 |
截止日期 | 3 | 4 | 2 | 1 | 2 |
利润 | 100 | 80 | 60 | 40 | 20 |
最大截止日期dm为4。因此,所有任务必须在4之前结束。
选择利润最高的作业J4。它占用最大截止日期的3个部分。
因此,下一个作业的时间段必须为1。
总利润 = 100。
步骤3
下一个利润最高的作业是J5。但是J5花费的时间为4,超过截止日期3。因此,它不能添加到输出集合中。
步骤4
下一个利润最高的作业是J2。J5花费的时间为2,也超过截止日期1。因此,它不能添加到输出集合中。
步骤5
下一个利润更高的作业是J3。J3花费的时间为1,不超过给定的截止日期。因此,J3被添加到输出集合中。
Total Profit: 100 + 40 = 140
步骤6
由于满足最大截止日期,算法结束。在截止日期内安排的作业输出集为{J4,J3},最大利润为140。
示例
以下是使用贪心方法的作业排序算法的最终实现:
#include <stdbool.h> #include <stdio.h> #include <stdlib.h> // A structure to represent a Jobs typedef struct Jobs { char id; // Jobs Id int dead; // Deadline of Jobs int profit; // Profit if Jobs is over before or on deadline } Jobs; // This function is used for sorting all Jobss according to // profit int compare(const void* a, const void* b){ Jobs* temp1 = (Jobs*)a; Jobs* temp2 = (Jobs*)b; return (temp2->profit - temp1->profit); } // Find minimum between two numbers. int min(int num1, int num2){ return (num1 > num2) ? num2 : num1; } int main(){ Jobs arr[] = { { 'a', 2, 100 }, { 'b', 2, 20 }, { 'c', 1, 40 }, { 'd', 3, 35 }, { 'e', 1, 25 } }; int n = sizeof(arr) / sizeof(arr[0]); printf("Following is maximum profit sequence of Jobs: \n"); qsort(arr, n, sizeof(Jobs), compare); int result[n]; // To store result sequence of Jobs bool slot[n]; // To keep track of free time slots // Initialize all slots to be free for (int i = 0; i < n; i++) slot[i] = false; // Iterate through all given Jobs for (int i = 0; i < n; i++) { // Find a free slot for this Job for (int j = min(n, arr[i].dead) - 1; j >= 0; j--) { // Free slot found if (slot[j] == false) { result[j] = i; slot[j] = true; break; } } } // Print the result for (int i = 0; i < n; i++) if (slot[i]) printf("%c ", arr[result[i]].id); return 0; }
输出
Following is maximum profit sequence of Jobs: c a d
#include<iostream> #include<algorithm> using namespace std; struct Job { char id; int deadLine; int profit; }; bool comp(Job j1, Job j2){ return (j1.profit > j2.profit); //compare jobs based on profit } int min(int a, int b){ return (a<b)?a:b; } int main(){ Job jobs[] = { { 'a', 2, 100 }, { 'b', 2, 20 }, { 'c', 1, 40 }, { 'd', 3, 35 }, { 'e', 1, 25 } }; int n = 5; cout << "Following is maximum profit sequence of Jobs: "<<"\n"; sort(jobs, jobs+n, comp); //sort jobs on profit int jobSeq[n]; // To store result (Sequence of jobs) bool slot[n]; // To keep track of free time slots for (int i=0; i<n; i++) slot[i] = false; //initially all slots are free for (int i=0; i<n; i++) { //for all given jobs for (int j=min(n, jobs[i].deadLine)-1; j>=0; j--) { //search from last free slot if (slot[j]==false) { jobSeq[j] = i; // Add this job to job sequence slot[j] = true; // mark this slot as occupied break; } } } for (int i=0; i<n; i++) if (slot[i]) cout << jobs[jobSeq[i]].id << " "; //display the sequence }
输出
Following is maximum profit sequence of Jobs: c a d
import java.util.*; public class Job { // Each job has a unique-id,profit and deadline char id; int deadline, profit; // Constructors public Job() {} public Job(char id, int deadline, int profit) { this.id = id; this.deadline = deadline; this.profit = profit; } // Function to schedule the jobs take 2 arguments // arraylist and no of jobs to schedule void printJobScheduling(ArrayList<Job> arr, int t) { // Length of array int n = arr.size(); // Sort all jobs according to decreasing order of // profit Collections.sort(arr,(a, b) -> b.profit - a.profit); // To keep track of free time slots boolean result[] = new boolean[t]; // To store result (Sequence of jobs) char job[] = new char[t]; // Iterate through all given jobs for (int i = 0; i < n; i++) { // Find a free slot for this job (Note that we // start from the last possible slot) for (int j = Math.min(t - 1, arr.get(i).deadline - 1); j >= 0; j--) { // Free slot found if (result[j] == false) { result[j] = true; job[j] = arr.get(i).id; break; } } } // Print the sequence for (char jb : job) System.out.print(jb + " "); System.out.println(); } // Driver code public static void main(String args[]) { ArrayList<Job> arr = new ArrayList<Job>(); arr.add(new Job('a', 2, 100)); arr.add(new Job('b', 2, 20)); arr.add(new Job('c', 1, 40)); arr.add(new Job('d', 3, 35)); arr.add(new Job('e', 1, 25)); // Function call System.out.println("Following is maximum profit sequence of Jobs: "); Job job = new Job(); // Calling function job.printJobScheduling(arr, 3); } }
输出
Following is maximum profit sequence of Jobs: c a d
arr = [ ['a', 2, 100], ['b', 2, 20], ['c', 1, 40], ['d', 3, 35], ['e', 1, 25] ] print("Following is maximum profit sequence of Jobs: ") # length of array n = len(arr) t = 3 # Sort all jobs according to # decreasing order of profit for i in range(n): for j in range(n - 1 - i): if arr[j][2] < arr[j + 1][2]: arr[j], arr[j + 1] = arr[j + 1], arr[j] # To keep track of free time slots result = [False] * t # To store result (Sequence of jobs) job = ['-1'] * t # Iterate through all given jobs for i in range(len(arr)): # Find a free slot for this job # (Note that we start from the # last possible slot) for j in range(min(t - 1, arr[i][1] - 1), -1, -1): # Free slot found if result[j] is False: result[j] = True job[j] = arr[i][0] break # print the sequence print(job)
输出
Following is maximum profit sequence of Jobs: ['c', 'a', 'd']
广告